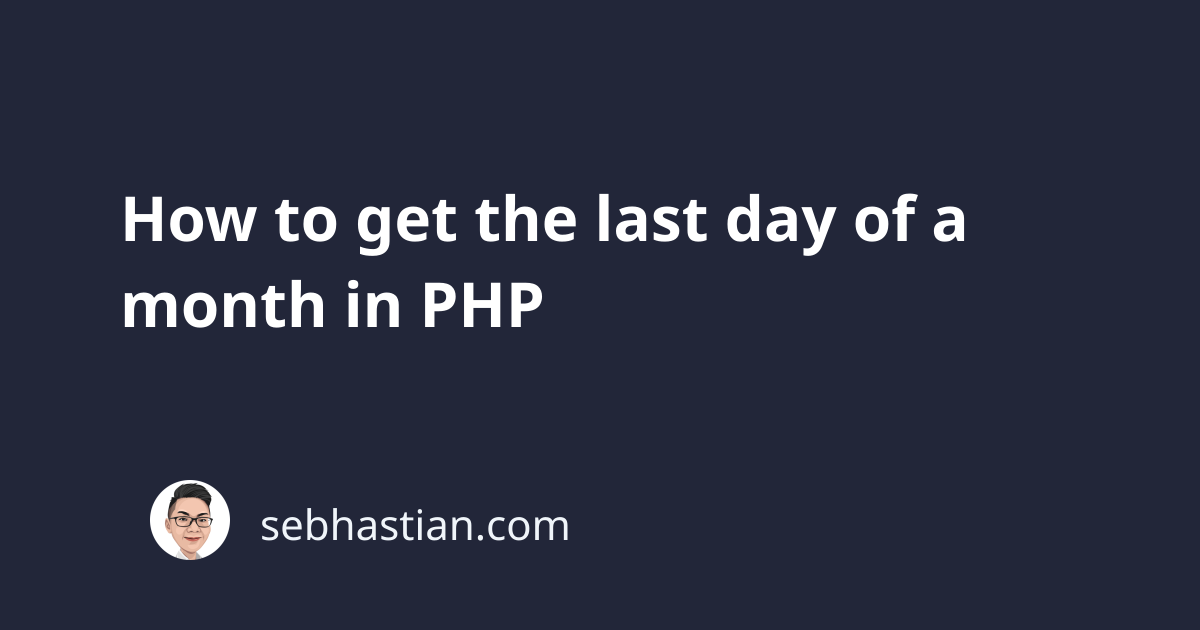
To get the last day of a month in PHP, you need to call the strtotime()
and date()
functions.
You also need to call the date()
function twice:
- First, you find the last date of the month with
date('Y-m-t')
- Then, you use the last date to get the day with
date('l')
This tutorial will show you an example of how to do the steps above.
Get the last day of a month with strtotime()
Before you get the last day of a month, you need to have a date time value that will be passed to the date()
function.
You can use the strtotime()
function to get a Unix timestamp value of a date.
For example, suppose you need to find the last day of “2nd January 2022”:
<?php
// 👇 get the timestamp of the date
$date = strtotime("2nd January 2022");
// 👇 get the last date for that month
$last_date = date("Y-m-t", $date);
print $last_date; // 2022-01-31
// 👇 convert last date to timestamp again
$last_date = strtotime($last_date);
// 👇 get the day of the last date
$last_day = date("l", $last_date);
print $last_day; // Monday
?>
If you only need the last date, then you can stop after you get the $last_date
value.
To get the last day, you need to convert the last date back to Unix timestamp using strtotime()
, then convert it back again.
Get the last day of a month with DateTime class
Alternatively, you can also use the DateTime
class to get the last date or last day of a month.
Consider the example below:
<?php
// 👇 create DateTime object from current time
$date = new DateTime();
// 👇 modify the date
$date->modify("last day of this month");
// 👇 format and print the date
print $date->format("Y-m-d l"); // 2022-09-30 Friday
?>
As you can see, using the DateTime
class to create a new DateTime
object allows you to write less code when compared with using strtotime()
.
You can move the date to the last day of the month and print it using the Y-m-d l
format to get the date and day.
This also works when you have a defined date (not using the current time):
<?php
// 👇 create DateTime object from specific time
$date = new DateTime("3rd March 2021");
// 👇 modify the date
$date->modify("last day of this month");
// 👇 print
print $date->format("Y-m-d") . "\n"; // 2021-03-31
print $date->format("l") . "\n"; // Wednesday
?>
Depending on your requirements, you can easily get the last day or the last day using the DateTime
object.
strtotime()
is an old function available since PHP v4. The DateTime
object is a newer addition in PHP v5.2 that helps you manipulate date and time values.
Now you’ve learned how to get the last day of a month in PHP. Good work! 👍