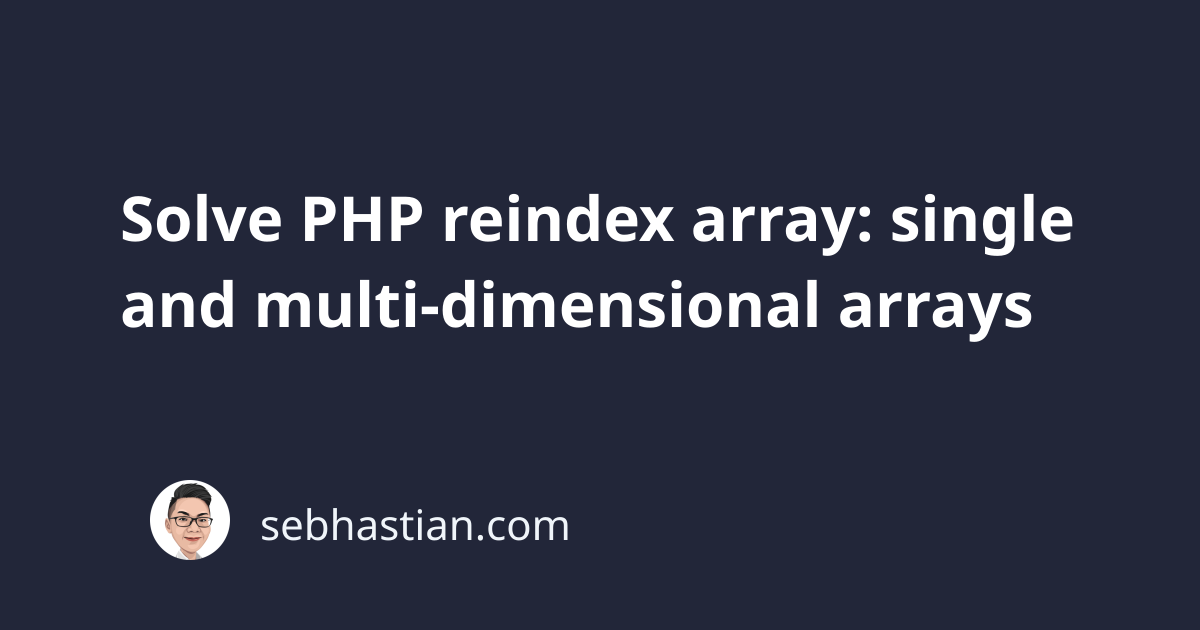
Sometimes, your PHP code will create an array that has gaps in its keys as shown below:
Array
(
[1] => Nathan
[6] => Jack
[10] => Jessie
)
In the above example, the array keys are unordered as it goes from 1
to 6
to 10
.
To reindex the array keys of the array, you need to call the arra_values()
function on your array.
See the code example below:
<?php
$arr = [
1 => "Nathan",
6 => "Jack",
10 => "Jessie",
];
$arr = array_values($arr);
print_r ($arr);
The print_r
output will be:
Array
(
[0] => Nathan
[1] => Jack
[2] => Jessie
)
Reindex a multi-dimensional array
The array_values()
only works for a single-dimension array.
When you need to reindex a multi-dimensional array, you need to call the array_map()
function and pass array_values
as its first parameter:
<?php
$arr = [
1 => [
4 => "Nathan",
5 => "PHP developer"
],
6 => [
4 => "Jack",
5 => "JavaScript developer"
]
];
// 👇 reindex the child arrays
$arr = array_map("array_values", $arr);
print_r ($arr);
The output will be:
Array
(
[1] => Array
(
[0] => Nathan
[1] => PHP developer
)
[6] => Array
(
[0] => Jack
[1] => JavaScript developer
)
)
Note that only the child arrays are being reindexed with the array_map()
.
This is because the array_map()
function runs the array_values()
function for each child element of the array.
To reindex both parent and child arrays, you need to call array_values()
again:
$arr = array_map("array_values", $arr);
$arr = array_values($arr);
Now both parent and child array keys will be reset:
(
[0] => Array
(
[0] => Nathan
[1] => PHP developer
)
[1] => Array
(
[0] => Jack
[1] => JavaScript developer
)
)
But keep in mind that this trick won’t work when you have an unstructured array.
Suppose you have an array with the following structure:
$arr = [
1 => [
4 => "Nathan",
5 => "PHP developer"
],
6 => [
4 => "Jack",
5 => "JavaScript developer"
],
10 => "Jessie",
];
Calling the array_map()
function will result in a fatal error:
PHP Fatal error: Uncaught TypeError: array_values():
Argument #1 ($array) must be of type array, string given in ...
Stack trace:
#0 [internal function]: array_values('Jessie')
To reindex an unstructured multi-dimensional array, you need to create a custom function.
This function needs to loop over the array and call array_values()
on all values that are an array
type.
The function below should be good:
function array_reset(array $arr)
{
$arr = array_values($arr);
foreach ($arr as $k => $v) {
if (is_array($v)){
$v = array_reset($v);
$arr[$k] = $v;
}
}
return $arr;
}
Let’s test running the arra_reset()
function:
<?php
$arr = [
1 => [
4 => "Nathan",
5 => "PHP developer"
],
6 => [
4 => "Jack",
5 => "JavaScript developer"
],
10 => "Jessie",
];
// 👇 reindex the array
$arr = array_reset($arr);
print_r ($arr);
The code above produces the following output:
Array
(
[0] => Array
(
[0] => Nathan
[1] => PHP developer
)
[1] => Array
(
[0] => Jack
[1] => JavaScript developer
)
[2] => Jessie
)
As you can see, both parent and child arrays are reindexed using the array_reset()
function.
This function is also able to reset the index of unstructured arrays, where the array value can be a non-array.
Feel free to use the array_reset()
function in your code.
Now you’ve learned how to reindex a PHP array and reset its keys. Great job! 👍