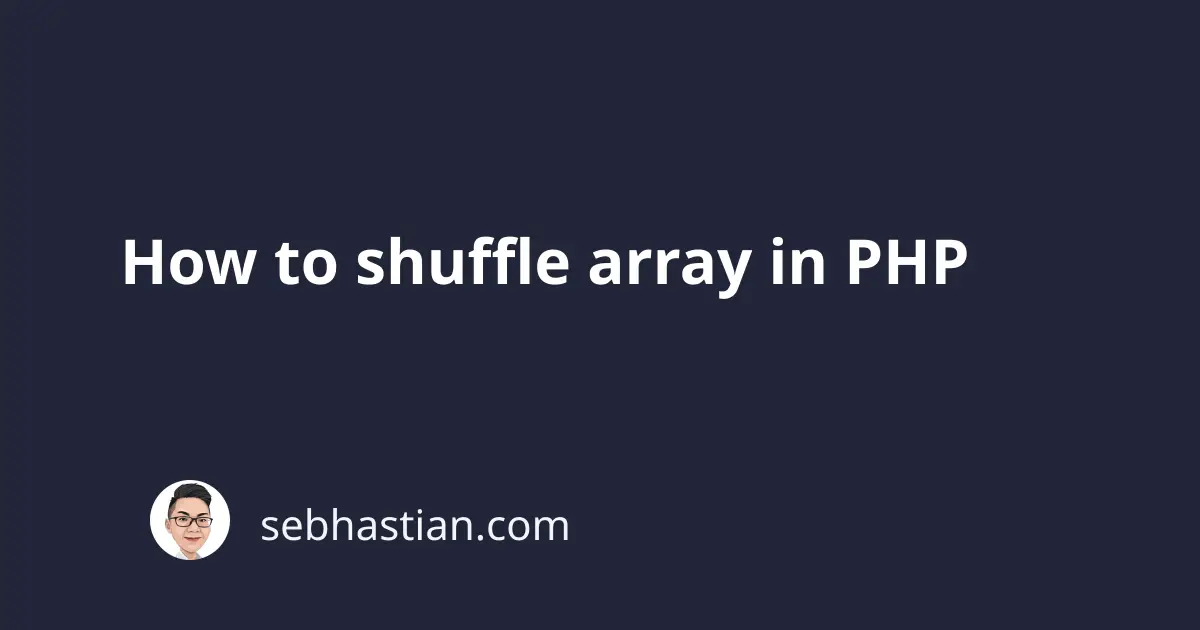
To shuffle arrays in PHP, you can use the provided shuffle()
function.
The shuffle()
function randomizes the order of array elements that you passed as its argument:
shuffle(array &$array): bool
This function modifies the original array variable. It returns boolean true
when the shuffle succeeds, otherwise it returns false
.
Here’s an example of using the shuffle()
array:
<?php
$arr = [1, 2, 3, 4, 5];
shuffle($arr);
print_r($arr);
The print_r()
result is randomized, but here’s one example:
Array
(
[0] => 4
[1] => 1
[2] => 3
[3] => 5
[4] => 2
)
Keep in mind that the shuffle()
function resets the array key pointers.
If you have an associative array, the function will replace the keys with numbers when you call it:
<?php
// shuffle an associative array
$arr = [
"user" => "Nathan",
"age" => 28,
"likes" => ["PHP", "JavaScript"]
];
shuffle($arr);
print_r($arr);
The resulting array will be as shown below:
Array
(
[0] => 28
[1] => Array
(
[0] => PHP
[1] => JavaScript
)
[2] => Nathan
)
To keep the keys of your associative array, you need to use the array_keys()
together with the shuffle()
function.
See the following example:
<?php
$arr = [
"user" => "Nathan",
"age" => 28,
"likes" => ["PHP", "JavaScript"]
];
// 👇 get the array keys
$keys = array_keys($arr);
// 👇 shuffle the keys
shuffle($keys);
// 👇 use foreach to create a new array order
foreach($keys as $k) {
$new_array[$k] = $arr[$k];
}
// 👇 assign new array to array
$arr = $new_array;
// 👇 print the result
print_r($arr);
Here’s the output of the code above:
Array
(
[user] => Nathan
[likes] => Array
(
[0] => PHP
[1] => JavaScript
)
[age] => 28
)
Now you’ve learned how to shuffle arrays in PHP.
Shuffling an associative array is a little more complex than shuffling a regular array.