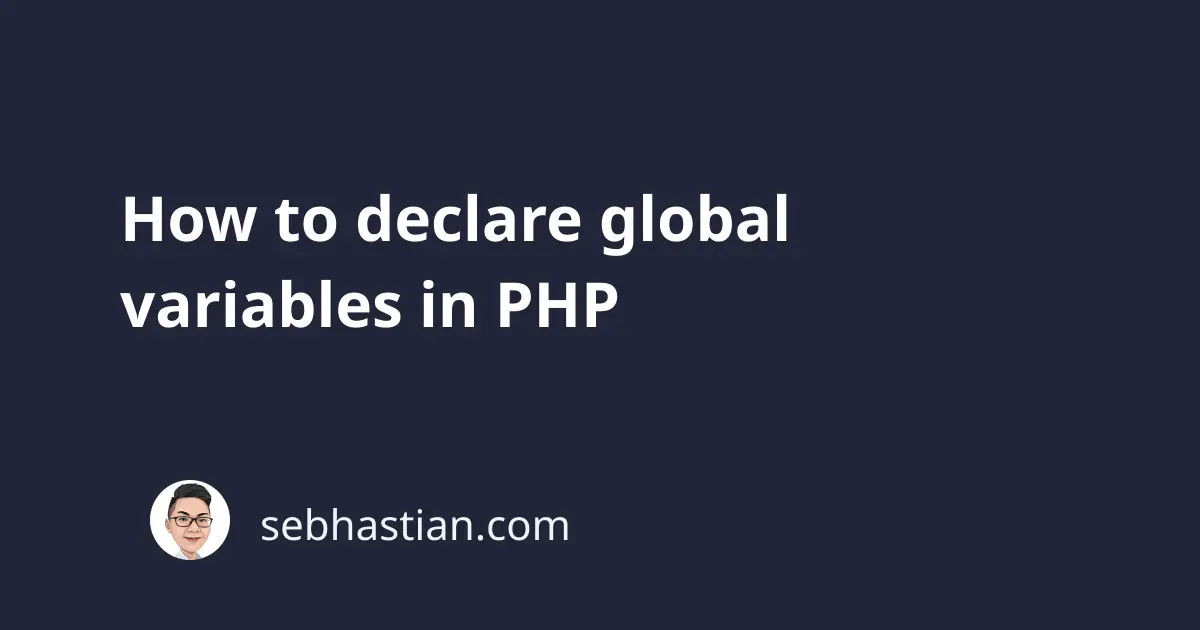
PHP global variables refer to any variables you declared outside of a function.
Here’s an example of declaring a global variable in PHP:
<?php
// 👇 declare global variables in PHP
$first_name = "Nathan";
$last_name = "Sebhastian";
// 👇 concatenate the values
echo "{$first_name} {$last_name}";
PHP global variables are directly accessible from any part of the PHP script as long as it’s outside of a function
.
For example, suppose you split the code above into two PHP files related using the include
keyword:
<?php
// a.php content:
$first_name = "Nathan";
$last_name = "Sebhastian";
include "b.php";
?>
<?php
// b.php content:
echo "{$first_name} {$last_name}";
?>
The b.php
script can still access the $first_name
and $last_name
variables as long as you include
or require
the script as shown above.
Access PHP global variables from a function
Global variables are not directly available inside a PHP function.
When you access a global variable from a function, the undefined variable warning will appear:
$name = "Nathan";
function greetings() {
echo $name; // Warning: Undefined variable $name
}
greetings();
There are two ways you can access PHP global variables from a function:
- Declare the variables using the
global
keyword - Access the
$GLOBALS
array
Let’s learn about these methods next.
Access PHP global variables using the global keyword
The global
keyword is used to reference variables from the global scope inside PHP functions.
Here’s an example of using the global
keyword:
$name = "Nathan";
function greetings() {
global $name;
echo $name;
}
//👇 prints Nathan
greetings();
In the code above, the $name
is declared global inside the function, so the $name
from outside of the function is imported into the function.
The global
keyword points to the global variable without copying the value.
When you change the $name
value inside the function, the actual $name
value will change as well:
$name = "Nathan";
function greetings() {
global $name;
$name = "Joe";
echo $name;
}
//👇 prints Joe
greetings();
//👇 prints Joe as well
echo $name;
Keep this in mind when you’re accessing the variable from many functions, as the variable may have been changed when you access it.
To access global variables from many functions, you can use the global
keyword in each function as follows:
$name = "Nathan";
function nav() {
global $name;
echo $name;
}
function body() {
global $name;
echo $name;
}
function footer() {
global $name;
echo $name;
}
But as you can see, the code becomes quite repetitive.
To avoid having to declare global
in each function, you can use the $GLOBALS
variable instead.
Access PHP global variables using the $GLOBALS array
The $GLOBALS
is an associative array containing references to all global variables available in your PHP script.
You can access the global variables by passing the variable name as the array key like this:
$name = "Nathan";
echo $GLOBALS["name"]; // Nathan
Using the $GLOBALS
array is more convenient when you need to access the global variable from many functions.
You don’t need to refer to the variable using the import
keyword in each function:
$name = "Nathan";
function nav() {
echo $GLOBALS["name"];
}
function body() {
echo $GLOBALS["name"];
}
function footer() {
echo $GLOBALS["name"];
}
When you modify the $GLOBALS
element, the actual variable value will be changed as well:
$name = "Nathan";
$GLOBALS["name"] = "Jack";
echo $name; // Jack
And that’s how you access the global variables using the $GLOBALS
array.
Should you use global variables in PHP?
Global variables can reduce the amount of code you need to write, but it’s also considered bad practice because it’s harder to debug your code when something goes wrong.
When you’re accessing a variable local to the function, you can easily check the function to see if you did something wrong with it.
But if the variable is global, you need to check all the lines where you call that variable and see if the code goes wrong in one of the lines.
To conclude, you are free to use global variables in PHP when it helps. But you also need to consider the difficulty in maintaining and debugging global variables in a complex web application.
I hope this tutorial has been useful for you 🙏