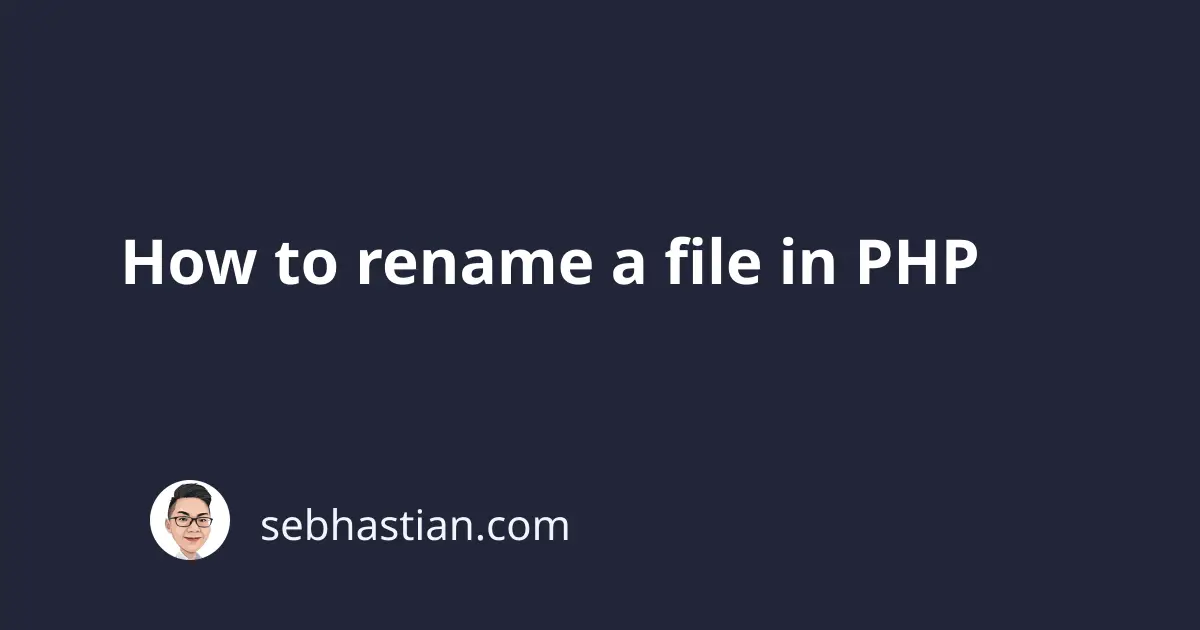
To rename a file in PHP, you need to call the rename()
function.
The PHP rename()
function allows you to rename a file or a directory by passing the old and new names for that file or directory.
The syntax of rename()
is as follows:
rename(
string $from,
string $to,
?resource $context = null
): bool
The parameters of the functions are:
- The
$from
string specifies the file you want to change - The
$to
string specifies the new file name - The
$context
for a custom context stream. It’s optional and not needed most of the time
For example, the code below will rename index.php
to server.php
:
<?php
rename("index.php", "server.php");
The rename()
function will move the file between directories when necessary.
Suppose you have the following file structure for your project:
files
└── project
└── index.php
When you rename the index.php
file using the following code:
<?php
rename("project/index.php", "server.php");
Then the index.php
file will be moved from the project
folder.
The new file structure will be as shown below:
files
├── project
└── server.php
Without passing the location path, PHP will move the file to the current working directory (where you run the code from)
To avoid getting your file moved by the rename()
function, you need to pass the same path in the $from
and $to
parameters.
<?php
rename("project/index.php", "project/server.php");
The above code will rename the index.php
file inside the project
folder to server.php
without moving it.
And that’s how you rename a file in PHP using the rename()
function. Easy, right?