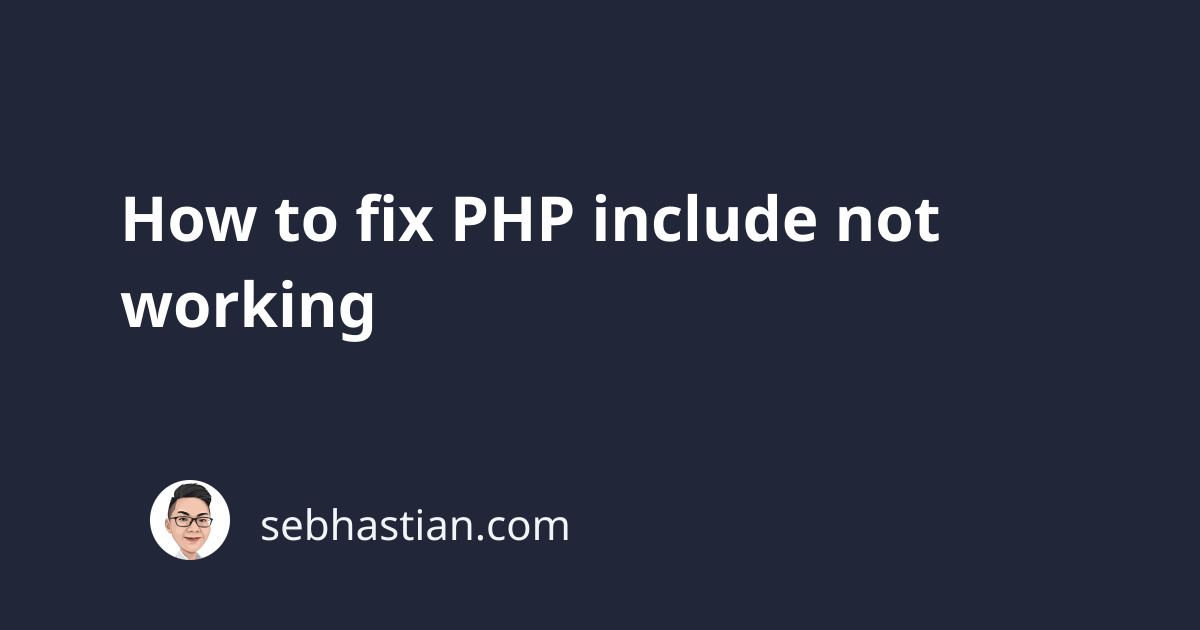
When you add a PHP file to another PHP file using the include
function, you may find that the include
call doesn’t work.
For example, suppose you include a file as follows:
include "functions.php";
PHP may return the following warning on your screen:
Warning: include(functions.php): Failed to open stream:
No such file or directory in ... on line ...
This tutorial will help you to debug and solve the problem above.
Here are some things you can do to get the include
statement working:
Make sure the file exists
This should go without saying, but sometimes you may have a typo in the file name you specify.
For example, you may write functions.php
when you actually have a function.php
file.
Before you check anything else, make sure that the file you are referencing exists.
Make sure you use the correct path
The include
function will try to import your PHP file by navigating the file system path.
This means that you need to make sure that the included script can be accessed from the script that needs it using the specified path.
For example, suppose you have a project structured as follows:
├── main
│ ├── index.php
│ └── lib
│ └── functions.php
└── template
└── header.php
Say you need to include the functions.php
file in the index.php
file.
You need to specify the path to the lib/
folder from index.php
as shown below:
// index.php
include "lib/functions.php";
The same applies when you have a script located above the script where you include
it in the file system.
Suppose you need to include the template/
folder script in index.php
. Here’s how you do it:
include "../template/header.php";
Because the template/
folder is on the same level as the main/
folder, you need to add ../
to move one level up in the include
statement.
Let’s see another example. Now you need to include the header.php
in the functions.php
file.
Here’s how you do it:
include "../../template/header.php";
But note that if you also include functions.php
inside index.php
, then PHP will try to get to header.php
from index.php
location.
To handle this kind of pathing error, you need to add $_SERVER["DOCUMENT_ROOT"]
to the include path.
This way, you will reference the included file from the root of your PHP project
// index.php
include $_SERVER["DOCUMENT_ROOT"] . "/main/lib/functions.php";
// functions.php
include $_SERVER["DOCUMENT_ROOT"] . "/template/header.php";
The include path is not dynamic and won’t adjust based on where you call the files from.
To avoid pathing errors, add $_SERVER["DOCUMENT_ROOT"]
and specify the absolute path instead of the dynamic path.
Make sure you use the system file path and not URL path
When you define the file path in the include
statement, you need to use the file path instead of the URL path.
You can’t do the following:
include "http://localhost:8000/template/header.php";
As it will cause the warning below:
Warning: include(http://localhost:8000/template/header.php):
Failed to open stream:
no suitable wrapper could be found in ... on line ...
If you want to include a URL path as shown above, you need to activate the allow_url_include
option in your php.ini
file:
# 👇 allow URL include
allow_url_include = On
But this is not recommended because your PHP application will be open to exploitation by malicious hackers.
Remote URL for include
statement introduces vulnerability to your application.
Change include with require to find the cause
When there’s an issue with the include
function, PHP will continue running your script and only emits a warning.
This makes it hard to debug and find why the include
function doesn’t work.
To find the cause of the issue, you need to change include
with require
.
Unlike include
, the require
function will stop the script execution and emits a compile error.
// 👇 change include with require
require "functions.php";
By using a require
statement, PHP will stop running your script when the file import encounters any issue.
And those are the four things you can do to solve the PHP include
not working issue.
I hope this tutorial has been useful for you. 🙏