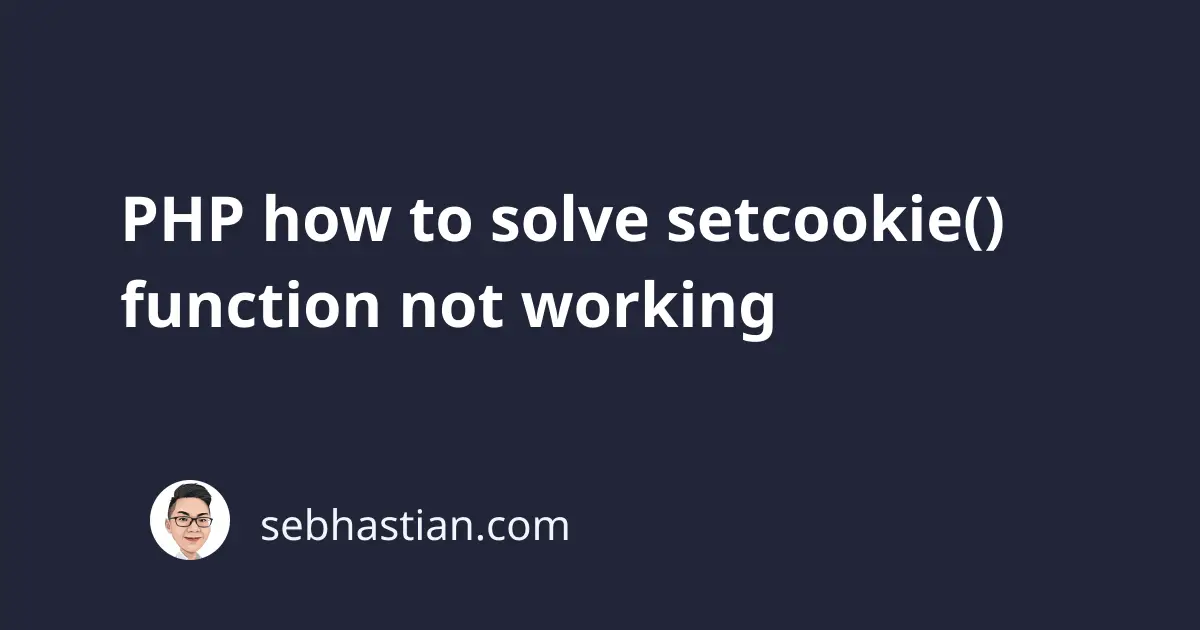
The PHP setcookie()
function is used to create cookie data in your web applications.
Any cookie value you set can then be accessed using the $_COOKIE
global variable.
This tutorial provides solutions to try when the setcookie()
function is not working:
Make sure you refresh the page after setcookie() call
The PHP superglobal variable $_COOKIE
is populated when PHP runs the code.
This means that when you call the setcookie()
function in a PHP script, the $_COOKIE
variable is already assigned.
To access the cookie data, you need to make PHP runs another script by refreshing the page or executing another script.
To test your cookie data, run the script below:
<?php
// ๐ cookie name and value
$name = "username";
$value = "Nathan";
// ๐ set cookie for 1 day (86400 seconds)
setcookie($name, $value, time() + 86400, "/");
?>
<html>
<body>
<?php // ๐ check if cookie exists and print the value
if (!isset($_COOKIE[$name])) {
print "Cookie called '" . $name . "' is not set";
} else {
print "Cookie '" . $name . "' has been set<br>";
print "Value: " . $_COOKIE[$name];
} ?>
</body>
</html>
The first time you load the page above, PHP should respond with โCookie called โusernameโ is not setโ.
Refresh the page, and you will see the following response:
Cookie 'username' has been set
Value: Nathan
This is because PHP populates the $_COOKIE
variable before running the script.
If you need the cookie data to be accessible immediately, you can manually assign the cookie values to the $_COOKIE
variable:
// ๐ cookie name and value
$name = "username";
$value = "Nathan";
// ๐ set cookie for 1 day (86400 seconds)
setcookie($name, $value, time() + 86400, "/");
// ๐ manually set the cookie
$_COOKIE[$name] = $value;
Now you can access the cookie data immediately without sending another request to the server.
Make sure setcookie() is called before any output
The setcookie()
function modifies the HTTP headers to include that cookie in your request.
This means that the function must be called before you produce any output in your script.
An output can be raw HTML or the echo
/ print
construct in your PHP file.
Suppose you have a PHP script as shown below:
<?php
echo "Hello";
setcookie("username", "Nathan", time() + 3600, "/");
?>
Then PHP will produce a warning in the output as follows:
Warning: Cannot modify header information - headers already sent by ...
The same warning appears when you have raw HTML output before calling setcookie()
:
<html>
<body>
<h1>Hello World!</h1>
<body>
<html>
setcookie("username", "Nathan", time() + 3600, "/");
To solve this warning, you need to call the setcookie()
function before any output:
<?php
setcookie("username", "Nathan", time() + 3600, "/");
echo "Hello";
?>
This way, the HTTP headers will have the cookie data set using setcookie()
.
You still need to send another request to access the cookie with $_COOKIE
though.
Output buffering to set the cookie after output
If you really need to set the cookie after some output code, then you can use the output buffering function.
The output buffering function command PHP to store any output in the buffer until you manually release it.
The function to start output buffering is ob_start()
and the function to release the buffer is ob_end_flush()
.
Hereโs how you buffer output in PHP:
<?php
// ๐ buffer the output
ob_start();
echo "Hello World!";
// ๐ set the cookie
setcookie("username", "Nathan", time() + 3600, "/");
// ๐ release the buffer
ob_end_flush();
This solution works because PHP wonโt send the HTTP headers until you release the buffer (PHP sends HTTP headers along with the output)
And now youโve learned what to do when the setcookie()
function is not working.
I hope this tutorial has been helpful to you. ๐