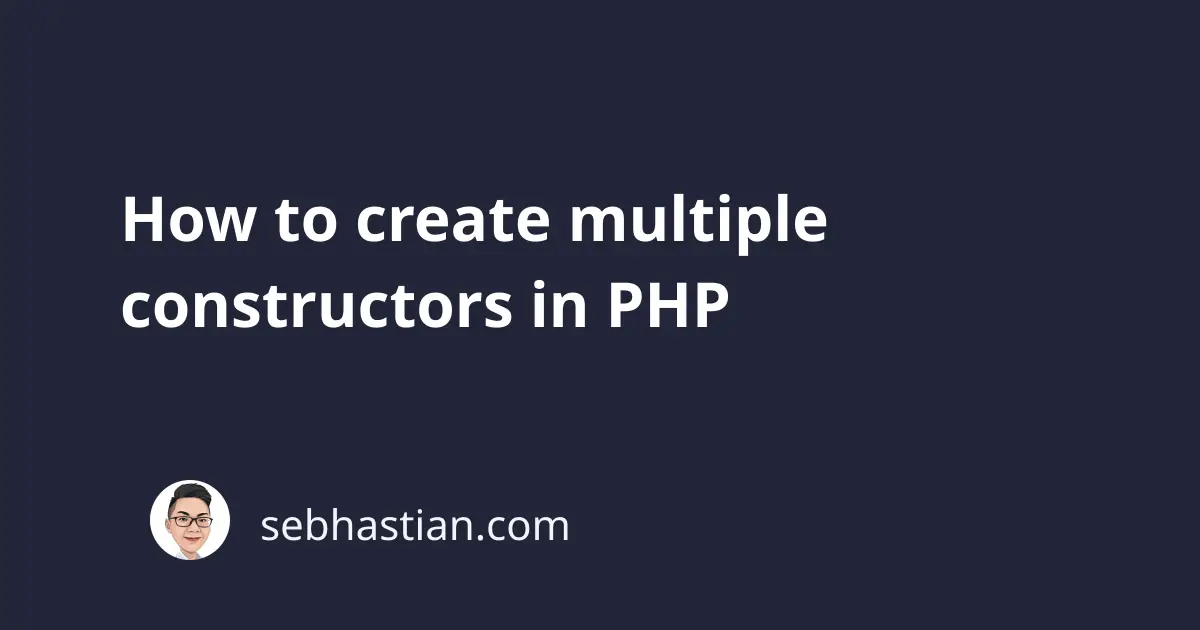
A constructor is a function defined in a class that gets called automatically when you initialize an object of that class.
The constructors are commonly used to initialize properties of an object to make it usable.
In PHP, a constructor is defined using the __construct()
name like this:
<?php
class Person {
public string $name;
// 👇 initialize with name property
public function __construct(string $name) {
$this->name = $name;
}
}
$user = new Person("Nathan");
print $user->name; // Nathan
In the example above, the __construct()
function of the Person
class gets called when you initialize an object of that class.
The arguments you passed to the new Person()
then get passed to the __construct()
function.
How to declare multiple constructors in a class?
PHP doesn’t allow you to create multiple constructors in a single class. It will throw an error if you try:
class Person {
public string $name;
public int $age;
public function __construct(string $name) {
$this->name = $name;
}
// 👇 Fatal error: Cannot redeclare Person::__construct()
public function __construct(string $name, int $age) {
$this->age = $age;
}
}
To declare multiple constructors in PHP, you need to create a workaround that simulates the way constructors work.
There are three ways you can simulate multiple constructors in PHP:
Let’s see how to do them in this tutorial.
Use optional parameters in the constructor
To simulate multiple constructors in PHP, you can define the parameters in the constructor function as optional.
Consider the following example:
class Person {
public string $name;
public int $age;
// 👇 make $age optional
public function __construct(string $name, int $age = null) {
$this->name = $name;
$this->age = $age;
}
}
The $age
parameter in the example above has the default value of null
.
This is like having two constructors where you are free to pass one or two parameters.
Use static helper methods in the class
This workaround solution uses static
functions to simulate constructors.
You need to create a new instance of the class inside the static
functions as shown below:
<?php
class Person {
public string $name;
public int $age;
public string $gender;
public function __construct($name) {
$this->name = $name;
}
public static function __construct2($name, $age) {
$instance = new self($name);
$instance->age = $age;
return $instance;
}
public static function __construct3($name, $age, $gender) {
$instance = new self($name);
$instance->age = $age;
$instance->gender = $gender;
return $instance;
}
}
After you created the class, you can create new instances of the class like this:
$p = new Person("Nathan");
$p = Person::__construct2("Nathan", 29);
$p = Person::__construct3("Nathan", 29, "Male");
When you have more than one parameter, you need to call the static functions to initialize the object.
The constructor gets called with the new self()
function, then you assign the parameters as properties to the instance.
Next, let’s see how you can use the call_user_func_array()
function to really simulate multiple constructors.
Use call_user_func_array() to simulate multiple constructors
The call_user_func_array()
is used to dynamically call a function.
This function is useful when you don’t know the function’s name before running the code.
Together with func_get_args()
, func_num_args()
, and method_exists()
functions, you can simulate multiple constructors in PHP.
Let’s see an example. First, you create multiple construct
functions with the number of arguments added to the function name as shown below:
class Person {
public function __construct1($name) {
print "__construct with 1 param called: " . $name . PHP_EOL;
}
public function __construct2($name, $age) {
print "__construct with 2 params called: " .
$name .
"," .
$age .
PHP_EOL;
}
public function __construct3($name, $age, $gender) {
print "__construct with 3 params called: " .
$name .
"," .
$age .
"," .
$gender .
PHP_EOL;
}
}
The functions above will act as multiple constructors of the Person
class.
Next, create the __construct()
function that will be called when the class gets initialized.
You need to get the arguments and the number of arguments first:
class Person {
public function __construct() {
$arguments = func_get_args();
$numberOfArguments = func_num_args();
}
}
Next, you call the method_exists()
function to see if a construct
method exists with that number of arguments.
When the method exists, call the method using call_user_func_array()
:
class Person {
public function __construct() {
$arguments = func_get_args();
$numberOfArguments = func_num_args();
$constructor = method_exists(
$this,
$fn = "__construct" . $numberOfArguments
);
if ($constructor) {
call_user_func_array([$this, $fn], $arguments);
} else {
print "No matching constructor found";
}
}
// ... code omitted for clarity
}
Now you can initialize the class object with multiple parameters, and it will call the corresponding constructor.
Here’s the complete code for simulating multiple constructors in PHP:
<?php
class Person {
public function __construct() {
$arguments = func_get_args();
$numberOfArguments = func_num_args();
$constructor = method_exists(
$this,
$fn = "__construct" . $numberOfArguments
);
if ($constructor) {
call_user_func_array([$this, $fn], $arguments);
} else {
print "No matching constructor found" . PHP_EOL;
}
}
public function __construct1($name) {
print "__construct with 1 param called: " . $name . PHP_EOL;
}
public function __construct2($name, $age) {
print "__construct with 2 params called: " .
$name .
"," .
$age .
PHP_EOL;
}
public function __construct3($name, $age, $gender) {
print "__construct with 3 params called: " .
$name .
"," .
$age .
"," .
$gender .
PHP_EOL;
}
}
$p = new Person("Nathan");
$p = new Person("Nathan", 29);
$p = new Person("Nathan", 29, "Male");
$p = new Person("Nathan", 29, "Male", "Tester");
This solution is the closest to multiple constructors that you can get in PHP.
It’s also the most complex solution, so you need to choose the right solution for your situation.
Feel free to use the solution you liked best. 😉