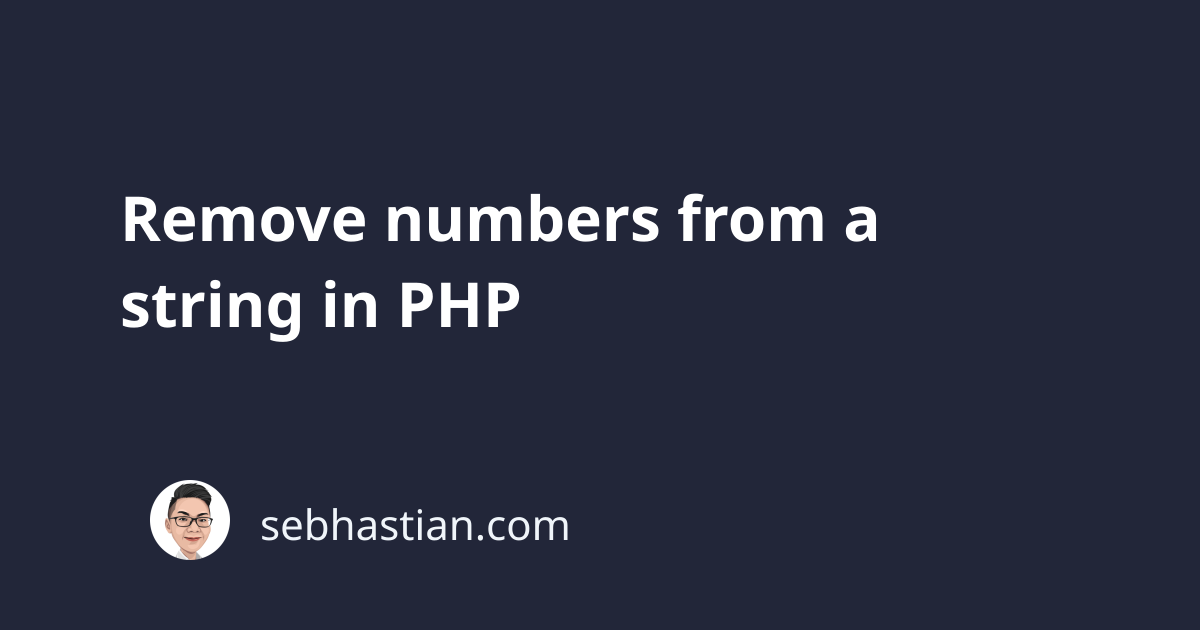
To remove numbers from a PHP string, you can use the str_replace()
or preg_replace()
function.
The str_replace()
function allows you to replace all occurrences of the search string with the replacement string.
This function allows you to pass an array
as the search string as well. To remove numbers from a string, use str_replace()
like this:
<?php
$string = "123Remove9893 Numbers 7654";
$numbers = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9];
// 👇 replace numbers with empty string
$result = str_replace($numbers, "", $string);
print $result; // "Remove Numbers "
?>
But str_replace()
is limited to the numbers you put in the array.
If you want to remove numbers in all numeric systems, then it’s better to use preg_replace()
instead.
The regex to match all numbers is /\d/u
, so use it in preg_replace()
like this:
<?php
// 👇 string with Brahmi numerals
$a = "𑁫𑁪Hello𑁧𑁨𑁩";
// 👇 string with Bengali numerals
$b = "১২Happy৫৭";
print preg_replace("/\d/u", "", $a);// "Hello"
print PHP_EOL;
print preg_replace("/\d/u", "", $b);// "Happy"
Now that you know how to remove numbers from a string, you can create a custom function for this purpose.
Let’s name the function remove_numbers()
:
function remove_numbers(string $str){
return preg_replace("/\d/u", "", $str);
}
Anytime you need to remove numbers from a string, just call the function:
// 👇 more examples
$a = "123Hel90௭lo𑁧𑁨𑁩";
$b = "௭Happy৫৭";
print remove_numbers($a);// "Hello"
print PHP_EOL;
print remove_numbers($b);// "Happy"
Now you’ve learned how to remove numbers from a string in PHP. Isn’t that easy? 😉