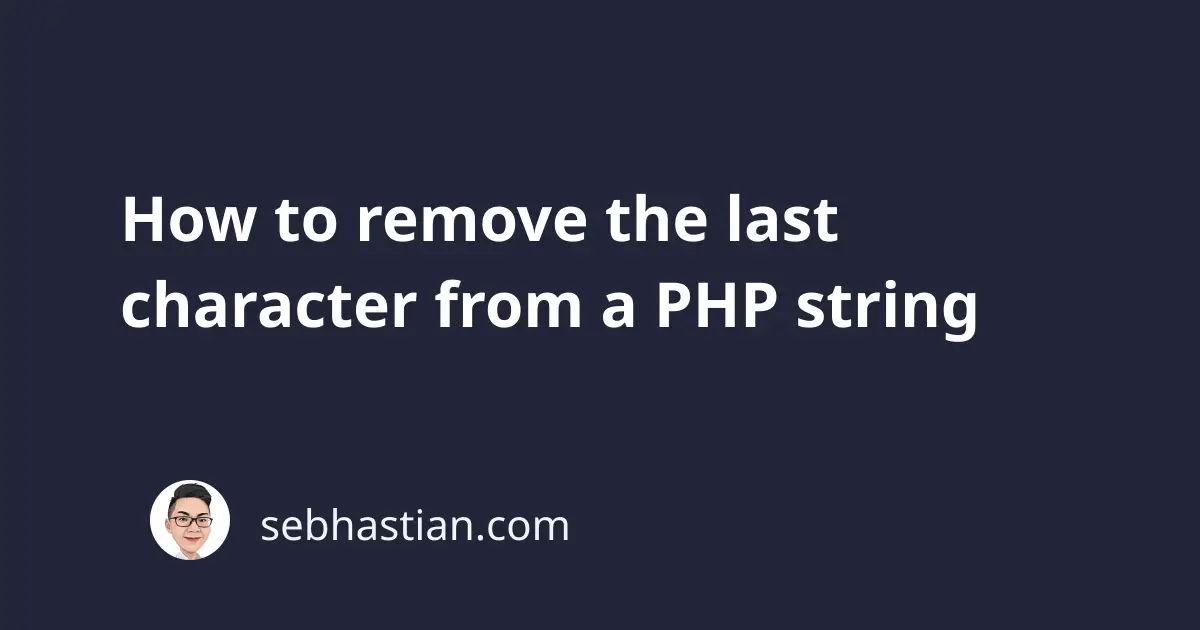
Removing the last character from a string in PHP is a simple task that can be achieved in a variety of ways.
There are three ways you can remove the last character from a string in PHP:
These three solutions are easy, and you will learn them in this tutorial.
Use substr() function
The substr()
function allows you to extract a portion of a string based on a starting position and a length.
To remove the last character from a string using substr()
, you need to specify the starting position as 0
and the length as -1
(the second and third arguments respectively).
Consider the example below:
$string = "Hello World!!!";
// extract all characters except the last one
$new_string = substr($string, 0, -1);
echo $new_string; // "Hello World!!"
The code above works because the -1
index in PHP string equals the first character from the end of the string.
That’s why the last bang symbol !
is removed from the string.
Use rtrim() function
Another way to remove the last character from a string in PHP is to use the rtrim()
function. This function allows you to remove specified characters from the end of a string.
To remove the last character, you need to specify the character you want to remove as the second argument to rtrim()
.
Here is an example:
$string = "Hello World!!!";
// specify the last character as the second argument
$new_string = rtrim($string, "!");
echo $new_string; // "Hello World"
Notice how the three bang symbols in the string above is removed by the rtrim()
function.
This function removes all matching characters from the end of the string, making it useful when you have several strings where you want to remove the last character(s).
Use the preg_replace() function
Finally, another way to remove the last character from a string in PHP is to use the preg_replace()
function.
The function allows you to search for and replace patterns in a string using regular expressions.
To remove the last character from a string using preg_replace()
, you can use the regular expression "/.$/"
, which matches any character at the end of the string.
Here is an example:
$string = "Developer";
// Remove the last character from the string
$new_string = preg_replace("/.$/", "", $string);
// Output the new string
echo $new_string; // "Develope"
As you can see, there are multiple ways to remove the last character from a string in PHP.
Whether you use substr()
, rtrim()
, or preg_replace()
, the process is fairly straightforward and can be easily incorporated into your PHP scripts.
You can select the solution that fits your requirements.