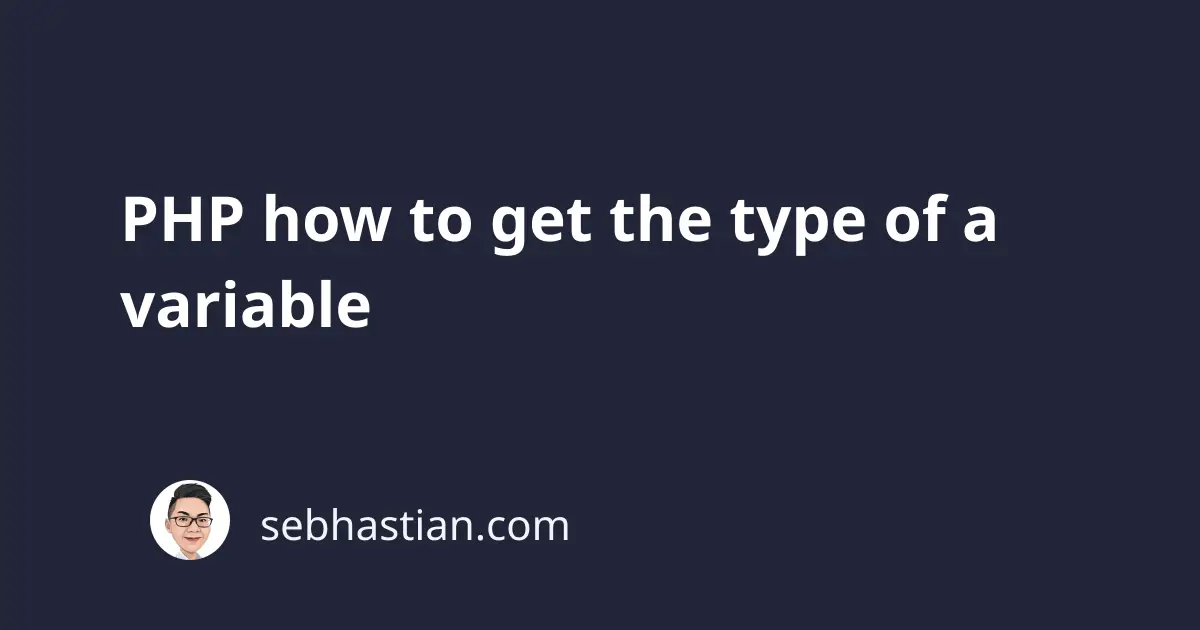
To get the type of a variable in PHP, you need to call the gettype()
function.
The syntax of the gettype()
function is as follows:
gettype(mixed $value): string
You need to pass the variable you want to check as the function’s parameter.
This function then returns a string
that represents the type of the variable.
Here’s an example of running the function:
$str = "Hello World!";
echo gettype($str); // string
$bool = true;
echo gettype($bool); // boolean
$arr = array(1, 2, 3);
echo gettype($arr); // array
// 👇 you can also pass a value directly
echo gettype(72); // integer
echo gettype(5.789); // double
Possible values for the returned string are as follows:
- string
- boolean
- integer
- double
- array
- object
- resource
- resource (closed) available since PHP 7.2
- NULL
- unknown type
There is no float type because it will be returned as a double by the function.
And that’s how you get the type of a variable in PHP.
The gettype()
function reference can be found at:
https://www.php.net/manual/en/function.gettype.php
Thanks for reading! 🙏