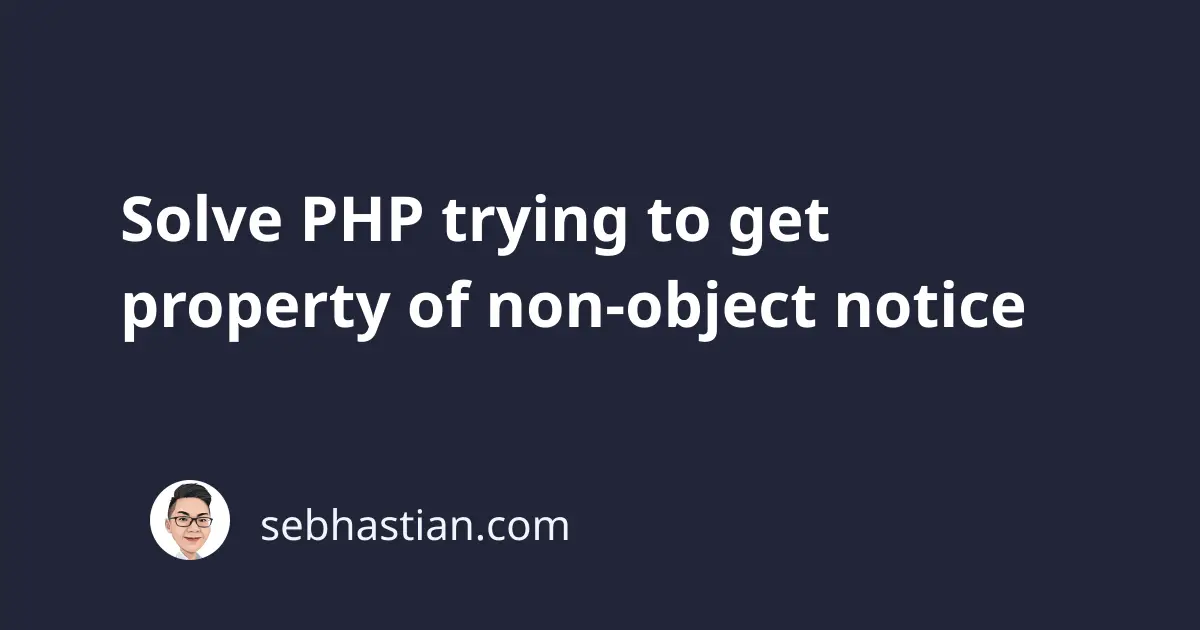
The PHP Notice: Trying to get property of non-object appears when your code tries to access a variable that’s not an object
type.
When accessing an object property, you need to use the property accessor syntax ->
as shown below:
// 👇 access the name property of user
$user->name;
But if the $user
variable is of another type, then PHP will show the notice:
<?php
$user = [
"name" => "Nathan",
];
// 👇 Notice: Trying to get property 'name' of non-object
print $user->name;
?>
The same happens when your $user
variable is of another type like a string
:
<?php
$user = "name: Nathan";
// 👇 Notice: Trying to get property 'name' of non-object
print $user->name;
?>
This PHP Notice can appear when you use vanilla PHP, WordPress, or Laravel to develop your application.
PHP doesn’t stop the script execution because of this notice, but the print
command will output nothing.
So if you have a code like this:
<?php
$user = [
"name" => "Nathan",
];
print "<h1>PHP output:</h1>";
print "<p>".$user->name."</p>";
print "<p>End</p>";
?>
The output will be:
The third print
line still gets printed even when the second fails.
To solve this issue, you first need to check the type of that non-object
variable.
Then, you need to adjust the variable accessor according to the type.
You can check a variable type using the var_dump()
function:
<?php
$user = [
"name" => "Nathan",
];
var_dump($user);
The output of var_dump()
will show the type of the $user
variable:
array(1) { ["name"]=> string(6) "Nathan" }
👆
variable type
If the variable is an object, then var_dump()
will show:
object(stdClass)#1 (1) { ["name"]=> string(6) "Nathan" }
In both outputs above, PHP uses the curly braces {}
before the elements and properties of array and object.
This is why people often mistake an array for an object. You need to pay attention to the left-most output instead of the content as shown below:
When the variable is an array
, you use the array element accessor []
instead of ->
like this:
<?php
$user = [
"name" => "Nathan",
];
print $user["name"]; // Nathan
?>
If it’s a string
, then access the variable using its name directly:
<?php
$user = "name: Nathan",
print $user;
To conclude, the PHP Notice: Trying to get property of non-object appears when you try to access a non-object variable like an object (using ->
to get the property value)
To solve this issue, you need to check the type of the variable and adjust the accessor accordingly.
Most of the time, you are mistaking an array for an object, so changing the accessor from ->
to []
should solve the issue:
<?php
$user = [
"name" => "Nathan",
];
// 👇 Change this
print $user->name;
// 👇 to this
print $user["name"];
?>
Don’t forget to add quotations around the array element name.
And that’s how you solve the PHP Notice: Trying to get property of non-object message. Good work! 👍