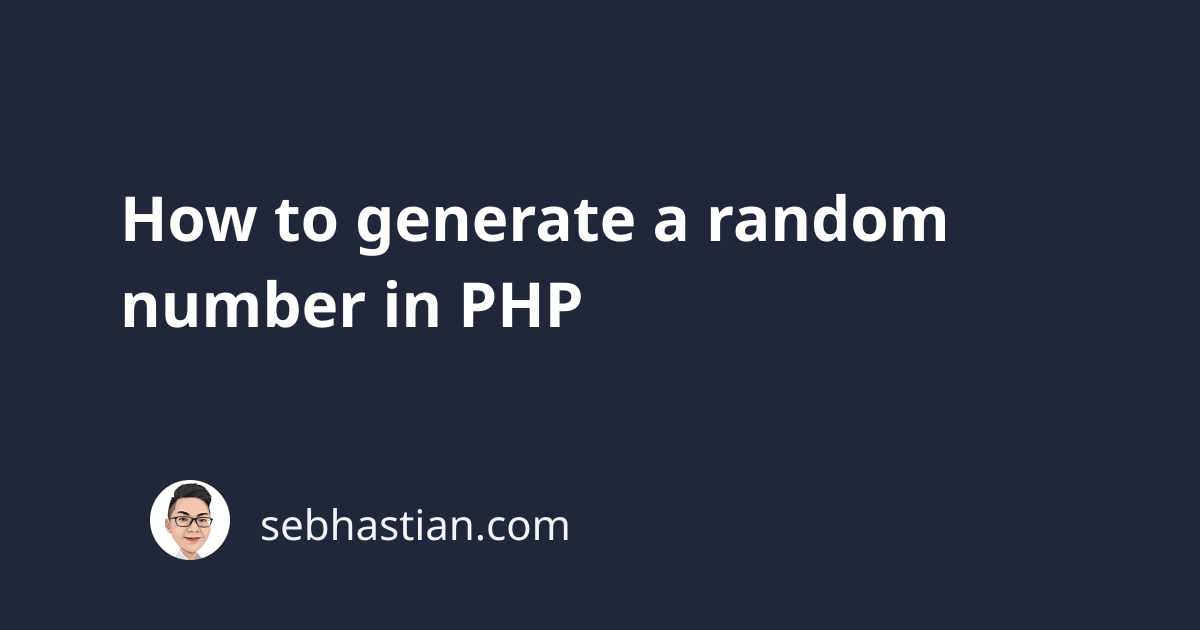
To generate a random number using PHP, you need to call the rand()
function in your script.
The rand()
function returns an int
integer number between 0
and getrandmax()
function result.
Let’s call the rand()
function and print the value using echo
:
echo rand(); // 978288474
echo rand(); // 1344295660
You will see a different number printed for each time you call the rand()
function.
You can set the minimum and maximum number the rand()
function will generate by passing the $min
and $max
arguments:
rand(int $min, int $max): int
For example, to random between 10
to 30
:
echo rand(10, 30); // random between 10 to 30
You can also generate a random negative number as shown below:
echo rand(-39, -12); // random between -39 to -12
The rand()
function has inclusive behavior, which means the $min
and $max
numbers are included in the random selection.
In PHP version 7.1, the rand()
function is an alias for the mt_rand()
function.
The mt_rand()
function is generates a random number using the Mersenne Twister algorithm, which has been tested to be faster and gives more random results (less duplicate in the returned integer) than the rand()
function.
The rand()
function allows you to reverse the $min
and $max
arguments.
When you pass a $max
number that is less than the $min
number, the function will work the same:
echo rand(10, 5); // random 5 to 10
echo mt_rand(10, 5); // Uncaught ValueError
Calling mt_rand()
with a lesser $max
value will return false
and throw the following warning:
Warning: Uncaught ValueError: mt_rand():
Argument #2 ($max) must be greater than or
equal to argument #1 ($min) in php shell code:1
When you’re using PHP v7.1 and above, the rand()
function is backward compatible.
The function will not return false
when the $max
number is lesser than $min
.
And that’s how you generate a random number using PHP 👍