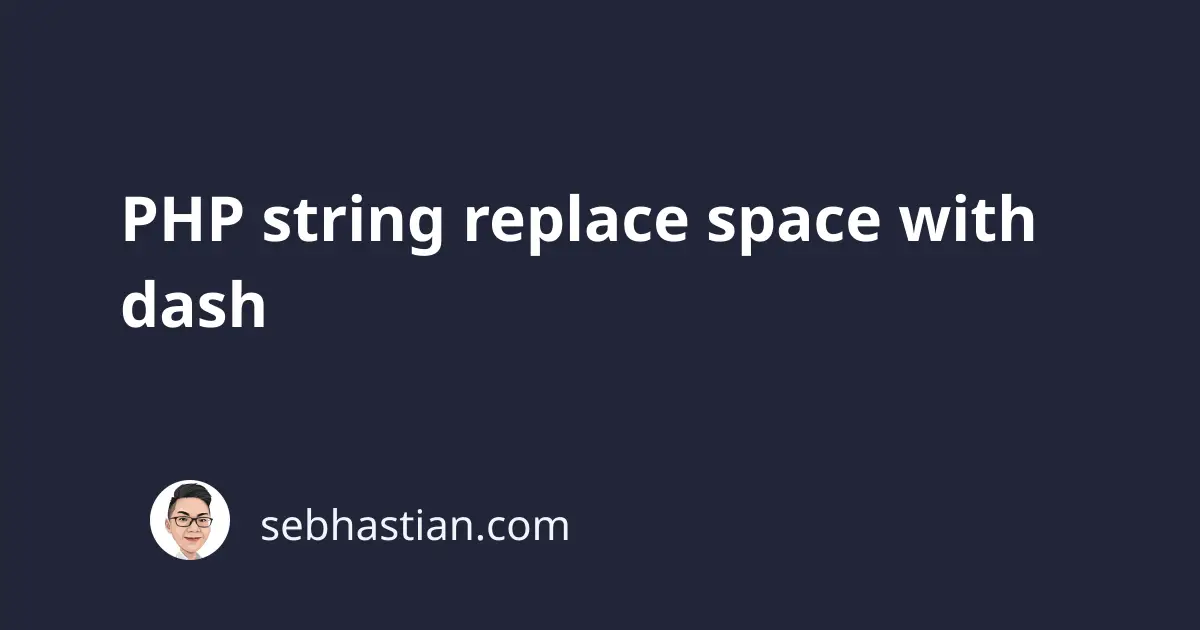
To replace space with dash or hyphen in PHP, you can use the str_replace()
function.
The str_replace()
function accepts three parameters:
- The characters or string you want to replace
- The characters or string to replace the existing characters
- The string to search for the characters
This function works recursively, which means it will look for all occurrences of the first parameter instead of stopping after one occurrence.
Consider the following example:
$str = "php replace space with dash";
$str = str_replace(" ", "-", $str);
echo $str; // php-replace-space-with-dash
If youโre making a URL, you may also want to convert the string to lowercase.
You can call the strtolower()
function to the string as shown below:
// ๐ convert string to lowercase before replace
$str = "PHP replace Space with Dash";
$str = strtolower($str);
$str = str_replace(" ", "-", $str);
echo $str; // php-replace-space-with-dash
// ๐ shorten the code above
$str = "PHP replace Space with Dash";
$str = str_replace(" ", "-", strtolower($str));
echo $str; // php-replace-space-with-dash
When you need to replace dash with space, you only need to reverse the first two parameters of the str_replace()
function:
$str = "php-replace-dash-with-space";
$str = str_replace("-", " ", $str);
echo $str; // php replace dash with space
You may see some people recommend using preg_replace()
function to replace space with dash.
While you can do so, the preg_replace()
function consumes more memory and time to perform the same operation.
Use preg_replace()
only when you have multiple spaces that you want to convert into a single dash as follows:
// ๐ str_replace multiple dash for multiple spaces
$str = "php replace space with dash";
$str = str_replace(" ", "-", $str);
echo $str; // php-replace-space--with---dash
// ๐ use preg_replace to replace multiple spaces with a single dash
$str = "php replace space with dash";
$str = preg_replace('/[[:space:]]+/', '-', $str);
echo $str; // php-replace-space-with-dash
Only use preg_replace()
when your string may have more than one space per word as shown above.
And thatโs how you replace space with dash in a PHP string. Nice work! ๐