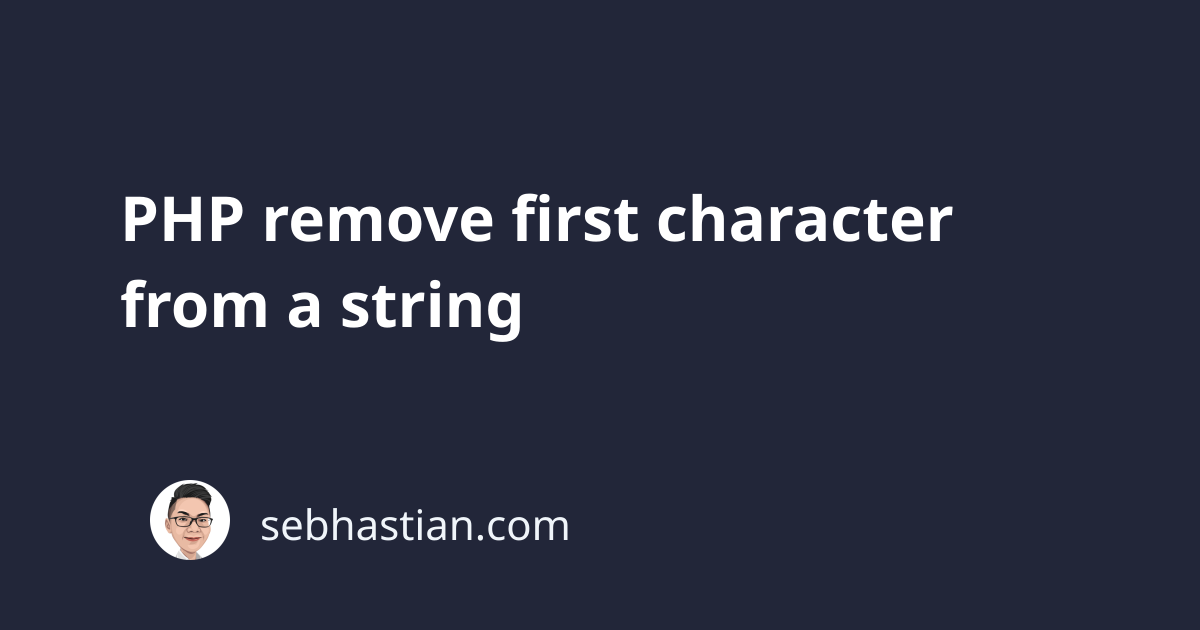
To remove the first character from a string, you can use the ltrim()
or substr()
function.
The ltrim()
function is used to strip whitespace from the beginning of a string.
To use the function, you need to pass the string and the character(s) to remove.
Consider the example below:
<?php
$str = "-Hello World!";
// π remove the dash in front of H
$new_string = ltrim($str, "-");
print $new_string; // Hello World!
?>
But keep in mind that the ltrim()
function removes all occurrences of the character(s) you specified as its second parameter:
<?php
$str = "---Hello World!";
// π remove dashes in front of H
$new_string = ltrim($str, "-");
print $new_string; // Hello World!
If you explicitly want to remove only the first character, you need to use the substr()
function instead.
The substr()
function is used to return a portion of a string. You can use it to cut the first character as shown below:
<?php
$str = "---Hello World!";
// π remove the first dash in front of H
$new_string = substr($str, 1);
print $new_string; // --Hello World!
?>
The second parameter of the substr()
function determines the offset position where the function begins to cut the string.
Because a string index starts from 0
, passing 1
as the offset position will cause the function to remove the first character from the string.
And thatβs how you remove the first character in PHP. Nice! π