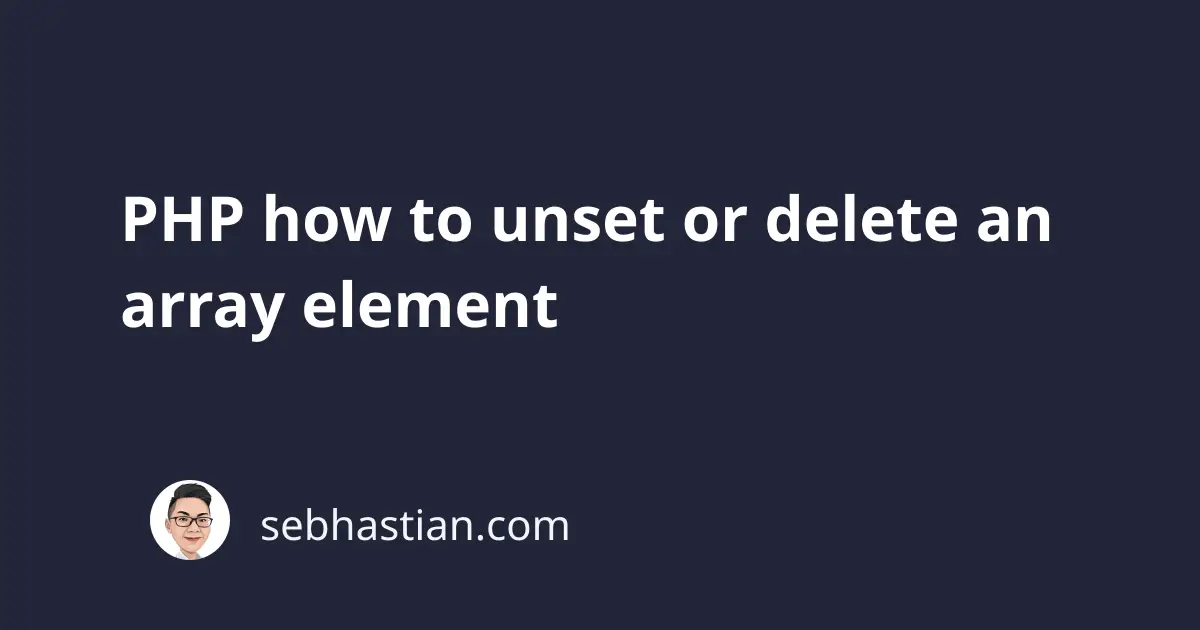
To remove an array element in PHP, you can call the unset()
function.
The unset()
function allows you to remove an array element at a specific key as follows:
<?php
$arr = ["Nathan", "Jack", "John"];
// 👇 remove array element at key 1
unset($arr[1]);
print_r($arr);
The print_r
output will be as follows:
Array
(
[0] => Nathan
[2] => John
)
Note how the array keys are not reindexed after you remove the element.
To reset the order of the keys, you need to call the array_values()
function as shown below:
<?php
$arr = ["Nathan", "Jack", "John"];
// 👇 remove array element at key 1
unset($arr[1]);
// 👇 reindex the array keys
$arr = array_values($arr);
print_r($arr);
Calling the array_values()
function will return an array containing the values of the array with numbered keys.
The print_r
output will show a reindexed array:
Array
(
[0] => Nathan
[1] => John
)
The reason the array keys are not reindexed after unset()
is that so you can use the function to remove an element from an associative array.
With an associative array, the string keys will not be replaced with number keys:
<?php
$arr = [
"user_1" => "Nathan",
"user_2" => "Jack",
"user_3" => "John",
];
// 👇 remove user_2
unset($arr['user_2']);
print_r($arr);
The code above will give the following output:
Array
(
[user_1] => Nathan
[user_3] => John
)
And that’s how you remove an array element using PHP unset()
function.
You’ve also learned how to reindex a non-associative array using the array_values()
function after unsetting an element. Nice work!