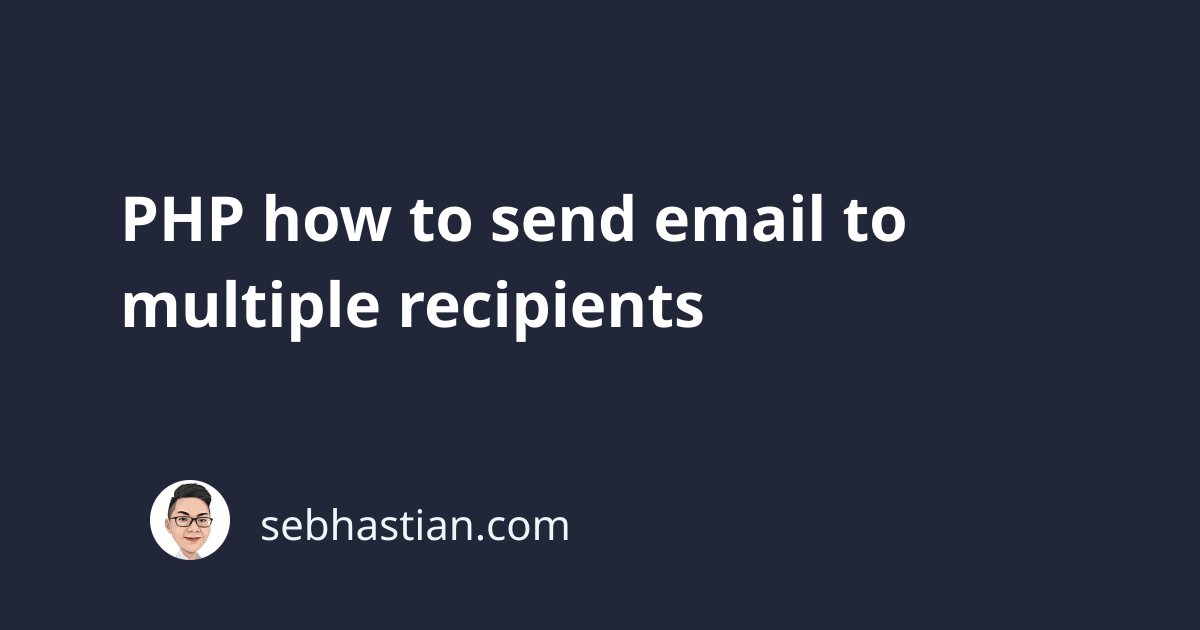
To send an email to multiple recipients in PHP, you need to specify the email addresses as a comma-separated value in the mail()
function.
The PHP mail()
function is used to send emails. The first parameter of the function is where you specify the recipients of your email:
// mail() parameters
mail(
string $to,
string $subject,
string $message,
array|string $additional_headers = [],
string $additional_params = ""
): bool
Although the $to
parameter accepts only a string, you can pass a comma-separated value of email addresses as shown below:
// π specify multiple recipients
$to = "[email protected], [email protected]"
$subject = "Hello World!";
$message = "The weather is great today.";
// π send a mail
mail($to, $subject, $message);
The example above will send the mail to two recipients. You can add as many recipients as you need.
Sometimes, you have the email addresses stored in a database column.
To get the emails from the database, you need to use a loop and put all email addresses in an array.
After that, you can use implode()
to join the array elements as a string:
// π fetch rows from the database
$rows = mysql_fetch_array($result);
// π loop over the array and get the mail addresses
while ($rows) {
$recipients[] = $rows["email"];
}
// π use implode to create comma-separated string
$to = implode(", ", $recipients);
// π send the email
$subject = "Hello World!";
$message = "The weather is great today.";
mail($to, $subject, $message);
The same solution applies when you already have the recipients as an array:
$recipients = ["[email protected]", "[email protected]"];
// π use implode to create comma-separated string
$to = implode(", ", $recipients);
// π send the email
$subject = "Hello World!";
$message = "The weather is great today.";
mail($to, $subject, $message);
And thatβs how you send an email to multiple recipients using PHP.
If you want to send the mail to multiple Ccs or Bccs, you need to add the recipients in the $additional_headers
parameter.
See the example below:
$to = "[email protected]";
$subject = "Hello World!";
$message = "The weather is great today.";
// π get the ccs as a string
$ccs = ["[email protected]", "[email protected]"];
$cc = implode(", ", $ccs);
// π add ccs and bccs here
$header = [
"Cc" => $cc,
"Bcc" => "[email protected], [email protected]",
];
// π send the mail
mail($to, $subject, $message, $header);
As you can see, sending PHP mail()
to multiple recipients can be done easily.
You can also add Ccs and Bccs like to the function.