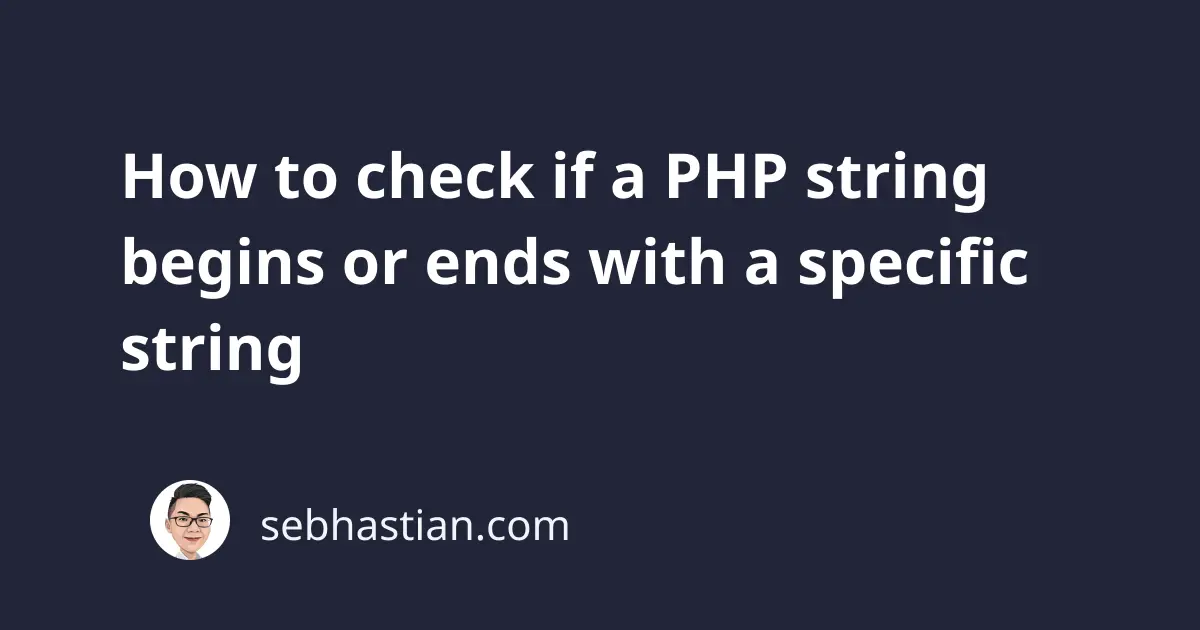
In version 8.0, two functions have been added to PHP that helps in manipulating a string:
- The
str_starts_with()
function allows you to check if a string starts with a specific string - The
str_ends_with()
function allows you to check if a string ends with a specific string
Both functions accept two parameters:
- The
$string
to search in - The
$substring
to search for in the$string
The functions will return a boolean value true
or false
depending on the result.
Here’s how to check if the string Hello World!
starts with he
:
$res = str_starts_with("Hello World!", "Hel");
var_dump($res); // bool(true)
The functions are case-sensitive, so they will return false when the case doesn’t match between the $string
and $substring
.
Here’s an example of checking the end of the string with str_ends_with()
:
$res = str_ends_with("Hello World!", "!");
var_dump($res); // bool(true)
Note that the two functions above won’t be available when you’re using PHP below version 8.0.
You need to create your own custom functions to do the same.
Creating PHP functions to check for a string start and end substrings
In PHP version 7, you will have Call to undefined function error when calling str_starts_with()
and str_ends_with()
functions.
You need to create these functions manually with the help of strlen()
and substr()
functions:
// 👇 PHP 7 str_starts_with() function
function str_starts_with(string $string, string $substring): bool {
// get the length of the substring
$len = strlen($substring);
// just return true when substring is an empty string
if ($len == 0) {
return true;
}
// return true or false based on the substring result
return substr($string, 0, $len) === $substring;
}
Here’s the test result of the str_starts_with()
function above:
str_starts_with("Hello World!", "He"); // true
str_starts_with("Hello World!", "he"); // false
str_starts_with("Hello World!", "H"); // true
For the str_ends_with()
function, you need to change the substr()
parameter.
See the following code snippet:
// 👇 PHP 7 str_ends_with() function
function str_ends_with(string $string, string $substring): bool {
$len = strlen($substring);
if ($len == 0) {
return true;
}
// check the string from the end by adding minus sign
return substr($string, -$len) === $substring;
}
The str_ends_with()
function produces the following result:
str_ends_with("Hello World!", "!"); // true
str_ends_with("Hello World!", "rld!"); // true
str_ends_with("Hello World!", "!!"); // false
With the functions above, you can check if a string starts with or ends with a certain substring in PHP 7.
Good work! 👍