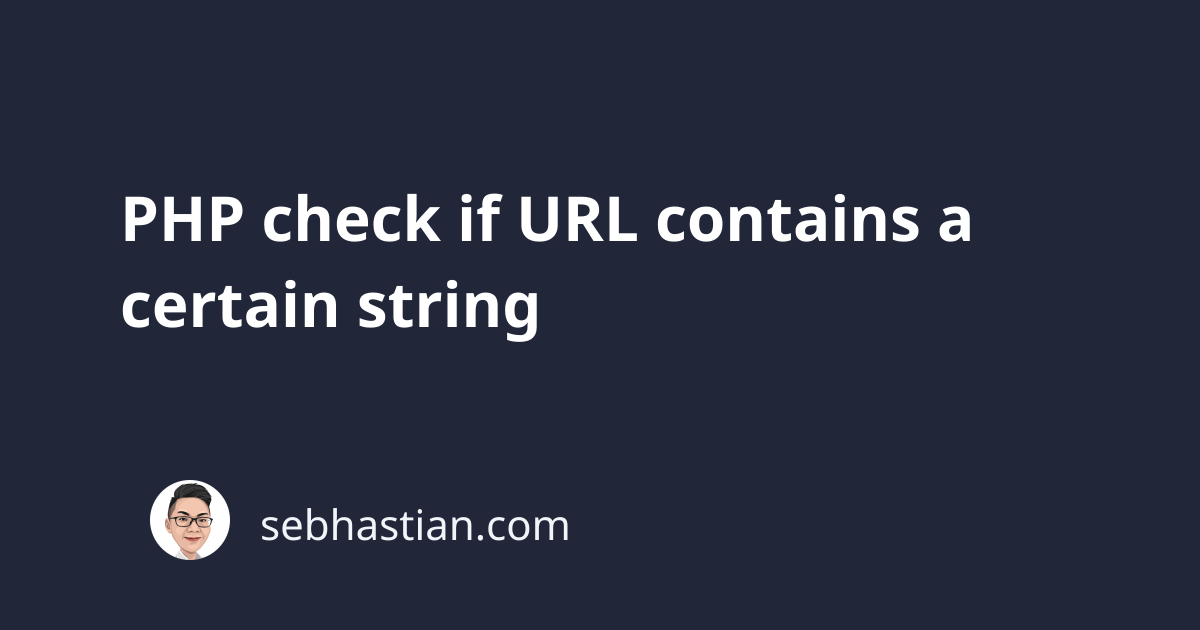
To check if a URL address contains a certain string in PHP, you need to use the strpos()
or str_contains()
function.
When you need to perform an exact match, you need to use a regex with preg_match()
function.
Let’s see how to do both in this tutorial.
Check if URL contains a string in PHP
The strpos()
function helps you check the position of a string portion in a string.
The function returns an int
indicating the position of the string, or false
when the string is not found:
strpos(
string $haystack,
string $needle,
int $offset = 0
): int|false
To check if a certains string exists in a URL address, call the strpos()
as shown below:
$url = "https://sebhastian.com/php";
if (strpos($url, "php")) {
print "php is found in the url";
} else {
print "php is NOT found in the url";
}
In PHP v8, you can also use the str_contains()
function, which checks whether a string contains a certain string.
It doesn’t return the position of the string like strpos()
, only true
if it contains the string or false
otherwise:
str_contains(
string $haystack,
string $needle
): bool
You call the str_contains()
like the strpos()
functions:
$url = "https://sebhastian.com/php";
if (str_contains($url, "php")) {
print "php is found in the url";
} else {
print "php is NOT found in the url";
}
Both str_contains()
and strpos()
will match a URL that has the $needle
anywhere.
For example, php
, php-study
, and phpstudy
are considered valid.
If you want only an exact match, you need another way.
Check if URL contains an exact string in PHP
To check if a URL contains an exact string, you need to call the preg_match()
function.
This function allows you to perform a regex match to your string.
You need to use the \b
word boundary expression in the function as follows:
$url = "https://sebhastian.com/php";
$str = "php";
if (preg_match("~\b$str\b~", $url)) {
print "php is found in the url";
} else {
print "php is NOT found in the url";
}
With the above preg_match()
function, php
and php-study
returns true
while phpstudy
will return false
.
And that’s how you check if URL contains a certain string in PHP.