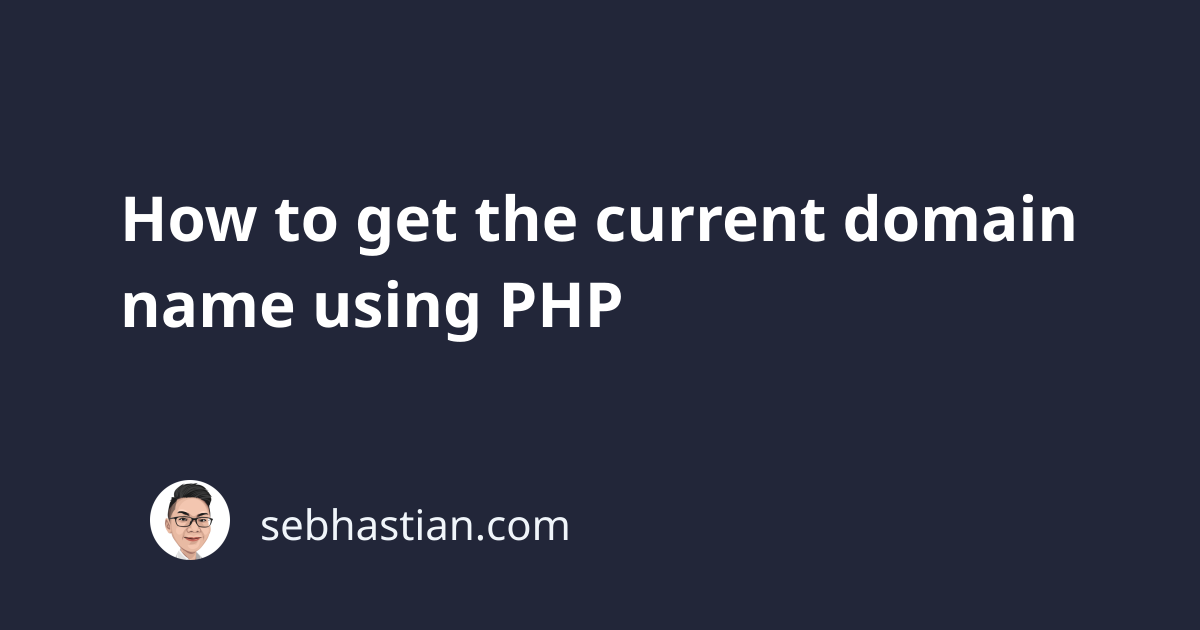
The $_SERVER
array is a global variable that contains server and header information.
To get the domain name where you run PHP code, you need to access the SERVER_NAME
or HTTP_HOST
index from the $_SERVER
array.
Suppose your website has the URL of https://example.com/post/1
. Here’s how you get the domain name:
<?php
print $_SERVER["SERVER_NAME"];
// Output: example.com
If you are using Apache server 2, you need to configure the directive UseCanonicalName
to On
and set the ServerName
.
Otherwise, the ServerName
value reflects the hostname supplied by the client, which can be spoofed.
Aside from the SERVER_NAME
index, you can also get the domain name using HTTP_HOST
like this:
<?php
print $_SERVER["HTTP_HOST"];
// Output: example.com
The difference is that HTTP_HOST
is controlled from the browser, while SERVER_NAME
is controlled from the server.
If you need the domain name for business logic, you should use SERVER_NAME
because it is more secure.
Finally, you can combine SERVER_NAME
with HTTPS
and REQUEST_URI
indices to get your website’s complete URL.
See the code example below:
<?php
// 1. write the http protocol
$full_url = "http://";
// 2. check if your server use HTTPS
if (isset($_SERVER["HTTPS"]) && $_SERVER["HTTPS"] === "on") {
$full_url = "https://";
}
// 3. append domain name
$full_url .= $_SERVER["SERVER_NAME"];
// 4. append request URI
$full_url .= $_SERVER["REQUEST_URI"];
print $full_url;
// example output: https://example.com/post/1
Now you’ve learned how to get the domain name of your website using PHP. Nice!