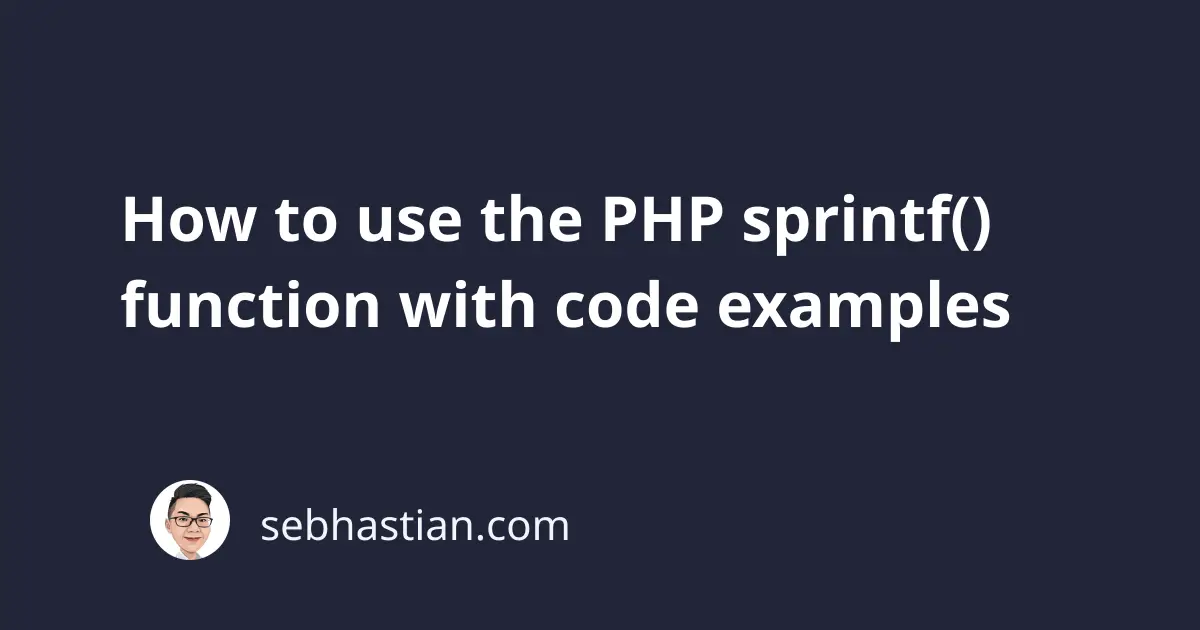
The sprintf()
function in PHP is a string formatting function that allows you to create a string formatted using variables.
sprintf(string $format, mixed ...$values): string
The function takes two or more arguments depending on your requirements:
- The first argument is the
format
string, which contains ordinary characters and directives instructing how the final string should be formatted. - Any number of additional arguments can be included which contain
values
to be included in the string.
For example, suppose you want to format a string to display a person’s name and age. You could use the sprintf()
function like this:
$name = "Nathan";
$age = 30;
$formatted_string = sprintf("%s is %d years old", $name, $age);
echo $formatted_string;
// output: "Nathan is 30 years old"
In the code above, the %s
and %d
directives in the first argument of the sprintf()
function are used to specify that the subsequent arguments should be treated as a string and an integer, respectively.
The sprintf()
function then replaces these directives with the corresponding data from the additional arguments. Finally, it returns the formatted string.
The sprintf()
function also allows you to specify formatting options for the data being inserted into the final string.
For example, you can use the %x
code to format a number as a hexadecimal value, like this:
$number = 255;
$formatted_string = sprintf(
"The hexadecimal value of %d is %x",
$number,
$number
);
echo $formatted_string;
// output: "The hexadecimal value of 255 is ff"
In addition to the %s
and %d
directives, the sprintf()
function supports a wide range of other formatting directives for different data types, including %f
for floating-point numbers, %c
for characters, and %b
for binary numbers.
You can even use complex formatting directives to specify the exact number of decimal places to display for a floating-point number, or the minimum number of digits to display for an integer.
One of the major benefits of the sprintf()
function in PHP is that it allows you to easily format a string with variables.
This can be useful for creating strings that have a consistent structure, such as in the case of generating reports or creating output for display on a website.
For example, you could use sprintf()
to create a string that includes a user’s name and their current account balance, like this:
$name = "Nathan";
$balance = "$100.00";
$output = sprintf("%s has a balance of %s", $name, $balance);
echo $output;
// output: "Nathan has a balance of $100.00"
Another benefit of sprintf()
is that it allows you to specify the format of the output string, including things like the number of decimal places to display for numeric values or the minimum width of a string.
This can be useful for ensuring that your output is consistently formatted and easy to read.
For example, you could use sprintf()
to create a string that includes a user’s account number, formatted with leading zeros like this:
$account_number = 123456;
$output = sprintf("Account number: %08d", $account_number);
echo $output;
// output: "Account number: 00123456"
Overall, the sprintf()
function is a great tool for formatting strings in PHP.
You can learn more about it on the sprintf
documentation page