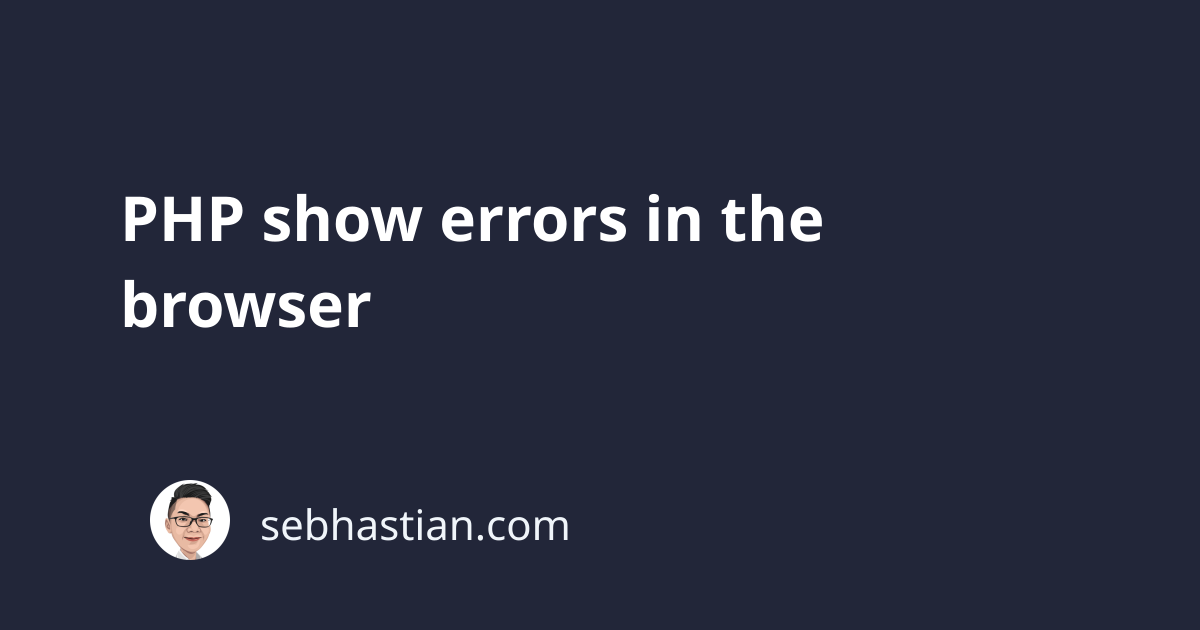
By default, PHP will try to keep all the warnings and errors from showing on your browser.
This is done so that your users won’t see the errors when using your application.
But to fix an issue found in your code, you may need to display the PHP errors and warnings so that you know what is wrong with your application.
This tutorial will help you to display PHP errors on the browser.
Enable error reporting on a PHP file
To display errors and warnings in your browser, you need to set the following directives at the top of your PHP code file:
<?php
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
// your code here...
?>
The ini_set()
function is used to override the configuration in your php.ini
file.
In the above example, the display_errors
and display_startup_errors
configuration is activated by setting their values to true
or 1
.
Usually, these configurations are set to false
to hide the errors and warnings in your PHP code execution.
Finally, the error_reporting()
function determines the error level that will be generated.
There are many different levels to PHP error reporting as follows:
E_ALL
- show all errors and warningsE_ERROR
- show only fatal run-time errorsE_RECOVERABLE_ERROR
- almost fatal run-time errorsE_WARNING
- run-time warnings (non-fatal errors)E_PARSE
- compile-time parse errorsE_NOTICE
- show run-time noticesE_STRICT
- enable PHP to suggest best practice in your codeE_CORE_ERROR
- fatal errors that occur during PHP’s initial startupE_CORE_WARNING
- warnings that occur during initial startupE_COMPILE_ERROR
- fatal compile-time errorsE_COMPILE_WARNING
- compile-time warnings (non-fatal errors)E_USER_ERROR
- user-generated error messageE_USER_WARNING
- user-generated warning messageE_USER_NOTICE
- user-generated notice messageE_DEPRECATED
- warn about features that will be dropped in future PHP versionsE_USER_DEPRECATED
- user-generated deprecation warnings
But usually, only the E_ALL
option is used for debugging your code.
With the three directives set, you should see errors and warnings in your code.
Suppose you have a PHP script as follows:
<?php
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
foreach ($arr as &$value) {
$value = $value * 2;
}
When calling the file from the browser, you will see warning messages because the $arr
is undefined as shown below:
But keep in mind that setting the directives in the PHP file won’t work when you have a parse error.
Enable error reporting from PHP ini file
A parse error happens when your PHP script is wrong at the syntax level, such as missing semicolons in your code.
Suppose you miss a semicolon as shown below:
echo "Hello World!" // 👈 no semicolon
Then the page will fail to load on the browser and it will show an HTTP 500 error code:
To make PHP show display parse errors, you need to change the configuration settings in the php.ini
file.
If you don’t know where the php.ini
configuration file is located, execute the following code from the terminal:
php -i | grep php.ini
You should see the location of your php.ini
file as shown below:
Loaded Configuration File =>
/opt/homebrew/etc/php/8.1/php.ini
Next, open the php.ini
file and set the display_errors
, display_startup_errors
, and error_reporting
configs as shown below:
error_reporting = E_ALL
display_errors = On
display_startup_errors = On
The configs above are exactly what you set using the ini_set()
and error_reporting()
functions.
By setting the configs from the .ini
file, the parse errors won’t cause PHP to return the error 500 code.
Restart your PHP server, then run the same file again. This time, you should see a parse error message as follows:
That’s how you display all PHP errors and warnings using the PHP ini file.
Alternatively, you can also display PHP errors using the .htaccess
configuration.
Display PHP error from the htaccess file
Sometimes, you may not be able to edit the php.ini
file because of restrictions from your hosting provider.
In that case, you can try to add error logging configurations from the .htaccess
file.
The .htaccess
file is a configuration file that you can use to instruct your web server.
This file is usually located in the root directory of your website.
Put the following php_flag
configs in your .htaccess
file:
php_flag display_startup_errors on
php_flag display_errors on
php_value error_reporting -1
Save the configurations, and your web server will follow the .htaccess
instructions the next time you run PHP code.
If that doesn’t work, try restarting your web server.
Now you’ve learned how to get PHP to display errors and warnings. Good work! 👍