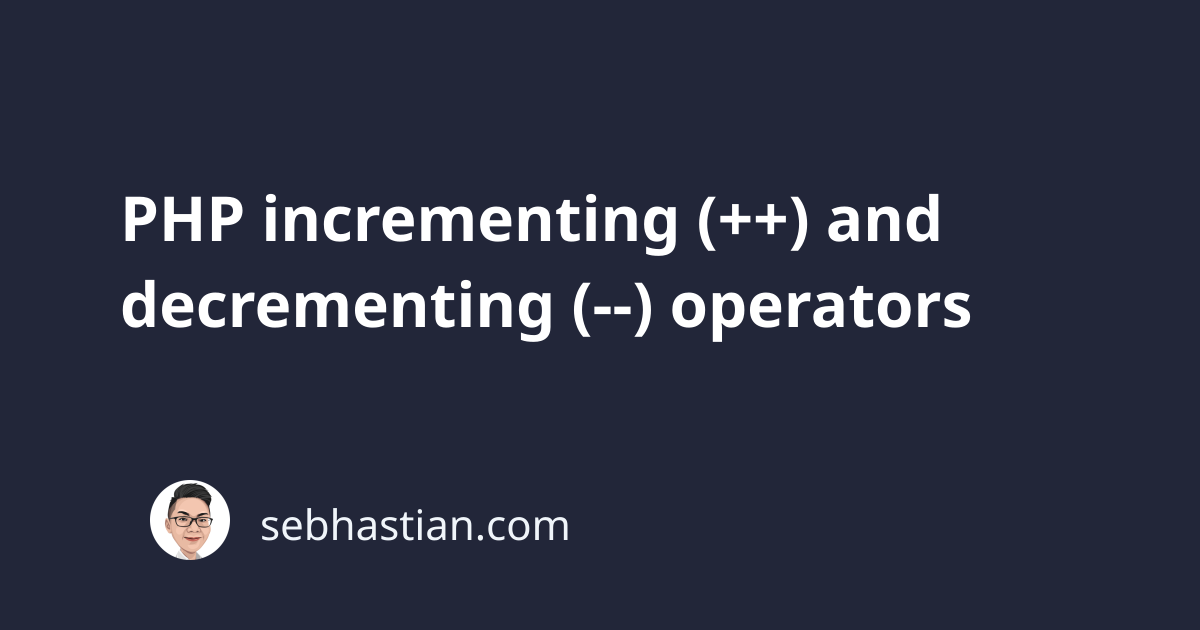
The PHP incrementing (++
) and decrementing (--
) operators are used to increment or decrement a variable value by one.
To use the operator, you add the symbol before or after the variable as shown below:
// π incrementing operator
$a = 7;
++$a;
echo $a; // 8
// π decrementing operator
$b = 7;
--$b;
echo $b; // 6
As you can see, you only need to add the ++
or --
operator before the variable.
Whatβs the difference between ++var and var++ in PHP?
PHPβs incrementing and decrementing operators can be added before or after the variable name.
Adding the operator before the variable is called pre-incrementing.
Adding the operator after the variable is called post-incrementing.
The only difference between the two methods is the timing of the increment/decrement operation.
Consider the following example and notice the difference in the highlighted lines:
// π pre-increment syntax
$a = 7;
echo ++$a; // 8
echo $a; // 8
// π post-increment syntax
$a = 7;
echo $a++; // 7
echo $a; // 8
When you pre-increment a variable, the increment is done before running that increment line.
But when you post-increment a variable, the increment is done after running that increment line.
This is why the pre-increment prints the variable as 8
in the highlighted line, but post-increment prints the variable as 7
.
The same logic applies to decrementing as well:
// π pre-decrement syntax
$a = 7;
echo --$a; // 6
echo $a; // 6
// π post-decrement syntax
$a = 7;
echo $a--; // 7
echo $a; // 6
In most cases, pre-incrementing is preferred over post-incrementing because it performs faster and is more intuitive.
When you increment or decrement a variable, you would expect the variable has been evaluated to the new value when the code line is running.
Finally, the increment or decrement operators only work on numbers and strings. Other types like arrays, booleans, and resources will have no effect.
And now youβve learned how the PHP ++
and --
operators work. Nice work! π