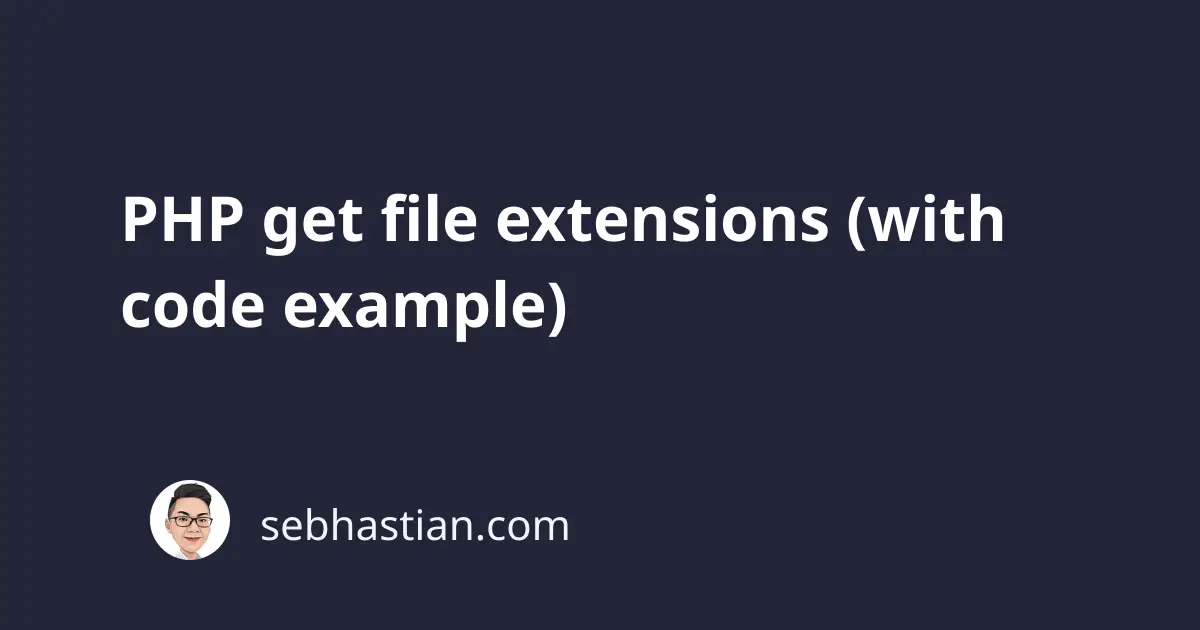
You need to call the pathinfo()
function to get the file extension in PHP.
Here’s an example:
$file = "Picture.png";
$ext = pathinfo($file, PATHINFO_EXTENSION);
echo "The file extension is $ext";
The output will be:
The file extension is png
Explanation
The pathinfo()
is a built-in PHP function used to get information from a file path.
pathinfo(
string $file,
int $flags = PATHINFO_ALL
): array|string
The function returns either an array
or a string
depending on the parameter you passed into it.
The two parameters are:
- The
$file
string that you want to parse - The
$flags
to specify what you want to get from the$file
There are four valid $flags
values:
PATHINFO_DIRNAME
PATHINFO_BASENAME
PATHINFO_EXTENSION
PATHINFO_FILENAME
If you don’t pass a $flags
value, then PHP will get all four information as an array of strings.
This is why to get a file extension, you pass the string
of the file path and the PATHINFO_EXTENSION
to the function:
$ext = pathinfo($file, PATHINFO_EXTENSION);
When you upload a file using an HTML <input>
tag, you need to get the file name
parameter from the $_FILES
array.
Here’s an example code to get the extension from an HTML form:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$file = $_FILES["the_file"];
$filename = $file["name"];
$ext = pathinfo($filename, PATHINFO_EXTENSION);
echo "<h1 style='color:red;'>The file extension is $ext</h1>";
}
?>
<h1>PHP Get file extension</h1>
<form
method="post"
action=""
enctype="multipart/form-data">
File:
<input type="file" name="the_file">
<input type="submit" name="submit" value="Submit">
</form>
When you put a file into the <input>
type and hit submit, the file extension will be printed on the page.
Here’s an example of the PHP code in action:
And now you’ve learned how to get the file extension in PHP. Nice work! 👍