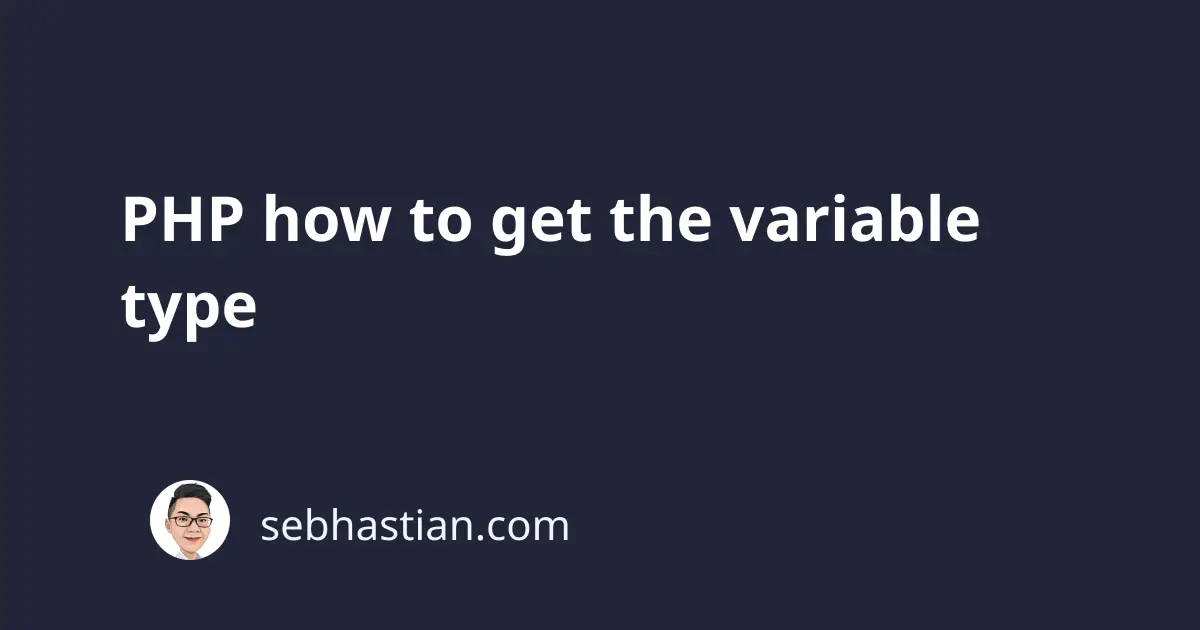
To get the type of variables you have in your code, you can use the PHP gettype()
or var_dump()
function.
The PHP gettype()
function returns a string
that shows the type of the variable you passed as its argument:
$myVar = "Hello";
print gettype($myVar); // string
print gettype(999); // integer
$arr = [1, 2, "A"];
print gettype($arr); // array
The var_dump()
function returns a structured information from an expression.
This var_dump()
can be used to get the type and value that your variable holds.
Unlike gettype()
which returns a string
value, var_dump()
directly produces an output to the browser. You don’t need to add print
or echo
before calling the function:
$myVar = "Hello";
var_dump($myVar);
var_dump(999);
$arr = [1, 2, "A"];
var_dump($arr);
The code above will produce the following output:
string(5) "Hello"
int(999)
array(3) {
[0]=>
int(1)
[1]=>
int(2)
[2]=>
string(1) "A"
}
When you pass a string
value, the var_dump()
function also returns the length of the string as you see in the example above.
When you need the variable type returned as a string, you should use the gettype()
function.
If you are debugging an issue during development, you may want to use the var_dump()
function as it gives you more information about a specific variable.
Now you’ve learned how to get the variable type in PHP. Nice work!