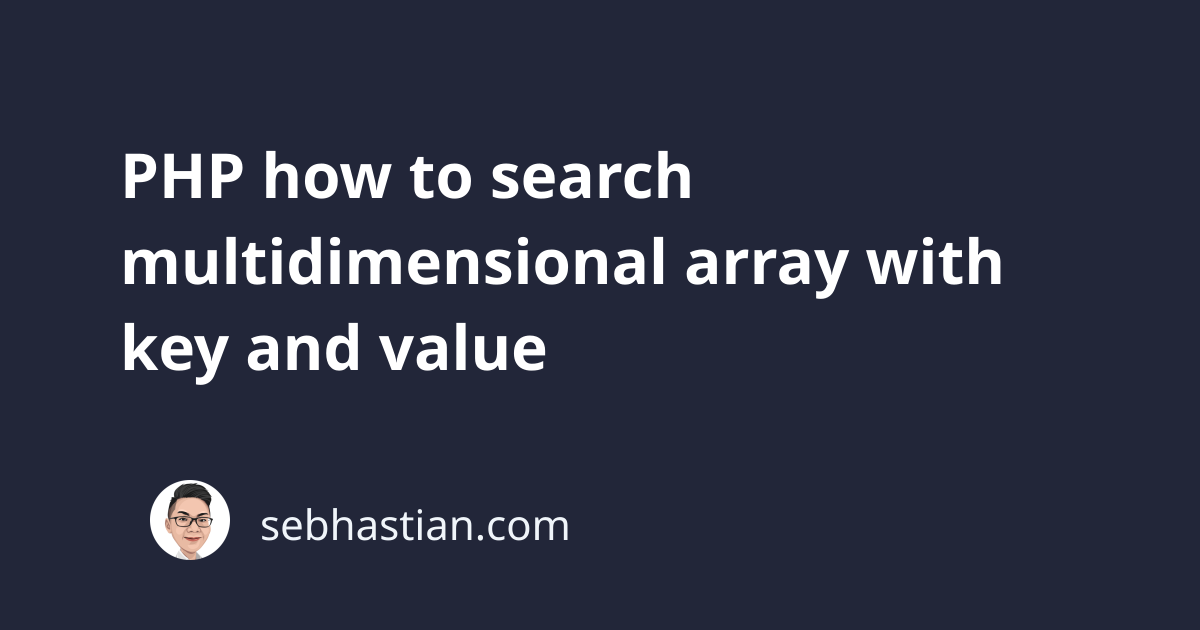
To handle searching a multidimensional array, you can use either the foreach
statement or the array_search()
function.
A PHP multidimensional array can be searched to see if it has a certain value.
Let’s see an example of performing the search. Suppose you have a multidimensional array with the following structure:
$users = [
[
"uid" => "111",
"name" => "Nathan",
"age" => 29,
],
[
"uid" => "254",
"name" => "Jessie",
"age" => 25,
],
[
"uid" => "305",
"name" => "Michael",
"age" => 30,
],
];
To search the array by its value, you can use the foreach
statement.
You need to loop over the array and see if one of the child arrays has a specific value.
For example, suppose you want to get the array with the uid
value of 111
:
$id = "111";
foreach ($users as $k => $v) {
if ($v["uid"] === $id) {
print $users[$k];
}
}
Note that the comparison operator in the code above uses triple equal ===
.
This means the type of compared values must be the same.
The code above will produce the following output:
Array
(
[uid] => 111
[name] => Nathan
[age] => 29
)
In PHP 5.5 and above, you can also use the array_search()
function combined with the array_column()
function to find an array that matches a condition.
See the example below:
$name = "Michael";
$key = array_search($name, array_column($users, "name"));
print_r($users[$key]);
The above code will produce the following output:
Array
(
[uid] => 305
[name] => Michael
[age] => 30
)
Let’s create a custom function from the search code so that you can perform a more dynamic search based on key and value.
This custom function accepts three parameters:
- The
key
you want to search - The
value
you want the key to have - The
array
you want to search
The function can be written as follows:
function find_array($name, $value, $array) {
$key = array_search($name, array_column($array, $value));
return $array[$key];
}
To handle a case where the specific value is not found, you need to add an if
condition to the function.
You can return false
or null
when the $key
is not found:
function find_array($k, $v, $array) {
$key = array_search($v, array_column($array, $k));
// 👇 key is found, return the array
if ($key !== false) {
return $array[$key];
}
// 👇 key is not found, return false
return false;
}
Now you can use the find_array()
function anytime you need to search a multidimensional array.
Here are some examples:
// 👇 value exists
$result = find_array("name", "Jessie", $users);
if ($result) {
print_r($result);
} else {
print "Array with that value is not found!";
}
// 👇 value doesn't exists
$result = find_array("uid", "1000", $users);
if ($result) {
print_r($result);
} else {
print "Array with that value is not found!";
}
The code above will produce the following output:
Array
(
[uid] => 254
[name] => Jessie
[age] => 25
)
Array with that value is not found!
Now you’ve learned how to search a multidimensional array in PHP.
When you need to find an array with specific values, you only need to call the find_array()
function above.
Feel free to use the function in your PHP project. 👍