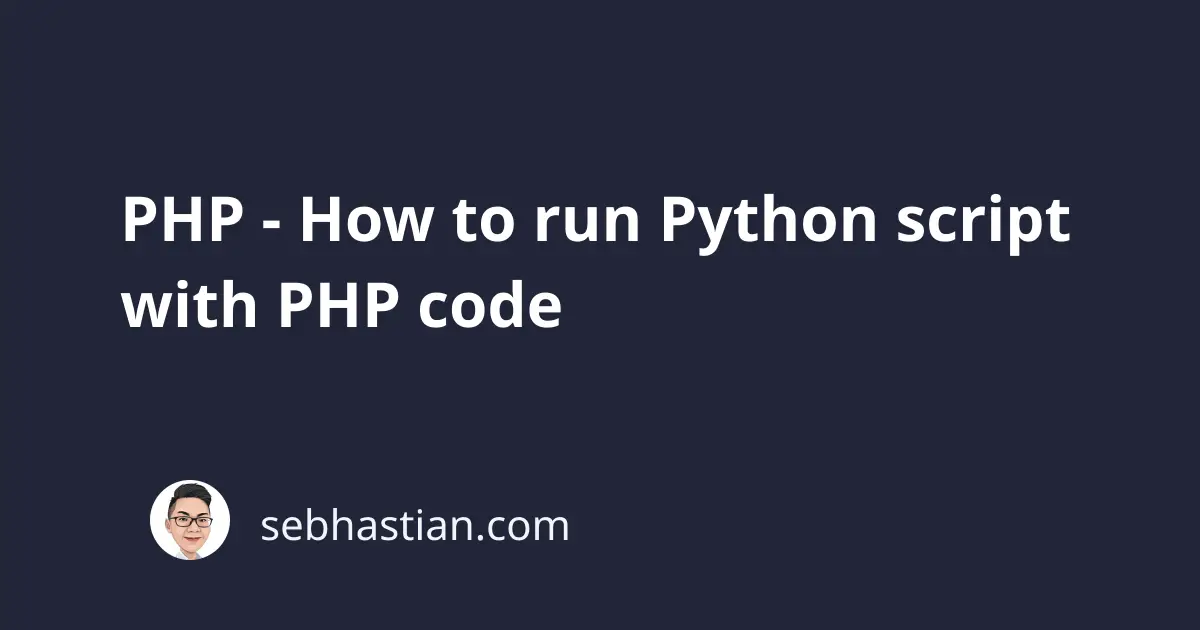
To run a Python script using PHP, you need to call the shell_exec()
function.
The shell_exec()
function allows you to run a command from the shell (or terminal) and get the output as a string.
Since the function runs a command from the shell, you need to have Python installed and accessible from your computer.
PHP can’t run Python scripts directly. It just passes a command to the shell to run a Python script.
For example, suppose you have a hello.py
script with the following code:
print("Hello from Python 3")
To run the script above, you need to write the following code to your PHP file:
<?php
$output = shell_exec("python hello.py");
echo $output;
The Python script that you want to run needs to be passed as an argument to the shell_exec()
function.
The echo
construct will print the output of the script execution.
If you see the shell responds with python: command not found
, then that means the python
program can’t be found from the shell.
You need to make sure that the python
program can be found by running the which
command as follows:
$ which python
/usr/bin/python
You may also have a Python interpreter saved as python3
, which is how Python is installed in the latest macOS version.
In this case, you need to run the script using python3
in the shell_exec()
function:
<?php
$output = shell_exec("python3 hello.py");
echo $output;
In a UNIX environment, you can also specify the Python interpreter in the .py
file as the shebang line.
Write the interpreter you want to use for the script as follows:
#!/usr/bin/env python
print("Hello from Python 3")
With the shebang line defined, you can remove the python
runner from the shell_exec()
function:
<?php {hl_lines=[1]}
$output = shell_exec("./hello.py");
echo $output;
Now run the PHP script. You should see the same output as when you add the runner to the shell_exec()
function.
Sometimes you may see the shell responds with permission denied as follows:
$ php test.php
sh: ./hello.py: Permission denied
This means that the PHP runner doesn’t have the execute permission for the Python script that you want to run.
To fix this, you need to add the execute permission to the Python script with chmod
like this:
chmod +x hello.py
When you run the above command from the shell, the execute permission (x
) will be added to the file.
And that’s how you can run Python script with PHP. Nice! 👍