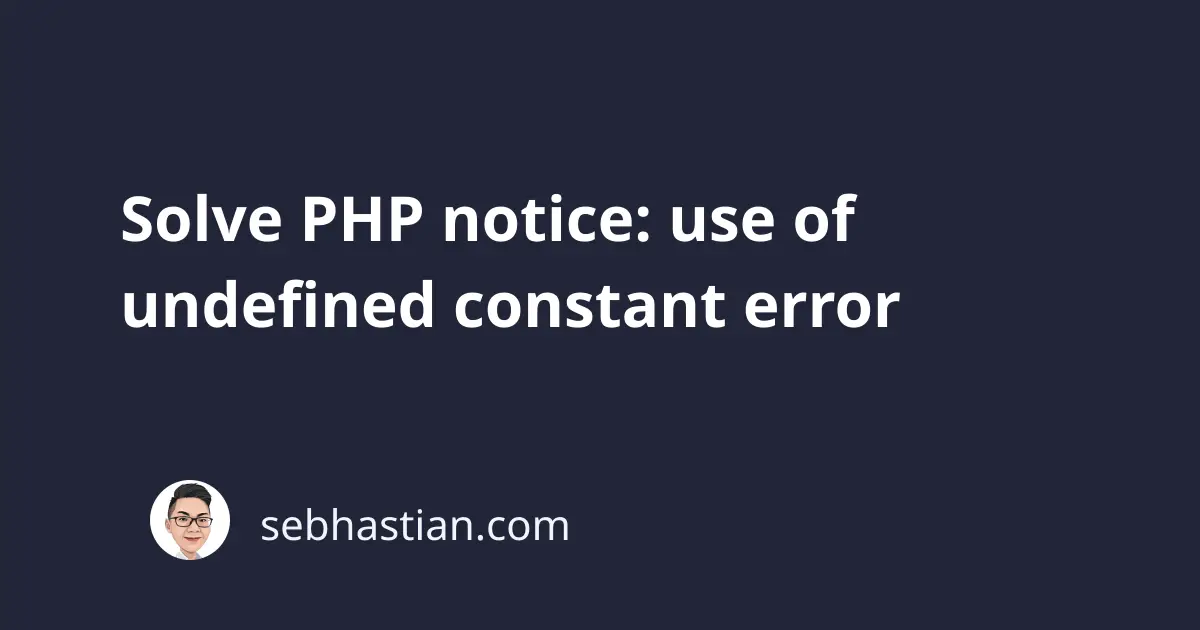
The message PHP notice: use of undefined constant
means that you are calling a constant that has not been defined in your code.
There are three known causes of this notice:
Let’s see how you can solve the error causes in this tutorial.
Calling an undefined constant
In PHP, a constant is a variable that’s created using the const
keyword or define()
function.
Here’s an example of defining constants:
<?php
// 👇 define constant #1
define ('MAX', 9);
// 👇 define constant #2
const MIN = 1;
// 👇 print the constants
print MAX . "\n"; // 9
print MIN; // 1
To solve the PHP notice: use of undefined constant
warning message, you need to check your code and make sure the constant has been defined either using define()
or const
as shown above.
When you have the constant defined, the warning should disappear.
Leaving out the $ symbol when calling variables
The error also appears when you call a variable without adding the $
symbol:
<?php
$name = "Nathan";
// 👇 Use of undefined constant name
print name;
To solve the warning, check your code and add the $
symbol when you meant to call a variable.
Leaving out the quotes around strings
The use of undefined constant warning also appears when you forget to add quotes around a string.
This usually occurs when calling an array value using a string as its key.
Consider the example below:
<?php
// 👇 create an array
$person = [
"name" => "Nathan",
"age" => 29
];
// 👇 Use of undefined constant name
print $person[name];
In the above code, the call to $person[name]
causes the warning because there’s no string around the name
key.
Add a single or double quote around the key, and the warning will disappear.
Now you’ve learned how to resolve the PHP notice: use of undefined constant message.
A constant has to be defined before you can call it. If it’s not a constant, then you need to add a $
symbol when calling a variable or put quotes when calling a string.
I hope this tutorial has been useful for you. 🙏