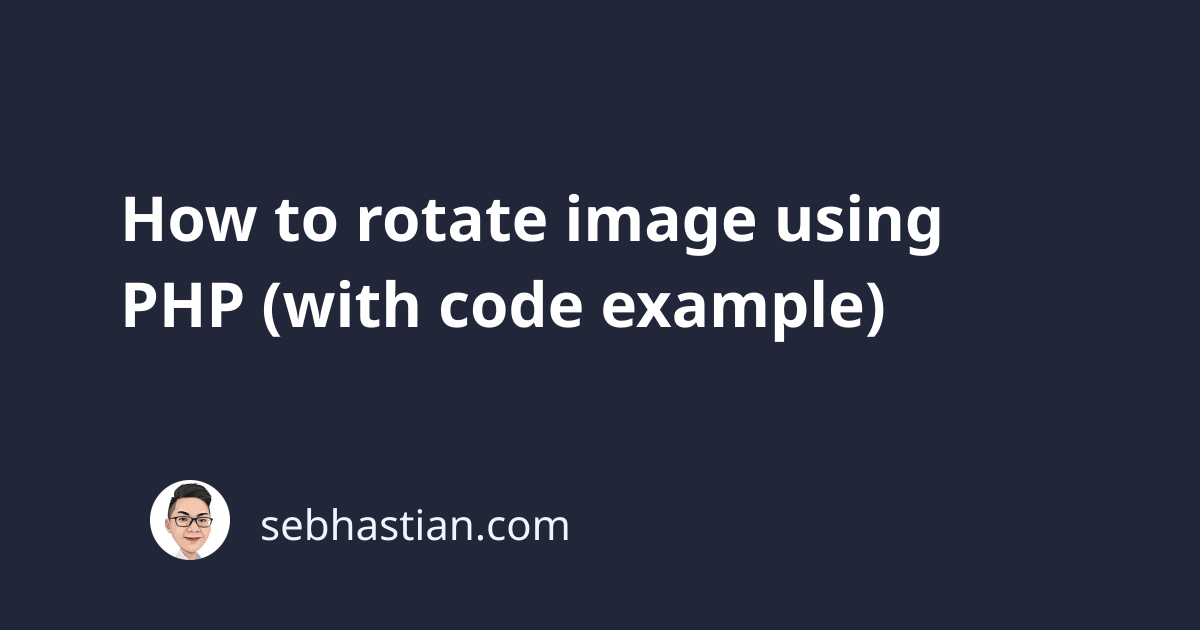
To rotate an image using PHP, you need to use the built-in imagerotate()
function.
This function allows you to rotate an image in a counterclockwise degree.
To get the image into PHP, you need to use the imagecreatefromjpeg()
or imagecreatefrompng()
function.
Here’s an example of rotating an image 90 degrees:
// define path to your image
$filename="./assets/image.jpg";
// Load the image as jpeg
$source = imagecreatefromjpeg($filename);
// Rotate 90 degrees
$rotate = imagerotate($source, 90, 0);
// Save the image as jpeg to your system
imagejpeg($rotate, "rotated-image.jpg");
// Destroy loaded image to free memory
imagedestroy($source);
Here’s an example of the image output. Image from Unsplash:
When you have a jpg or jpeg file, use the imagecreatefromjpeg()
to load the image and imagejpeg()
to save it.
When you have a png file, use the imagecreatefrompng()
to load the image and imagepng()
to save it.
The imagerotate()
function only goes counterclockwise (to the left) so keep that in mind as you specify the rotation angle.
And that’s how you rotate an image using PHP. Good work!