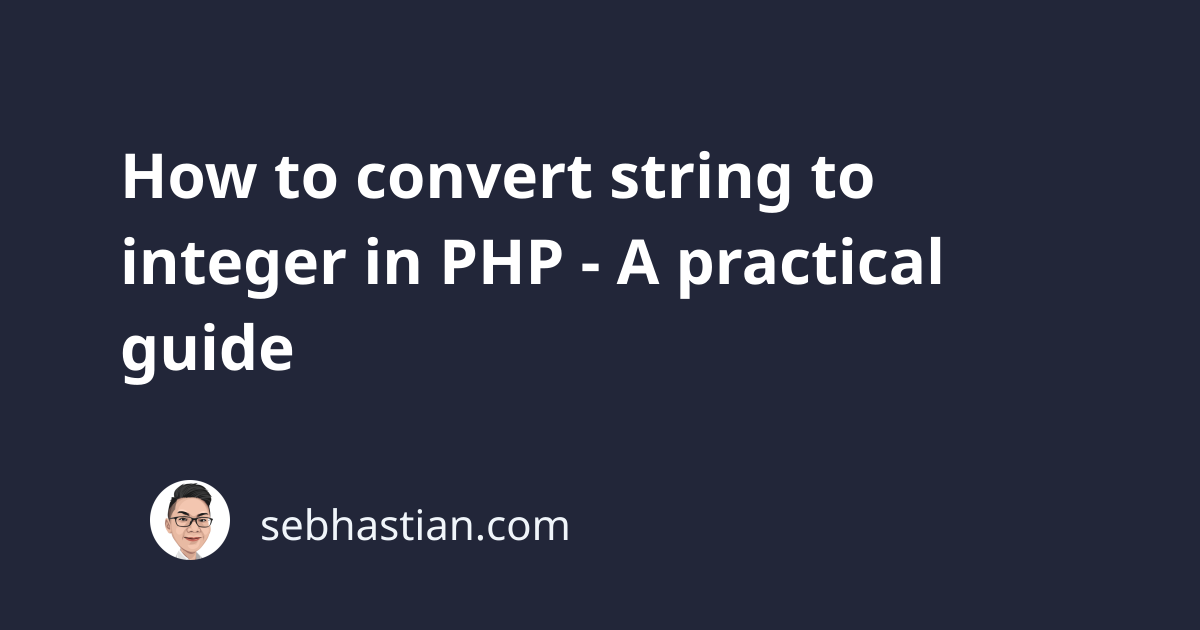
PHP offers several ways to convert a string to an integer. In this article, we’ll take a look at some of the methods you can use to accomplish this task.
First, let’s define what we mean by a string and an integer. In PHP, a string is a sequence of characters, while an integer is a whole number.
For example, the string "hello"
is made up of the characters 'h'
, 'e'
, 'l'
, 'l'
, and 'o'
, while the integer 123
is a whole number.
Next, let’s see how to convert a string to an integer in PHP.
Use the intval() function
One of the simplest ways to convert a string to an integer is to use the intval()
function. This function takes a string as an argument and returns its integer equivalent.
Consider the two examples below:
// example 1
$str = "123hello";
$int = intval($str);
echo gettype($int) . "\n"; // integer
echo $int . "\n"; // 123
// example 2
$str = "hello123";
$int = intval($str);
echo gettype($int) . "\n"; // integer
echo $int . "\n"; // 0
The examples show how the intval()
function tries to convert a string that has letters and numbers.
In the first example, the variable $str
contains the string "123hello"
. When you pass it to the intval()
function, it returns the integer 123
.
The second example shows than when the letters are put in front of the numbers, the function will return 0
because it fails to get the numbers portion of the string.
Use (int) type cast
Another way to convert a string to an integer is to use type casting syntax.
You can use the (int)
or (integer)
operator to convert a value to an integer. Here’s an example:
$str = "3.4";
$int = (int) $str;
echo gettype($int) . "\n"; // integer
echo $int . "\n"; // 3
The above example shows that when you type cast a floating number string into an int
, the floating number portion is removed and a whole number is returned.
If you want to keep the floating number, then you need to use the (float)
type cast syntax instead.
Use the settype() function
Finally, you can use the settype()
function to convert a variable from one data type to another.
This function takes two arguments:
- the variable you want to convert
- The data type you want to convert as a
string
.
See the example below:
$str = "20 weeks";
settype($str, "integer");
echo $str; // 20
Please note that the settype()
function modifies the original value instead of returning a new value. This function may not fit when you need the original value to be available.
To conclude, there are several ways to convert a string to an integer in PHP.
You can use the intval()
function, the (int)
type casting syntax, or you can use the settype()
function.
You can choose the best method according to your requirements.