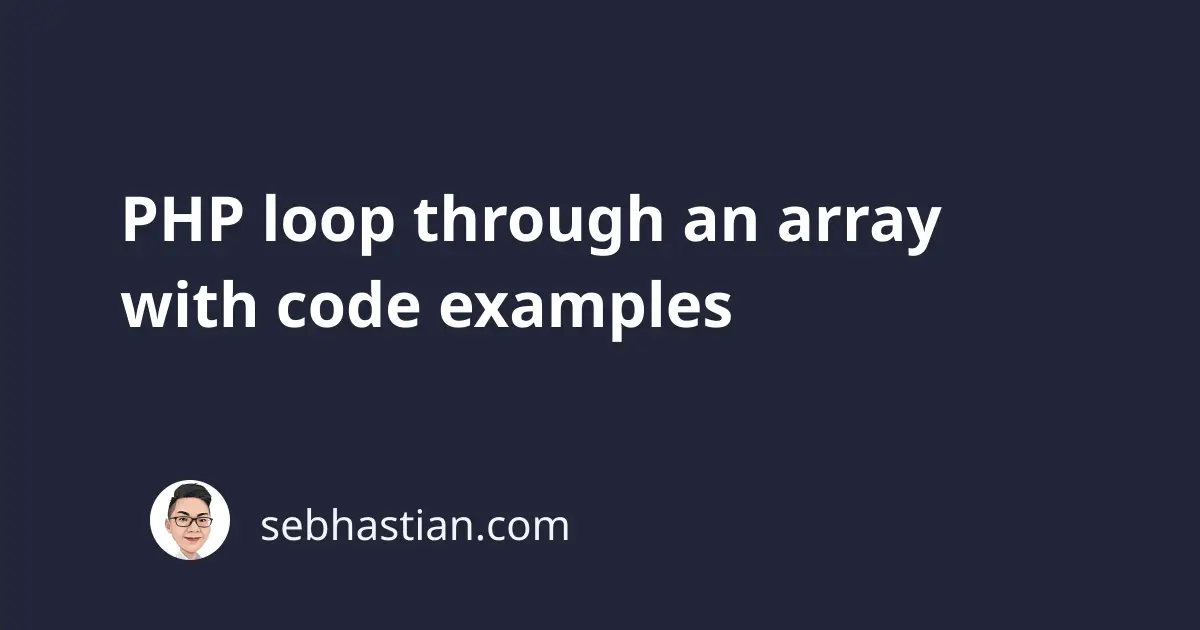
To loop through an array in PHP, you need to use the foreach()
construct.
Loop through regular and associative arrays in PHP
The foreach()
construct can be used to loop through an array as shown below:
$arr = [1, 2, 3];
foreach ($arr as $val) {
echo "{$val} ";
}
// 👇 Output:
// 1 2 3
When you have an associative array, you can also grab the keys inside the foreach()
construct.
Note the difference inside the foreach()
construct below:
$arr = [
"Name" => "Nathan",
"Age" => 28,
"Role" => "PHP Developer"
];
foreach ($arr as $key => $val) {
echo "{$key} : {$val} \n";
}
// 👇 Output:
// Name : Nathan
// Age : 28
// Role : PHP Developer
You can also use the colon symbol :
to open the foreach
block, then close it using the endforeach;
construct.
Some prefer this syntax as it’s more readable and clean as shown below:
<?php
$arr = [
"Name" => "Nathan",
"Age" => 28,
"Role" => "PHP Developer"
];
foreach ($arr as $key => $val):
echo "{$key} : {$val} \n";
endforeach;
Loop through a multi-dimensional array in PHP
The foreach
construct can also be used to loop through a multi-dimensional array in PHP.
But to do so, you need to extract the multi-dimensional array using the list
construct.
The list
construct will assign each element in your array as a variable.
Here’s an example of unpacking a two-dimensional array using foreach
:
$users = [
["Nathan", 28],
["Jane", 22],
["John", 32],
];
foreach ($users as list($name, $age)):
echo "{$name} : {$age} \n";
endforeach;
// 👇 Output:
// Nathan : 28
// Jane : 22
// John : 32
The variables assigned using the list
construct can then be used inside the foreach
body as shown above.
If you’re using PHP version 7.1 or above, you can also replace the list()
syntax with the array brackets notation []
like this:
$users = [
["Nathan", 28],
["Jane", 22],
["John", 32],
];
foreach ($users as [$name, $age]):
echo "{$name} : {$age} \n";
endforeach;
And that’s how you loop through a two-dimensional array using foreach
and list
constructs. 👍