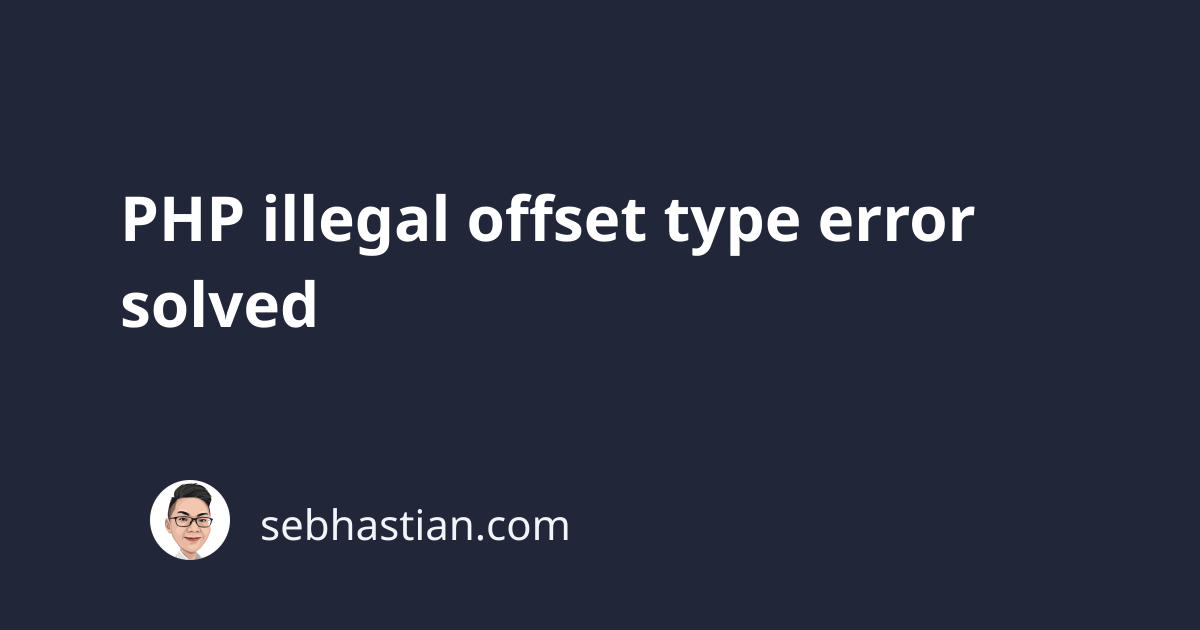
PHP illegal offset type error occurs when you try to access an array using an object or an array as the key.
The PHP array keys are defined using string or int data type as shown below:
<?php
$user[0] = "Nathan";
$user[1] = "Jane";
$user["three"] = "John";
$obj = new stdClass();
print $user[0];
print $user[$obj]; // error
When you try to access an array using $obj
as shown in the example above, PHP will throw the illegal offset type error:
Fatal error: Uncaught TypeError: Illegal offset type
in /home/code.php:9
To resolve this error, you need to make sure that you are accessing the array correctly.
This error also frequently occurs when you are iterating over an array as follows:
<?php
$keys = [0, 1, new StdClass(), 2];
$array = ["Nathan", "John", "Jane", "Mary"];
foreach ($keys as $key) {
print $array[$key] . PHP_EOL; // error
}
Because there is an object in the $keys
array, PHP will throw the illegal offset type error when the iteration reaches that object.
You can use print_r()
or var_dump()
to check on the values of $keys
in the example above:
print_r($keys);
You need to see the values of the $keys
variable and make sure that you are not using an object or an array. The above code will show the following output:
Array
(
[0] => 0
[1] => 1
[2] => stdClass Object
(
)
[3] => 2
)
Once you see the value that causes the error, you need to remove it from your $keys
array.
And that’s how you solve the PHP illegal offset type error.