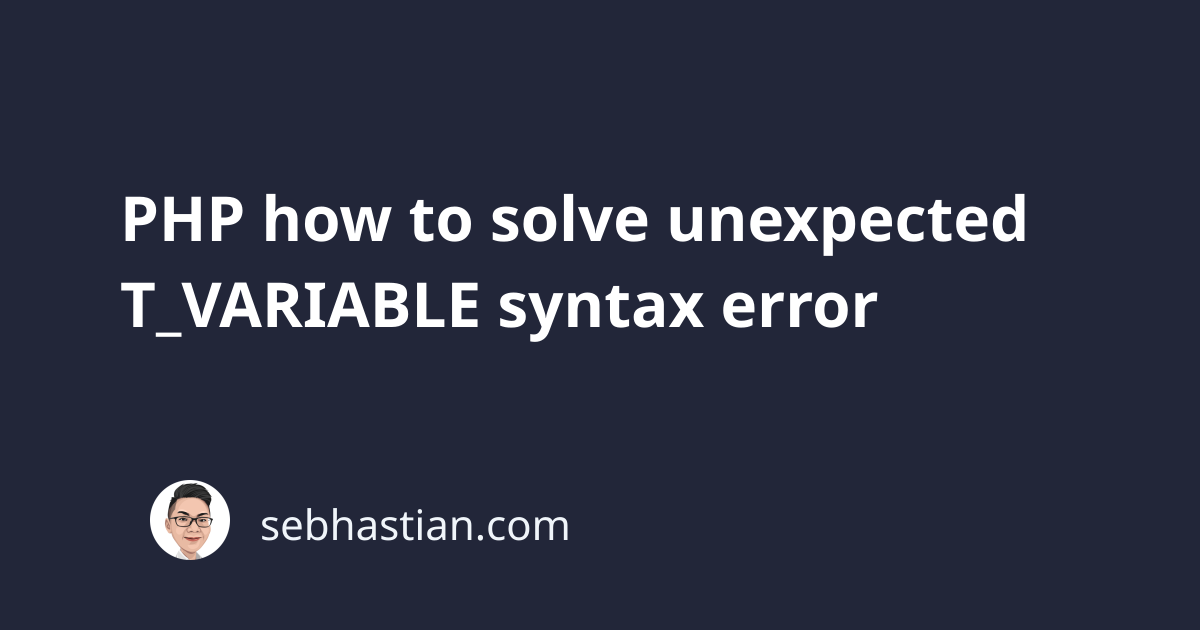
The syntax error unexpected T_VARIABLE
occurs when PHP unexpectedly finds a variable when it expected something else.
An example of the error can be produced by running the code below:
<?php
$a = "Nathan"
$b = "Jane";
$c = "Harry";
The above code will give the following error:
Parse error: syntax error, unexpected T_VARIABLE
in /DEV/index.php on line 3
When we declare the variable $a
in the example, PHP expected a semicolon to close the assignment.
But the semicolon was missing, and PHP found the $b
variable declaration instead.
A semicolon is required to end a variable declaration. Without a semicolon, PHP will continue to execute the code as if they are in the same line:
This is how PHP process the code above:
<?php
$a = "Nathan" $b = "Jane";
$c = "Harry";
The T_VARIABLE
in PHP means TOKEN_VARIABLE
, so this error means PHP didn’t expect a variable on the error line.
To fix the error, add a semicolon to the $a
declaration:
<?php
$a = "Nathan";
$b = "Jane";
$c = "Harry";
The cause of T_VARIABLE
may be different in your case, but most often you need to check the line above the one mentioned in the error message.
So when the error says line 3, you check on line 2.
Let’s see another example below:
<?php
require ("db.php")
$rows = mysql_query("SELECT * FROM users");
The above code will produce the error:
Parse error: syntax error, unexpected T_VARIABLE
in /DEV/index.php on line 4
The error happens because there is no semicolon after the require()
statement.
Add the semicolon to fix the error:
<?php
require ("db.php");
$rows = mysql_query("SELECT * FROM users");
The T_VARIABLE
syntax error happens when you forget to add a semicolon at the end of the line somewhere before the error.
You need to check on the line before the error happens. If the error message says line 4, then check on line 3.
If line 3 is empty as in the example above, check on line 2.
And that’s how you solve the unexpected T_VARIABLE
syntax error in PHP. Nice! 😉