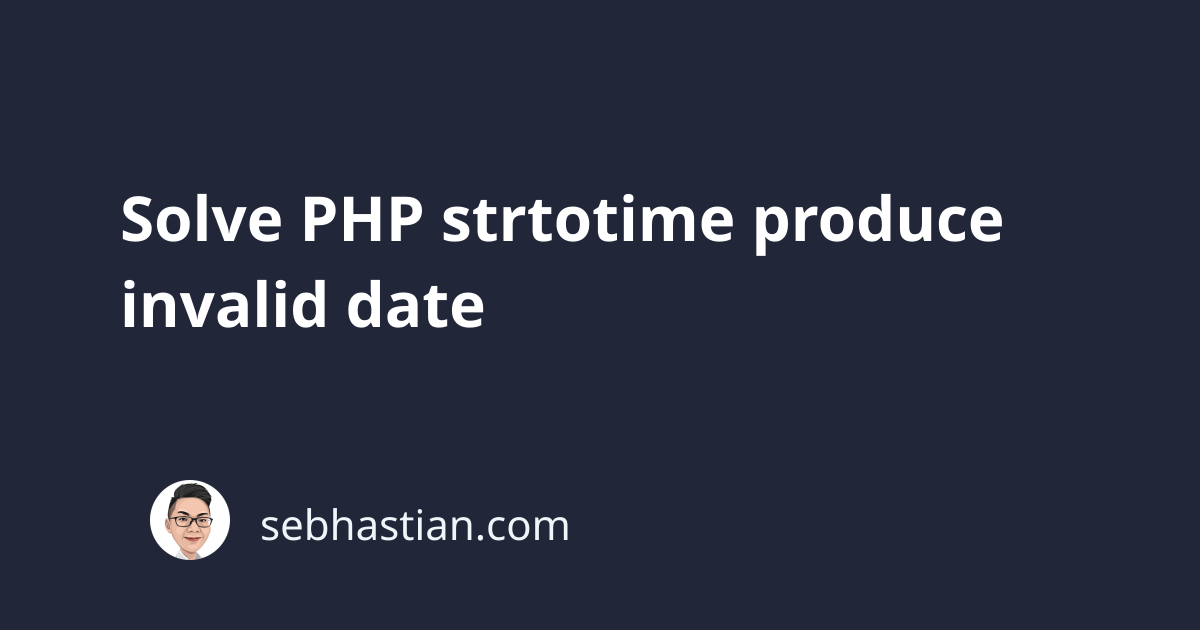
The strtotime()
function is a magical function that allows you to parse a string
of English datetime description into a Unix timestamp.
This function is designed to let PHP interpret what timestamp value you want from a string
, so it has some faults where it can produce an invalid date.
For example, when you put 31-Feb
in the string, strtotime()
pushed the date forward to 3-Mar
as shown below:
<?php
// π this produce invalid date
$time = "10:24 AM 31-Feb 2022";
$my_time = strtotime($time);
// π 10:24 AM 3-Mar 2022
print date("H:i A j-M Y", $my_time);
But strtotime()
will return false
when you put a date greater than 31
as follows:
// π put 32 as date
$time = "10:24 AM 32-Feb 2022";
$my_time = strtotime($time);
// π bool(false)
var_dump ($my_time);
Most often, the behavior that you want from strtotime()
is to return false
when you put an invalid date such as 30-Feb
or 31-Feb
.
Since strtotime()
doesnβt have a built-in date validation, you need to do it yourself.
To validate strtotime()
date, you need to transform the Unix timestamp back to a date string and see if it matches the original date.
You can use the date()
function to format the Unix timestamp as a date string.
Consider the example below:
// π use strtotime()
$time = "10:24 AM 31-Feb 2022";
$my_time = strtotime($time);
// π put strtotime back as date string
$my_time = date("H:i A j-M Y", $my_time);
// π compare original date and strtotime date
if ($time === $my_time) {
print "Date is VALID";
} else {
print "Date is INVALID";
}
Put the date format of your original date string as the first argument of the date()
function.
By comparing the date strings, you can check if the strtotime()
function returns a valid date.
This also works for leap year February dates as shown below:
// π check for 29-Feb 2020
$time = "29-Feb 2020";
$my_time = strtotime($time);
$my_time = date("j-M Y", $my_time);
if ($time === $my_time) {
print "$time is VALID";
} else {
print "$time is INVALID";
}
print "\n";
// π Do another round for 29-Feb 2021
$time = "29-Feb 2021";
$my_time = strtotime($time);
$my_time = date("j-M Y", $my_time);
if ($time === $my_time) {
print "$time is VALID";
} else {
print "$time is INVALID";
}
The output will be:
29-Feb 2020 is VALID
29-Feb 2021 is INVALID
Now youβve learned how to handle the PHP strtotime()
function producing an invalid date. Very nice! π