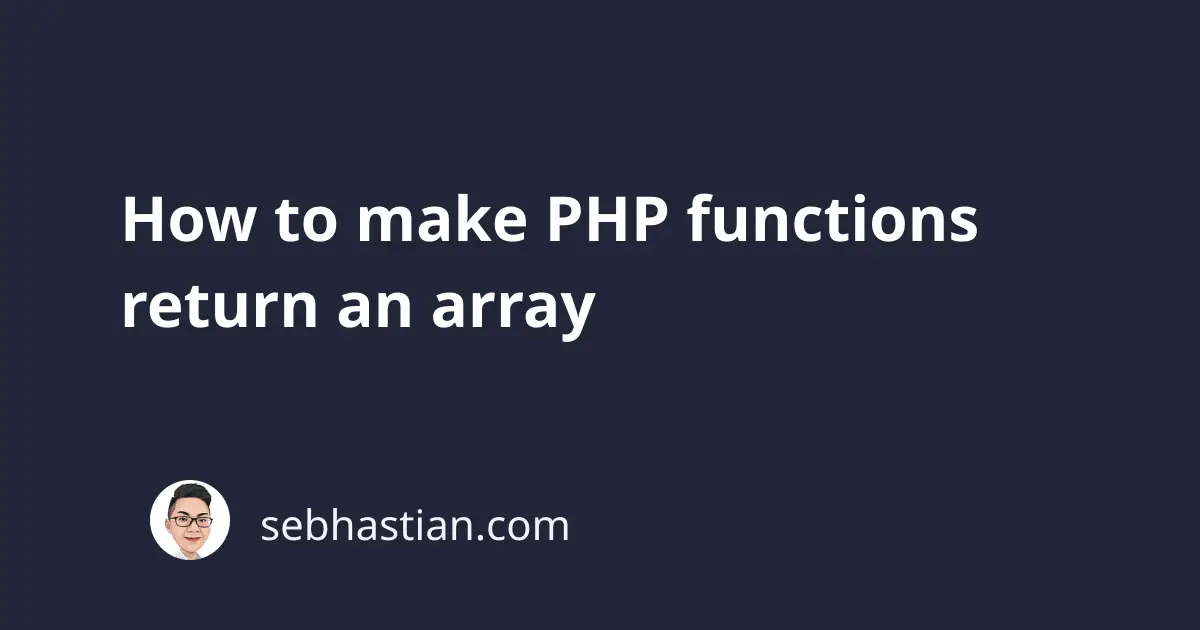
A PHP function can return only one value using the return
statement.
The return value from a PHP function can be of any type, including arrays and objects.
To return an array from a PHP function:
- you can create the array next to the
return
keyword - you can return a variable of array type
Here’s an example of making a PHP function return an array:
<?php
// 👇 1. return a literal array
function get_names() {
return ["Nathan", "Jenny"];
}
$names = get_names();
print $names[0]. "\r\n"; // Nathan
print $names[1]. "\r\n"; // Jenny
// 👇 2. return an array variable
function get_weathers() {
$weathers = ["Sunny", "Cloudy", "Rainy"];
return $weathers;
}
$var = get_weathers();
print $var[0]. "\r\n"; // Sunny
print $var[1]; // Cloudy
?>
As you can see, it’s very easy to make a PHP function returns an array.