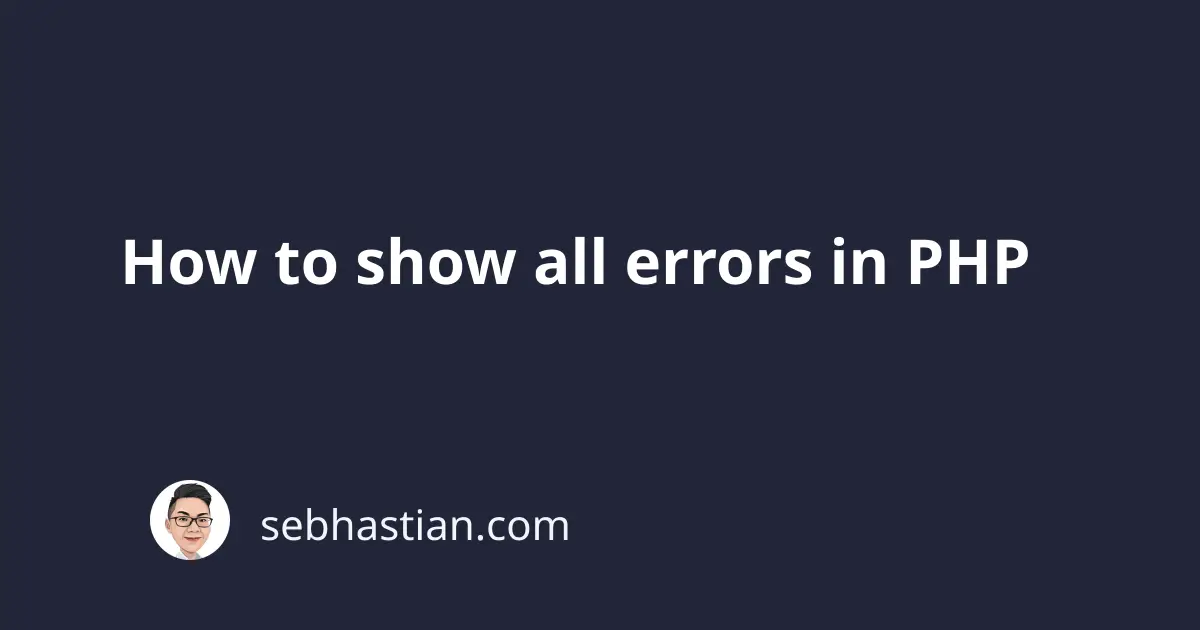
By default, PHP does not display error messages to the user. Instead, it logs them to a file or sends them to an email address.
This is useful in a production environment, as it prevents sensitive information from being exposed to users.
However, in a development environment, it is often helpful to display error messages to the developer so that any code issue can be fixed as soon as possible.
There are several ways to display error messages in PHP
Display error for a single script file
When debugging an error with your PHP application, the quickest way to show all errors is to call ini_set
and error_reporting
functions at the top of your .php
file.
Consider the example below:
<?php
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
// ... rest of your code
The above code will modify the error handling settings for the current file.
The ini_set
function is used to set the value of a configuration option at runtime.
The first ini_set
call sets the display_errors
option to 1
, which enables error messages to be displayed to the user.
The second ini_set
call sets the display_startup_errors
option to 1
, which enables error messages that occur during the PHP startup sequence to be displayed.
The error_reporting
function sets the error reporting level for the current script. In this case, the E_ALL
constant is used, which enables error reporting for all errors and warnings.
Together, these three lines of code enable error reporting and display of error messages for the current file, including errors that occur during startup.
This setting is useful when you know what file is causing an error, as it allows you to see all errors and warnings that occur while the code is running.
When you’re done debugging, don’t forget to remove the code above. Forgetting to do so will make any error gets displayed to your users.
Display error for all script files
When you set the directives using ini_set
and error_reporting
, the configurations only work on the script where you add the code.
To make PHP display error for all script files, you need to set the directives in the php.ini
file.
The php.ini
file is read when PHP starts up, and the settings it contains are used to configure the behavior of PHP.
You can change the error_reporting
and display_errors
in php.ini file with the following directives:
display_errors = on
display_startup_errors = On
error_reporting = E_ALL
The above directives are equivalent to the configurations you set using the ini_set
and error_reporting
functions.
But because they are set in the configuration file level, they will be effective for all PHP scripts you run.
Also, the php.ini
file is server-specific, so the settings it contains will only apply to the server on which it is located.
You can leave the directives enabled on your local development environment, and create another php.ini
file for the production environment with the settings disabled.
Logging error messages
Besides displaying error messages, you can also modify PHP to log any error and warning messages produced by the application.
Logging errors are useful in a production environment because you can record any error that occurs in real time without displaying it to users.
To enable error logging in PHP, you need to set the log_errors
directive to On
in your php.ini
file:
log_errors = On
You can also use ini_set('log_errors', 'On')
but since you don’t want to turn on logging just for a single script, it’s better to configure the option in the ini
file.
PHP will log errors to the default error log file determined by your web server.
You can specify the path to your own log file with the directive:
log_errors = /path/to/error.log
Finally, you can call the error_log()
function in your PHP file whenever you want to log something.
For example, suppose you have a variable that must not be null
. You can send a log when the variable is null
as shown below:
if (!isset($response)) {
error_log("Response is not defined!", 0);
}
The error_log
function accepts four parameters, but you only need to pass the first one, which is the message to log.
The second parameter is the message_type
which indicates what to do with the message:
In the example above, 0
means the message is sent to PHP’s system logger. This is also the default parameter when you didn’t pass any value.
Type 1
is used when you want to send the error log to an email as follows:
error_log("Response is not defined!", 1, "[email protected]");
If you want the error to be logged to a different log file, you can pass 3
as the type:
error_log("Response is not defined!", 3, "/path/to/error.log");
Type 4
parameter sends the message to the SAPI logging handler. SAPI stands for Server Application Programming Interface, and this is rarely used when you develop a web application with PHP.
Conclusion
Displaying and logging errors in a development environment can be useful when debugging and troubleshooting issues with your PHP code.
There are two ways you can display error messages in PHP: by using the ini_set
and error_reporting
functions or by setting directives in the php.ini
file.
Finally, you need to disable error display and reporting in a production environment to prevent sensitive information from being exposed to users. Instead, you need to log the error messages to a file or send them to an email address.
Now you’ve learned how to show all errors in PHP. Good work!