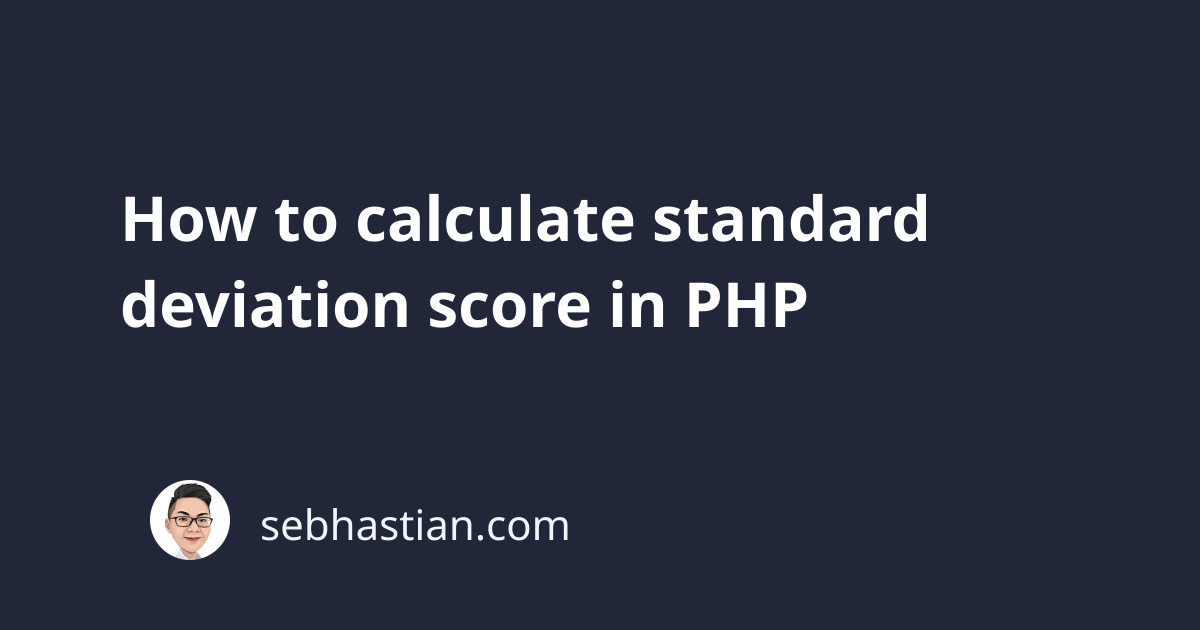
The standard deviation is a score that measures the amount of variation derived from a set of values.
A low standard deviation score means the values are close to the mean score of those values. A high standard deviation score means the values are more spread out.
The formula for standard deviation is as follows:
Std. Deviation = √ Σ|x - μ|2N
To calculate the standard deviation, you need to first find the mean score of the data set (μ).
Next, you calculate the distance between each data and the mean, then square the results (Σ|x - μ|^2).
Finally, get the mean score of the squared differences, then square root them (√Σ/n).
Let’s see how to do the steps above using PHP.
Calculate standard deviation using PHP
Suppose you need to calculate the standard deviation score of the following data set: 5, 8, 12, 10, 5, 8.
There are several PHP functions you can use to help you find the standard deviation score.
First, find the number of elements present in your set using the count()
function:
<?php
$data = [5, 8, 12, 10, 5, 8];
$n = count($data);
Then, count the mean score:
<?php
$data = [5, 8, 12, 10, 5, 8];
$n = count($data);
$mean = array_sum($data)/$n;
Next, you need to calculate the distance between each data and the mean.
Square each result and sum them, then find the mean of those squared differences (this mean of the squared numbers is also known as the variance score)
$distance_sum = 0;
foreach ($data as $i)
{
$distance_sum += ($i - $mean) ** 2;
}
$variance = $distance_sum/$n;
The standard deviation score is just the square root of the variance score, so the last thing you need to do is to call the sqrt()
function:
$std_deviation = sqrt($variance);
And now you have the standard deviation score!
Here’s the complete PHP code for calculating the standard deviation again:
$data = [5, 8, 12, 10, 5, 8];
$n = count($data);
$mean = array_sum($data) / $n;
$distance_sum = 0;
foreach ($data as $i) {
$distance_sum += ($i - $mean) ** 2;
}
$variance = $distance_sum / $n;
$std_deviation = sqrt($variance);
print "RESULT:";
print PHP_EOL;
print "Data set: " . implode(", ", $data);
print PHP_EOL;
print "Count: " . $n;
print PHP_EOL;
print "Sum: " . array_sum($data);
print PHP_EOL;
print "Mean: " . $mean;
print PHP_EOL;
print "Variance: " . $variance;
print PHP_EOL;
print "Standard Deviation: " . $std_deviation;
The code above will produce the following output:
RESULT:
Data set: 5, 8, 12, 10, 5, 8
Count: 6
Sum: 48
Mean: 8
Variance: 6.3333333333333
Standard Deviation: 2.5166114784236
You can create a custom function from the code above.
That way, you can just call the function the next time you need to calculate the standard deviation score.
Alternative: Use the stats package from PECL
PHP has a mathematical package called stats that contains functions for statistical computation.
This package has the stats_standard_deviation()
function that calculates the standard deviation score for you.
You only need to pass the data set you want to calculate as an array to the function:
<?php
$data = [5, 8, 12, 10, 5, 8];
$std_deviation = stats_standard_deviation($data);
print $std_deviation;
// Output: 2.5166114784236
As you can see from the example above, the result is the same as our custom function.
However, the stats package is no longer actively maintained and the latest release is in 2016.
Although this package helps you to do statistical computation, it’s not recommended to use it.
The latest version 2.0.3 failed to install on PHP version 8 as well, so it’s better to create your own functions instead of relying on the stats package.
Now you’ve learned how to calculate the standard deviation score using PHP. Well done!