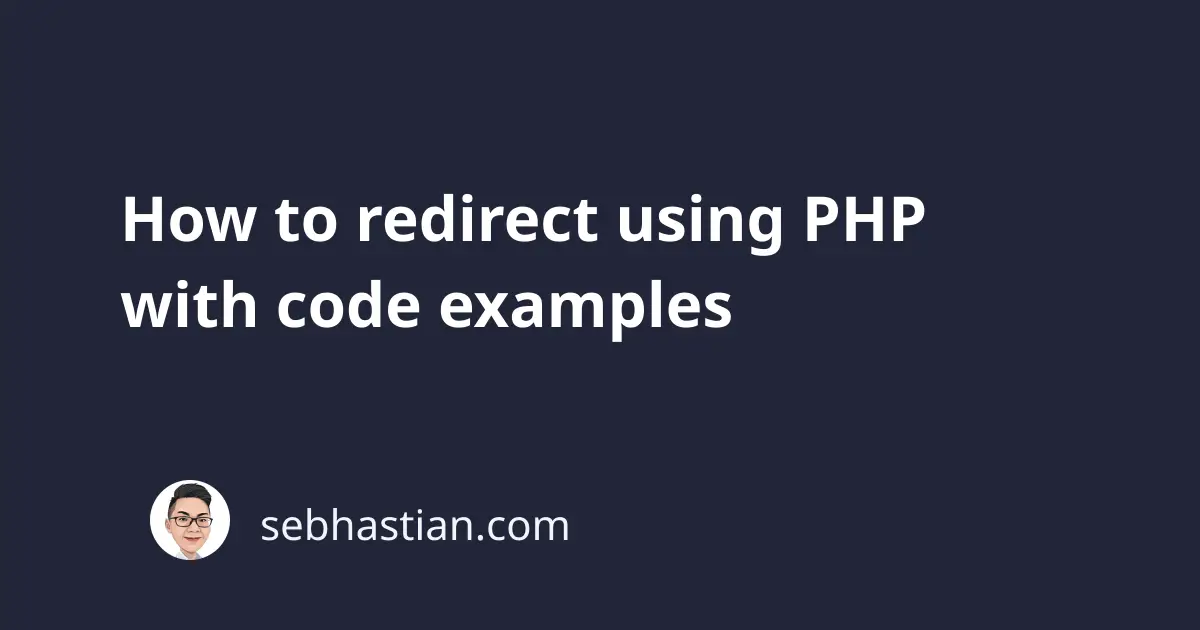
It’s often useful to redirect users to a different page after they submit a form or perform some other action on your website.
To redirect a user to a new page using PHP, you can use the header()
function and specify the Location
header with the URL of the page you want to redirect the user to.
Consider the example below:
<?php
// redirect the user to the thank you page
header("Location: thanks.php");
// make sure any code below does not get executed
exit;
?>
It’s important to note that the header()
function must be called before any output is sent to the browser.
The exit
function can be called so that any code below the header()
function doesn’t get executed.
If you are redirecting to a different domain, you need to specify the absolute URL to the new domain:
<?php
// redirect the user to a new domain
header("Location: http://www.yournewdomain.com/");
?>
You can also specify a status code with the header function to indicate the type of redirect that’s being performed.
For example, to perform a permanent redirect (status code 301), you can use the following code:
<?php
// redirect the user to the new domain permanently
header("Location: http://www.yournewdomain.com/", true, 301);
?>
Finally, you redirect to a file with another extension.
For example, here’s how to redirect to a .html
file:
<?php
// redirect the user to a file
header("Location: http://www.domain.com/index.html");
?>
You can redirect to files of any type: .html
, .php
, .js
, .png
, .jpg
, .mp3
, .mp4
, etc.
I hope this helps you get started with redirecting users to a new page with PHP. Nice work!