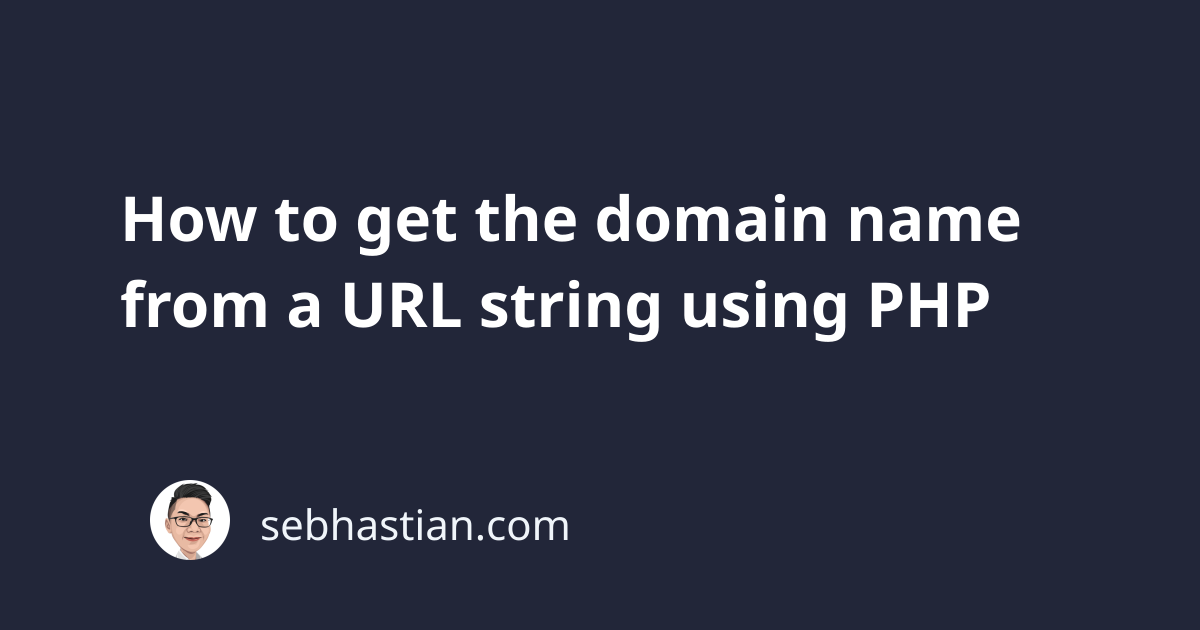
To get the domain name from a full URL string in PHP, you can use the parse_url()
function.
The parse_url()
function returns various components of the URL you passed as its argument.
The domain name will be returned under the host
key as follows:
<?php
$full_url = "http://google.com/search/console";
// π parse the url and print the host part
$array = parse_url($full_url);
print $array["host"]; // google.com
But keep in mind that the parse_url
function will return any subdomain you have in your URL.
Consider the following examples:
// π add www subdomain
$full_url = "http://www.google.co.uk/search/console";
$array = parse_url($full_url);
// π subdomain is returned
print $array["host"]; // www.google.co.uk
The same goes for any kind of subdomains your URL may have:
// π add sub subdomain
$full_url = "http://sub.reddit.com/r/gaming/comments";
$array = parse_url($full_url);
// π subdomain is returned
print $array["host"]; // sub.reddit.com
If you want to remove the subdomain from your URL, then you need to use the PHP Domain Parser library.
The PHP Domain Parser can parse a complex domain name that a regex canβt parse.
Use this library in combination with parse_url()
to get components of your domain name as shown below:
<?php
use Pdp\Domain;
use Pdp\Rules;
$full_url = "http://www.google.co.uk/search/console";
// π parse the url first
$parsed_url = parse_url($full_url);
// π parse the host to PDP
$domain = Domain::fromIDNA2008($parsed_url['host']);
// π get public suffix list from Mozilla
$publicSuffixList = Rules::fromPath('./public_suffix_list.dat');
// π resolve the domain
$result = $publicSuffixList->resolve($domain);
print $result->domain()->toString(); //display 'www.google.co.uk';
print PHP_EOL;
print $result->suffix()->toString(); //display 'co.uk';
print PHP_EOL;
print $result->secondLevelDomain()->toString();//display 'google';
print PHP_EOL;
print $result->registrableDomain()->toString();//display 'google.co.uk';
print PHP_EOL;
print $result->subDomain()->toString(); //display 'www';
print PHP_EOL;
print $result->suffix()->isIANA(); //return true
You need to have a copy of public_suffix_list.dat
that you can download from publicsuffix.org.
To get google.co.uk
from the URL, call the registrableDomain()
function from the resolved domain name.
You can see the documentation for PHP Domain Parser on its GitHub page.
Now youβve learned how to get the domain name from a URL using PHP. Nice work!