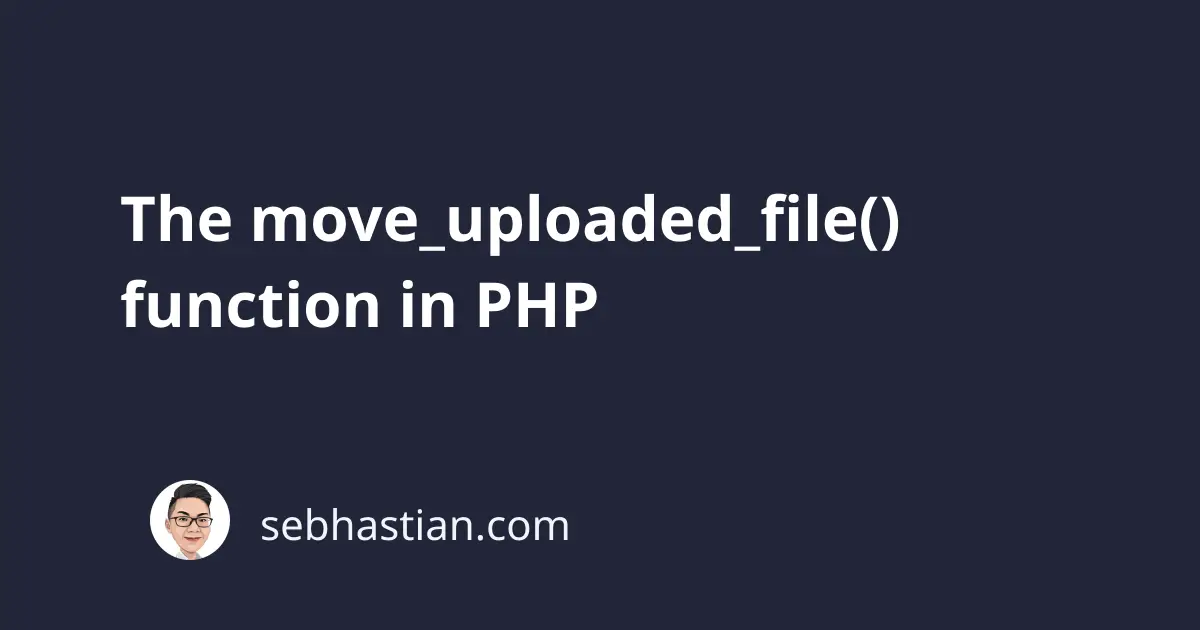
The PHP move_uploaded_file()
function is used to move an uploaded file to a new location.
This function has the following syntax:
move_uploaded_file(string $from, string $destination): bool
The function parameters are as follows:
- The
$from
string is the filename of the uploaded file (required) - The
$destination
string is the path for the new location (required)
The move_uploaded_file()
function returns true
when the file is successfully moved.
When the file is invalid or can’t be moved, the function returns false
.
When you upload a file using a form, PHP will store that file under the $_FILES
global variable.
Suppose you have an HTML form as follows:
<form action="upload.php"
method="POST"
enctype="multipart/form-data">
<input type="file" name="uploaded_file" />
<input type="submit" value="Submit Form" />
</form>
When you upload a file and click submit, then the file will be available under the $_FILES["uploaded_file"]
.
To move the file, you need to access the tmp_name
key from the $_FILES
array as shown below:
if (isset($_FILES['uploaded_file']))
{
move_uploaded_file(
$_FILES['uploaded_file']['tmp_name'],
"/Users/Uploads/"
);
} else {
echo "uploaded_file is empty";
}
The file will be moved to the /Users/Uploads/
folder in the example above.
You can also specify the name of the file on the new location like this:
// 👇 hard-code the file name as my_file.pdf
move_uploaded_file(
$_FILES['uploaded_file']['tmp_name'],
"/Users/Uploads/my_file.pdf"
);
// 👇 use the name element from the $_FILES array
move_uploaded_file(
$_FILES['uploaded_file']['tmp_name'],
"/Users/Uploads/$_FILES['uploaded_file']['name']"
);
I have some tips to help you work with this function:
Keep in mind that if a file with the same name already exists in the destination, then PHP will overwrite the file.
move_uploaded_file()
only works with a file uploaded using the HTTP POST request, so you can’t use it to move files in your server or system.Also, the
$destination
folder must already exist in your system because the function won’t create it for you.Finally, it’s recommended that you check if the file you want to move is available in the
$_FILES
variable using theisset()
function:
if (isset($_FILES['uploaded_file']))
{
// call move_uploaded_file here...
}
And now you’ve learned how the move_uploaded_file()
function works in PHP. Good work! 👍