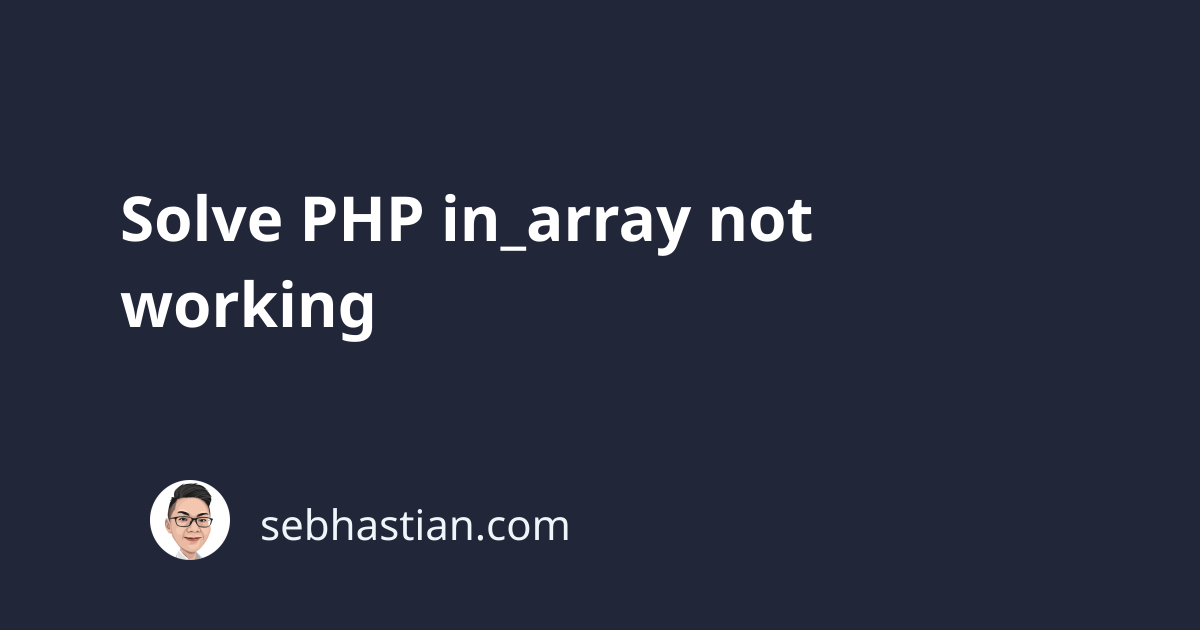
Sometimes, the PHP in_array()
function may return a different result than what you expected.
Depending on how you use the function, here are some common issues that cause the in_array()
function to not work:
This tutorial will help you make the in_array()
function works as expected.
Search for multiple values from the array
The first parameter of the in_array()
function is the value you want to search in the array.
The search parameter can only search for a single value, so you can’t search for multiple values by using an array.
Consider the following code example:
$animals = [
"Lion",
"Tiger",
"Horse",
"Bird"
];
// 👇 search for a Lion
var_dump(in_array("Lion", $animals)); // true
var_dump(in_array("Tiger", $animals)); // true
Suppose you want to in_array()
to return true when you have a Lion
or a Tiger
in the array.
You can’t use an array as the search parameter:
// 👇 search for a Lion or Tiger
var_dump(in_array(["Lion", "Tiger"], $animals)); // false
Because ["Lion", "Tiger"]
will cause the in_array()
function to look for an array instead of the values.
To search for two values using in_array()
, you need to call the function twice and join them with the &&
or ||
operator as follows:
// 👇 search for a Lion OR Fish
var_dump(
in_array("Lion", $animals) || in_array("Fish", $animals)
); // true
// 👇 search for Lion AND Fish
var_dump(
in_array("Lion", $animals) && in_array("Fish", $animals)
); // false
When you need all values present in the array, use the &&
operator.
When you need only one of the values to be present, use the ||
operator.
Only use an array when you’re looking for an array as shown below:
$animals = [
"Lion",
"Tiger",
"Horse",
"Bird",
["Fish", "Squid"]
];
// 👇 search for a literal array
var_dump(in_array(["Fish", "Squid"], $animals)); // true
The in_array()
function will work when you put the right value as its parameter.
The string type is case-sensitive
When you’re searching for a string using the in_array()
function, you need to keep in mind that the search will be case-sensitive.
This means that you need watch the string case carefully:
$animals = ["Lion", "Tiger", "Horse"];
// 👇 case match, returns true
var_dump(in_array("Lion", $animals)); // true
// 👇 case doesn't match, returns false
var_dump(in_array("LION", $animals)); // false
The value in the array above is Lion
, so putting LION
will cause the function to return false
.
Use the strict mode
The in_array()
function runs in loose mode by default.
When in loose mode, the function will return true
when you search for an integer
type with a string
and vice versa:
$arr = [
785,
"912",
];
// 👇 loose mode returns true
var_dump(in_array("785", $arr)); // true
var_dump(in_array(912, $arr)); // true
To make the function performs a strict check, you need to pass true
as its third parameter like this:
$arr = [
785,
"912",
];
// 👇 strict mode returns false
var_dump(in_array("785", $arr, true)); // false
var_dump(in_array(912, $arr, true)); // false
When you enable the strict mode, PHP immediately returns false
when the value you search for is not present in the array.
This may help in certain cases when PHP returns true
but you expect false
and vice versa.
Searching on multi-dimensional arrays
The in_array()
function doesn’t work on multi-dimensional arrays by default.
You need to create a custom function that uses the in_array()
function to make it work on multi-dimensional arrays.
The following function should be enough to help you:
function in_array_multi(
mixed $needle,
array $haystack,
bool $strict = false)
{
foreach ($haystack as $i) {
if (
($strict ? $i === $needle : $i == $needle) ||
(is_array($i) && in_array_multi($needle, $i, $strict))
) {
return true;
}
}
return false;
}
The in_array_multi()
function above will search for the $needle
value from the $haystack
array you passed as its parameter.
This function also has an extra is_array()
check. It will call the in_array_multi()
function recursively for all the arrays in the $haystack
variable.
Here’s an example of running the function:
$multi_arr = [
"plants" => [
"vegetable" => ["carrot", "lettuce"],
"fruit" => ["apple", "orange"],
],
"animals" => [
"flying" => ["bird", "insect"],
"swimming" => ["fish", "squid"],
]
];
var_dump(in_array_multi("fish", $multi_arr)); // true
var_dump(in_array_multi(["bird", "insect"], $multi_arr)); // true
Feel free to use the in_array_multi()
function in your code.
Conclusion
Because an array has many structures, the in_array()
may return a different result than what you expected.
This tutorial has explained the common issues you may have when using the in_array()
function.
By understanding how the in_array()
function works, you may adjust the code so that it works as you expected.