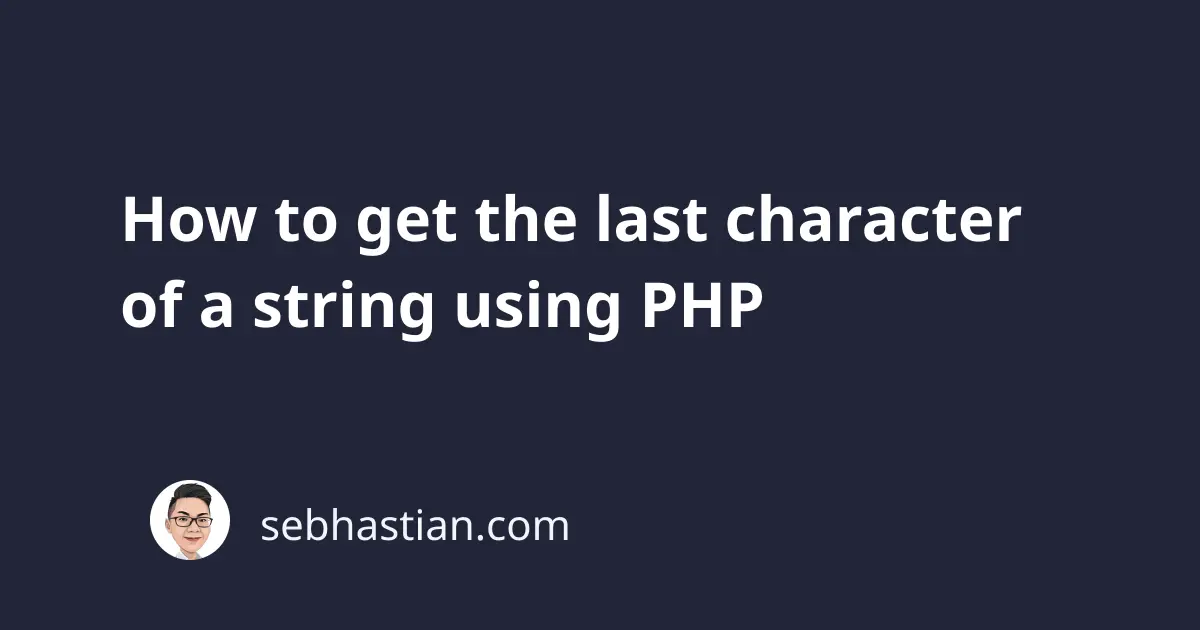
There are two ways you can get the last character of a string with PHP"
- Access the string directly using the string numeric index
- Use the
substr()
function to subtract the last character
Let’s learn how to do both in this tutorial.
Get the last character of a string with the numeric index access
Characters in a string can be accessed using a zero-based index like an array:
<?php
$str = "It's a wonderful day";
// 👇 Get the last character of the string
print $str[-1]; // Output: y
But keep in mind that the negative number index for strings only works in PHP version 7.1 and up.
This solution also won’t work if you have a multibyte string.
Use substr() to get the last character of a string
If you need to support multibyte strings and older versions of PHP, then it’s better to use the mb_substr()
function to get the last character of a string:
<?php
// get the last character of a string
print substr("It's a wonderful day", -1); // Output: y
// another example with multibyte string
print mb_substr("おはよう", -1); // Output: う
The substr()
and mb_substr()
functions will work with all kinds of strings.
They are also available in older PHP versions (>= v4.0.6).
Now you’ve learned how to get the last character of a string in PHP. Great!