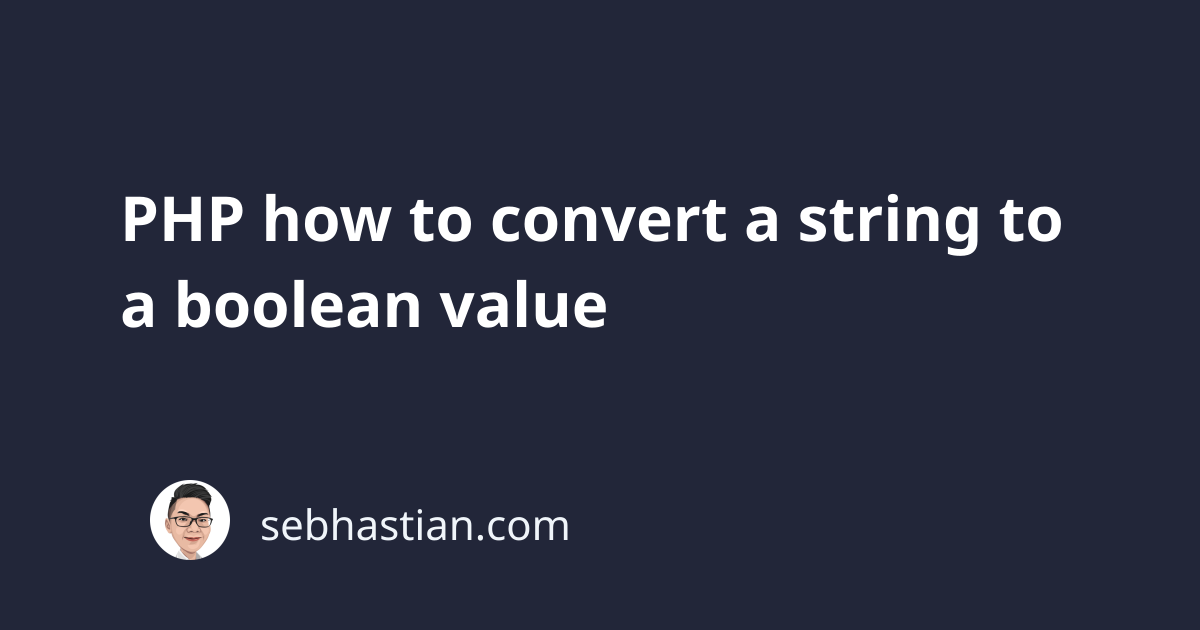
To convert string value to boolean value in PHP, you need to use the filter_var()
function.
The filter_var()
function can be used to validate whether a string is true
or false
depending on its value:
filter_var(
mixed $value,
int $filter = FILTER_DEFAULT,
array|int $options = 0
): mixed
To convert a string to a boolean, you need to pass two parameters to the function:
- The string as
$value
FILTER_VALIDATE_BOOLEAN
as the$filter
argument
The FILTER_VALIDATE_BOOLEAN
flag will return true
for “1”, “true”, “on”, and “yes” string values. Otherwise, it returns false
.
Here are some examples of converting strings to boolean values:
<?php
var_dump(filter_var("true", FILTER_VALIDATE_BOOLEAN));
var_dump(filter_var("false", FILTER_VALIDATE_BOOLEAN));
var_dump(filter_var("yes", FILTER_VALIDATE_BOOLEAN));
var_dump(filter_var("no", FILTER_VALIDATE_BOOLEAN));
The output of the code above will be:
bool(true)
bool(false)
bool(true)
bool(false)
As you can see, the filter_var()
function returns boolean when you use the FILTER_VALIDATE_BOOLEAN
flag.
And that’s how you convert a string to a boolean using PHP.