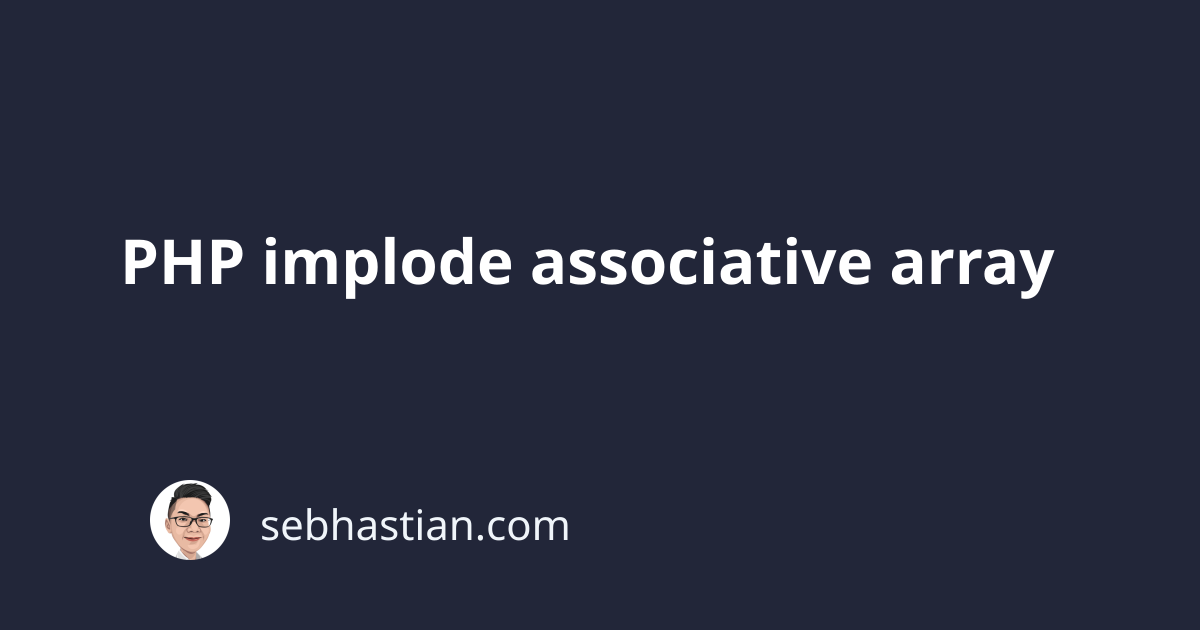
The PHP implode()
function is used to join array values as a string.
This function only takes the array values as the string, so any key you define in an associative array will be ignored.
Consider the following example:
<?php
$user = [
"name" => "Nathan",
"age" => 29,
"hobby" => "reading",
];
print "The values are: " . implode(", ", $user);
The print output will be:
The values are: Nathan, 29, reading
Suppose you need the keys to be included in the output like this:
The values are: name=Nathan, age=29, hobby=reading
Then you can’t use the implode()
function. Instead, you need to use the http_build_query()
to generate a string from the array.
Here’s how you use http_build_query()
to implode an associative array:
<?php
$user = [
"name" => "Nathan",
"age" => 29,
"hobby" => "reading",
];
$string = http_build_query($user, "", ", ");
print $string;
The $string
above will have the following output:
name=Nathan, age=29, hobby=reading
But keep in mind that the http_build_query()
function will encode any special characters found in your string.
Alternatively, you can create a custom function that implodes an array as key-value pairs.
Here’s the function to help you create key-value pairs from an array:
function implode_key_value($separator, $array, $symbol = "=") {
return implode(
$separator,
array_map(
function ($k, $v) use ($symbol) {
return $k . $symbol . $v;
},
array_keys($array),
array_values($array)
)
);
}
The implode_key_value()
function above accept three parameters:
- The
$separator
for the imploded array - The
$array
to implode - The
$symbol
to separate the key-value pair
This function will call the implode()
function on an array processed using the array_map()
function.
The array_map
function above creates a single dimension array from the associative array you passed as its parameter.
Run the following script to test the function:
<?php
function implode_key_value($separator, $array, $symbol = "=") {
return implode(
$separator,
array_map(
function ($k, $v) use ($symbol) {
return $k . $symbol . $v;
},
array_keys($array),
array_values($array)
)
);
}
$user = [
"name" => "Nathan",
"age" => 29,
"hobby" => "reading",
];
print implode_key_value(", ", $user, " => ");
The output will be as follows:
name => Nathan, age => 29, hobby => reading
Now you’ve learned how to implode an associative array in PHP. Nice work!
The easiest way to implode an associative array is to call the http_build_query()
function.
But if you need to format the imploded string or avoid encoding special characters, then you need to create a custom function, such as the implode_key_value()
function shown above.
Feel free to use the function in your project. 👍