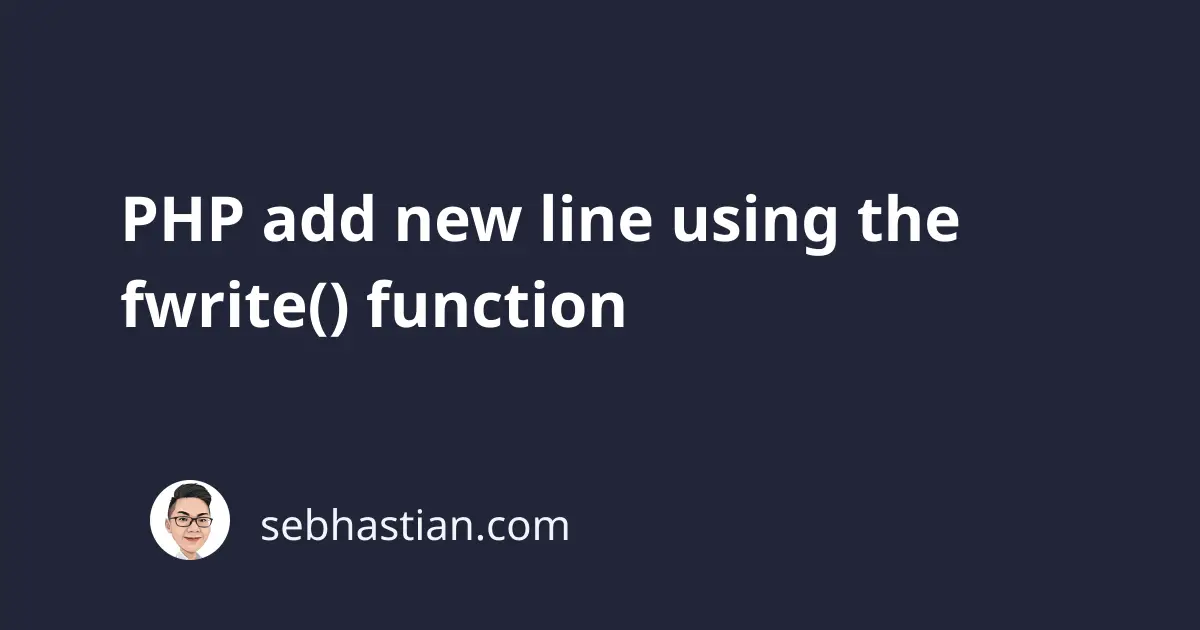
When you need to add a new line (or line break), you need to use the PHP_EOL
constant.
The reason to use PHP_EOL
instead of \n
or \r\n
is that PHP_EOL
will write the escape sequence that fits the operating system where you run PHP.
Windows OS uses \r\n
while UNIX uses \n
for its new line escape sequence.
PHP_EOL
automatically writes the escape sequence that fits the operating system.
The example below shows how to add new line at the end of the fwrite()
process:
<?php
// 👇 open file named test.txt
$file = fopen("test.txt", "w");
// 👇 add PHP_EOL as argument
fwrite($file, "Hello World!" . PHP_EOL);
// 👇 separate PHP_EOL for clarity
fwrite($file, "This text is written using PHP");
fwrite($file, PHP_EOL);
fwrite($file, "End of file");
// 👇 close file
fclose($file);
?>
The output in test.txt
will be:
Hello World!
This text is written using PHP
End of file
Using PHP_EOL
allows PHP to determine the right escape sequence to use depending on your operating system.
This constant helps you add a new line when writing to a file using the fwrite()
function.