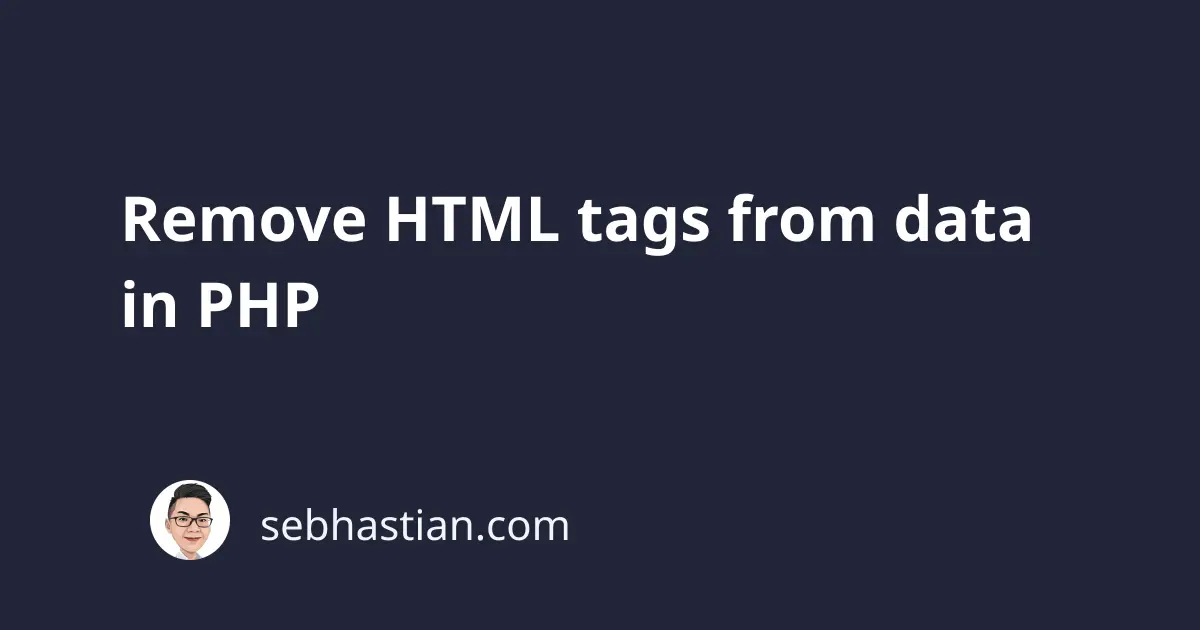
To remove HTML tags from a PHP string, you need to use the strip_tags()
function.
The strip_tags()
function is used to strip HTML and PHP tags from a string.
The syntax is as follows:
strip_tags(
string $string,
array|string|null $allowed_tags = null
): string
The function accepts two parameters:
- The
$string
to remove tags from (required) - The
$allowed_tags
for tags to ignore (optional)
This function returns the parameter $string
with the tags removed.
Hereโs an example of using the function:
// ๐ remove <h1> tag
$str = "<h1>Hello World!</h1>";
echo strip_tags($str); // Hello World!
// ๐ remove <p> and <br> tag
$str = "<p>Hi! <br>My name is Nathan</p>";
echo strip_tags($str); // Hi! My name is Nathan
// ๐ allow <p> tag but remove others
$str = "<p>Hi! <br><strong>My name is <span>Nathan</span><strong></p>";
echo strip_tags($str, "<p>"); // <p>Hi! My name is Nathan</p>
Calling the strip_tags()
function is the easiest way to remove HTML tags from a PHP string.