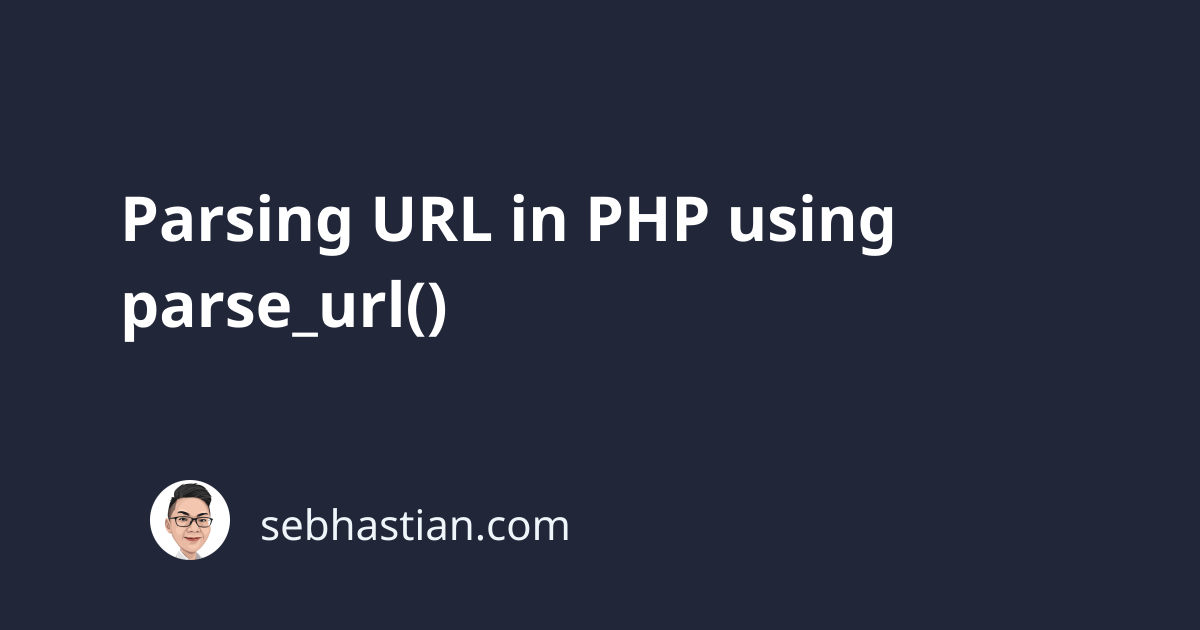
To parse a URL with PHP, you need to use the built-in parse_url()
function.
The parse_url()
function takes a URL string
and returns an associative array containing the URL’s components.
The syntax of the function is as follows:
parse_url(
string $url,
int $component = -1
): int|string|array|null|false
The parse_url()
function accepts two parameters:
- The required
$url
string to parse - The
$component
integer tells the function to retrieve a specific URL component (optional)
Here’s an example of calling the parse_url()
function:
<?php
$url = parse_url("https://sebhastian.com/php-parse-url");
print_r($url);
// 👇 output
// Array
// (
// [scheme] => https
// [host] => sebhastian.com
// [path] => /php-parse-url
// )
The above code shows how parse_url()
returns an associative array of three elements: the scheme
, host
, and path
elements.
Depending on your URL form, the function can return the elements below:
scheme
host
port
user
pass
path
query
fragment
You can select a specific component as follows:
PHP_URL_SCHEME
PHP_URL_HOST
PHP_URL_PORT
PHP_URL_USER
PHP_URL_PASS
PHP_URL_PATH
PHP_URL_QUERY
PHP_URL_FRAGMENT
Here’s an example of retrieving only the URL’s host:
<?php
echo parse_url(
"https://sebhastian.com/php-parse-url",
PHP_URL_HOST
);
// 👇 output:
// sebhastian.com -> string
The component you wish to retrieve will be returned as a string
except for PHP_URL_PORT
which will return an int
.
When the component doesn’t exist, the function returns null
.
For more information and examples, you can visit the parse_url
documentation.
Now you’ve learned how to parse a URL using PHP. Good work! 👍