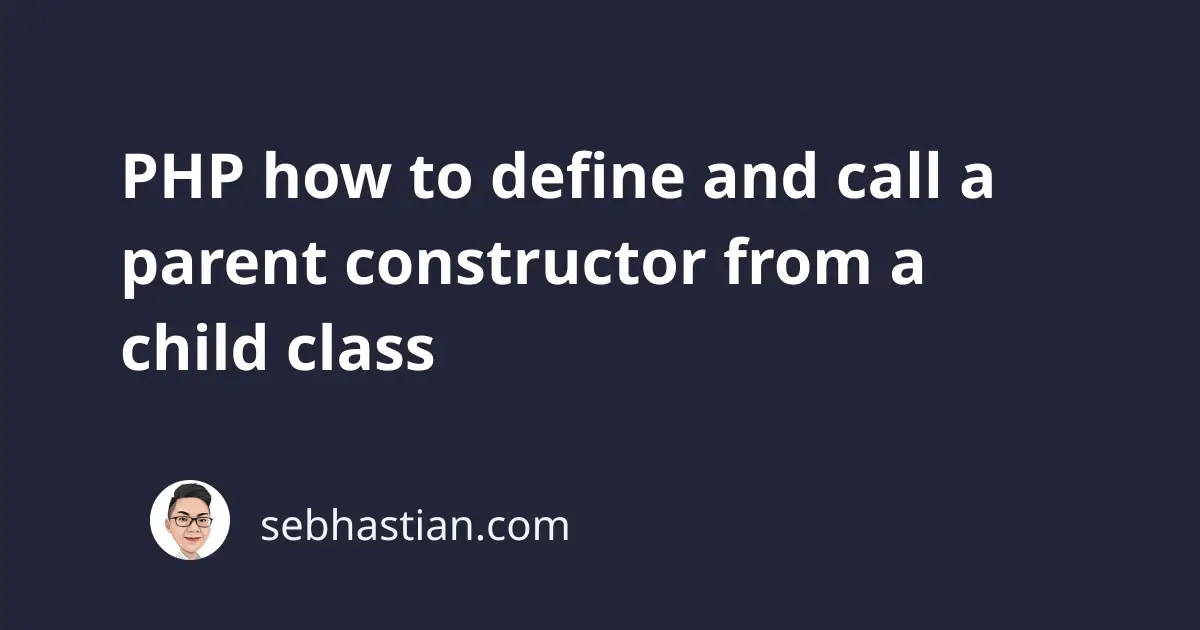
PHP inheritance features allow you to define a constructor method in a parent class.
The constructor can then be called from the child class with the parent::__construct(arguments)
syntax.
When the child class doesn’t have a constructor, the parent constructor will be called automatically.
For example, suppose you have a Vehicle
class as shown below:
class Vehicle {
function __construct() {
print "Constructing a vehicle";
}
}
Suppose you have a Car
class that extends the Vehicle
class as shown below:
class Car extends Vehicle {
function start() {
print "Starting the car";
}
}
When you create a Car
object, the Vehicle
constructor gets called automatically:
$car = new Car();
// 👆 prints: Constructing a vehicle
But if the child class has a constructor, then the parent constructor won’t be called automatically:
<?php
class Vehicle {
function __construct() {
print "Constructing a vehicle";
}
}
class Car extends Vehicle {
function __construct() {
print "Constructing a car";
}
}
$car = new Car();
// 👆 Constructing a car
To call the parent constructor in a child constructor, you need to use parent::__construct(arguments)
syntax.
See the example below:
class Vehicle {
function __construct() {
print "Constructing a vehicle";
}
}
class Car extends Vehicle {
function __construct() {
parent::__construct();
print "\nConstructing a car";
}
}
$car = new Car();
The output of the code above will be:
Constructing a vehicle
Constructing a car
As you can see, now the parent Vehicle
constructor gets called from the child Car
constructor.
When the constructor method requires argument(s), then you need to pass them when initializing the object or calling the parent constructor manually.
Now you’ve learned how to define and call a parent constructor in a child constructor using PHP. Nice work! 👍