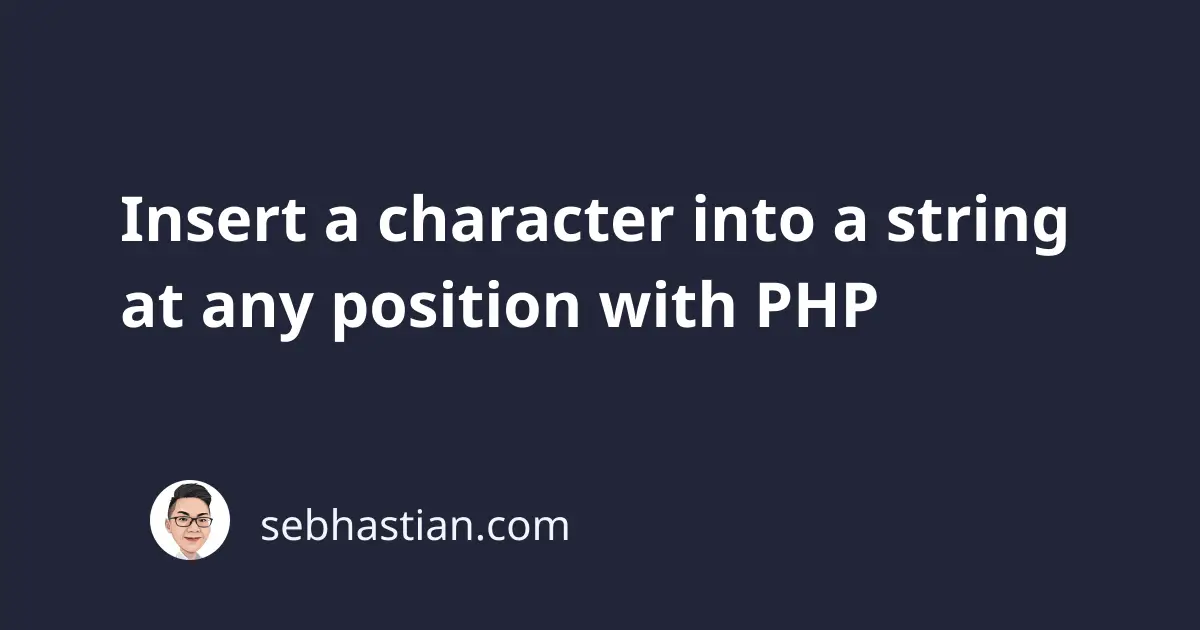
To insert a character into another string using PHP, you need to use the substr_replace()
function.
The substr_replace()
function is used to replace a portion of a string.
The function accepts 4 arguments as shown below:
substr_replace(
array|string $string,
array|string $replace,
array|int $offset,
array|int|null $length = null
): string|array
To insert characters without replacing the original string, you need to pass 0
as the $length
argument of this function.
Let’s see an example. Suppose you need to insert the word very
into the string I am feeling lucky today
.
Here’s how you do it using substr_replace()
:
<?php
$str = "I am feeling lucky today";
$new_str = substr_replace($str, "very ", 13, 0);
print $new_str; // I am feeling very lucky today
To insert characters into a string, you need to count the index where you want to insert the string. A string index starts from the left at 0
.
The word very
needs to be placed before the word lucky
.
The index of l
in lucky
is 13, so that’s what you need to pass as the $offset
argument.
You also need to pass 0
as the $length
argument, because the function will remove the rest of the string if you don’t pass any $length
value:
<?php
$str = "I am feeling lucky today";
$new_str = substr_replace($str, "very ", 13);
print $new_str; // I am feeling very
You can pass a single character or a string as the $replace()
argument to the substr_replace()
function.
Make sure that you pass the right $offset
argument, or you will have a wrong string.
Now you’ve learned how to insert characters into a string at any position with PHP. Great job!