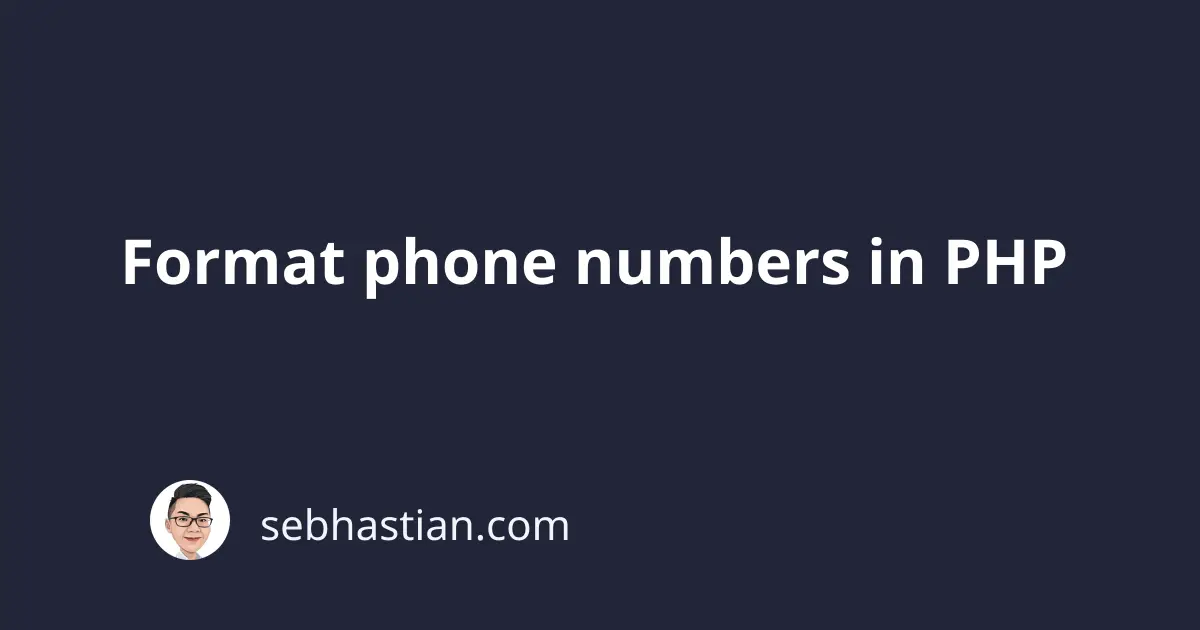
Phone numbers should not be treated as integer numbers because you don’t do math operations on them.
Some phone numbers also start with a zero, and integer type can’t save numbers with a leading zero.
Instead, phone numbers should be saved as strings that are specially formatted as phone numbers.
This tutorial will show you how to format phone numbers in PHP
Format phone numbers using substr()
Formatting phone numbers can be simple or complex depending on the requirements you need to fulfill.
One easy way to format phone numbers in PHP is by calling the substr()
function to separate your numbers like this:
<?php
$phone_no = "+1735550162";
// 👇 format phone number
$format_phone =
substr($phone_no, -10, -7) . "-" .
substr($phone_no, -7, -4) . "-" .
substr($phone_no, -4);
print $format_phone; // 173-555-0162
As you can see, the number +1735550162
is formatted as 173-555-0162
.
But keep in mind that the substr()
function can’t handle phone numbers in different formats.
For example, if you have numbers in the US national format like (111) 234 6789
then the output will be wrong.
To handle different phone numbers format, you need to use a regular expression and preg_replace()
function.
Format phone numbers using regex
Here’s an example to format US phone numbers with regex:
function format_phone(string $phone_no) {
return preg_replace(
"/.*(\d{3})[^\d]{0,7}(\d{3})[^\d]{0,7}(\d{4})/",
'($1) $2-$3',
$phone_no
);
}
print format_phone("+1-555-549-4137"); // (555) 549-4137
print PHP_EOL;
print format_phone("(555) 096 7286"); // (555) 096-7286
Please keep in mind that the above solution only works for US phone numbers.
Phone numbers from other countries may break the format created using the regular expression above.
Formatting phone numbers is hard because of the different formats and lengths.
If you want to have a complete phone number formatting solution in PHP, you need to write a lot of code for all the possible formats and lengths.
I recommend you use the libphonenumber
library instead.
Format phone numbers using libphonenumber
PHP libphonenumber is an implementation of Google’s libphonenumber, a library created for parsing, formatting, and validating phone numbers.
You can install the library using composer, then use the library in your code.
The following example shows how you can parse a phone number from Brazil:
<?php
require __DIR__ . "/vendor/autoload.php";
use libphonenumber\NumberParseException;
use libphonenumber\PhoneNumber;
use libphonenumber\PhoneNumberFormat;
use libphonenumber\PhoneNumberUtil;
$phoneUtil = PhoneNumberUtil::getInstance();
$phone_no = "+55-955-590-0169";
try {
// 👇 parse the number, pass the country code as second parameter
$parsed_number = $phoneUtil->parse($phone_no, "BR");
// 👇 get detailed output
print "Input: " . $phone_no . "\n";
print "Valid number: " .
($phoneUtil->isValidNumber($parsed_number) ? "true" : "false");
print PHP_EOL;
print "E164: " .
$phoneUtil->format($parsed_number, PhoneNumberFormat::E164);
print PHP_EOL;
print "National: " .
$phoneUtil->format($parsed_number, PhoneNumberFormat::NATIONAL);
print PHP_EOL;
print "International: " .
$phoneUtil->format($parsed_number, PhoneNumberFormat::INTERNATIONAL);
print PHP_EOL;
} catch (NumberParseException $e) {
var_dump($e);
}
The output of the code above will be:
Input: +55-955-590-0169
Valid number: true
E164: +559555900169
National: (95) 5590-0169
International: +55 95 5590-0169
Instead of writing your own library, you can use libphonenumber
to help you format phone numbers with ease.
I hope this tutorial has been useful for you. 🙏